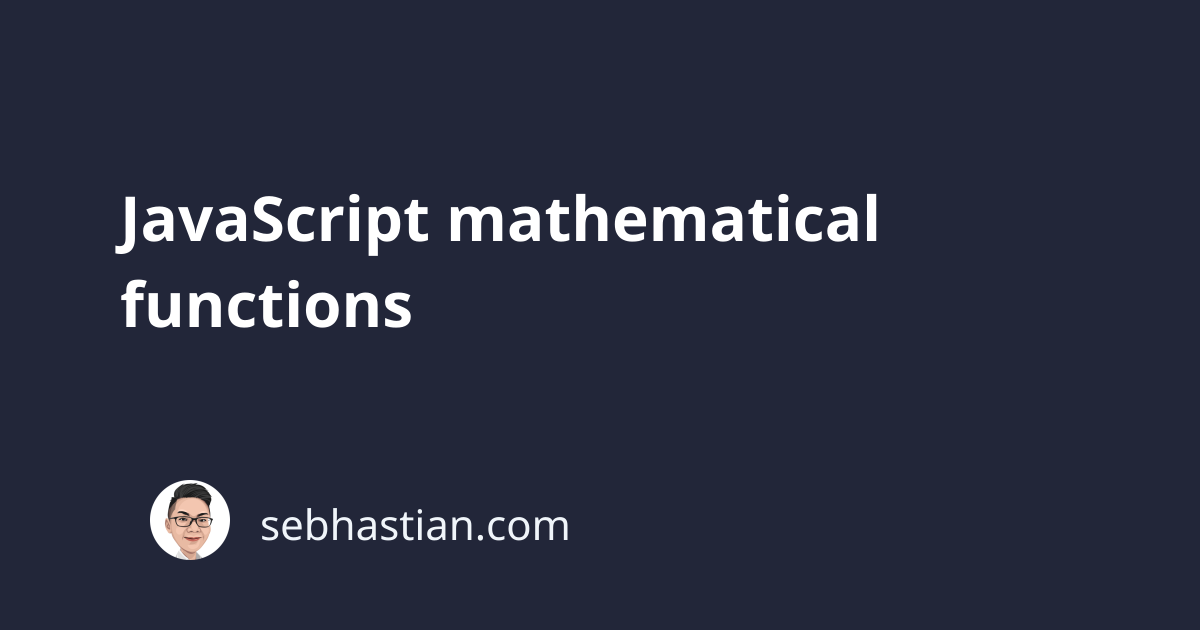
JavaScript has a built-in object called Math
that contains methods and constants to help you perform mathematical operations and manipulate numbers. All methods and constants of Math
are static, which means you don’t need to create an instance of Math
to call on them. You can call the methods right away in any part of your code.
For example, you can generate a random number between 0 and 1 using Math.random()
:
const randomNum = Math.random();
Math constant properties
Mathematics also has a number of fixed constants, such as the number of Pi (π) which is always 3.14
.
The Math
object also store these fixed mathematical constants as the object properties. There are 8 math constants currently provided by JavaScript:
Math.E;
// Euler's constant and the base of natural logarithms; approximately 2.718.
Math.LN2;
// Natural logarithm of 2; approximately 0.693.
Math.LN10;
// Natural logarithm of 10; approximately 2.303.
Math.LOG2E;
// Base-2 logarithm of E; approximately 1.443.
Math.LOG10E;
// Base-10 logarithm of E; approximately 0.434.
Math.PI;
// Ratio of the a circle's circumference to its diameter; approximately 3.14159.
Math.SQRT1_2;
// Square root of ½ (or equivalently, 1/√2); approximately 0.707.
Math.SQRT2;
// Square root of 2; approximately 1.414.
You can use these constants in your code immediately:
let diameter = 9;
let circumference = Math.PI * diameter;
// Circumference = π X Diameter
Math static methods reference
Some of the most used Math
methods are as follows:
Here’s the full list of the available methods:
Math.abs()
Returns the absolute value of the number parameter.
Math.abs(5); // 5
Math.abs(-5); // -5
Math.acos()
Returns the arccosine of the number, in radians.
The number argument must be between -1
and 1
or it will return NaN
Math.acos(0.8); // 0.6435011087932843
Math.acos(-0.8); // 2.498091544796509
Math.acosh()
Returns the hyperbolic arccosine of the number parameter.
Negative number and 0
will return NaN
Math.acosh(2); // 1.3169578969248166
Math.acosh(-2); // NaN
Math.asin()
Returns the arcsine of the number, in radians.
The number parameter must be between -1
and 1
or it will return NaN
Math.asin(0.1); // 0.1001674211615598
Math.asin(-0.1); // -0.1001674211615598
Math.asinh()
Returns the hyperbolic arcsine of the number parameter.
Math.asinh(5); // 2.3124383412727525
Math.asinh(-5); // -2.3124383412727525
Math.atan()
Returns the arctangent of the number parameter as a numeric value between -PI/2 and PI/2 radians.
Math.atan(25); // 1.5308176396716067
Math.atan(-25); // -1.5308176396716067
Math.atan2()
Returns the arctangent of the quotient of its argument. It requires two number parameters:
- The
y
coordinate as the first parameter - The
x
coordinate as the second parameter
Math.atan2(8, 4); // y=8 and x=4 returns 1.1071487177940904
Math.atanh()
Returns the hyperbolic arctangent of the parameter.
The number parameter must be between -1
and 1
or it will return NaN
If the parameter is 1
it will return Infinity
If the parameter is -1
it will return -Infinity
Math.atanh(0.5); // 0.5493061443340548
Math.cbrt()
Returns the cubic root of the parameter.
Math.cbrt(110); // 4.791419857062784
Math.ceil()
Returns the number rounded up to the closest round number (or integer)
Math.ceil(2.1); // 3
Math.ceil(-2.1); // -2
Math.clz32()
Returns the number of leading zeros in a 32-bit binary representation of the parameter.
The “clz32” is short for Count Leading Zeroes 32.
Math.clz32(1);
// 00000000000000000000000000000001
// returns 31
Math.clz32(4);
// 00000000000000000000000000000100
// returns 29
Math.cos()
Returns the cosine of the parameter. The return value will be between -1
and 1
Math.cos(8); // -0.14550003380861354
Math.cosh()
Returns the hyperbolic cosine of the parameter
Math.cosh(5); // 74.20994852478785
Math.exp()
Returns Euler’s number (Math.E
) to the power of the parameter (the parameter is the exponent number)
Math.exp(2);
// equals to Math.E * Math.E
// returns 7.3890560989306495
Math.exp(3);
// equals to Math.E * Math.E * Math.E
// returns 20.085536923187668
Math.expm1()
Returns the value of Math.exp
minus 1
Math.expm1(2); // 6.38905609893065
Math.expm1(3); // 19.085536923187668
Math.floor()
Returns the parameter, rounded down to the closest integer
Math.floor(9.9); // 9
Math.fround()
Returns the nearest (32-bit single precision) float representation of a number
Math.fround(3.33); // 3.3299999237060547
Math.fround(3.2); // 3.200000047683716
Math.log()
Returns the natural logarithm of the parameter
Math.log(2); // 0.6931471805599453
Math.log(10); // 2.302585092994046
Math.log10()
Returns the base-10 logarithm of the parameter
Math.log10(9); // 0.9542425094393249
Math.log10(20); // 1.3010299956639813
Math.log1p()
Returns the natural logarithm of 1 + x
Math.log1p(9); // 2.302585092994046
Math.log1p(20); // 3.044522437723423
Math.log2()
Returns the base-2 logarithm of the parameter
Math.log2(9); // 3.169925001442312
Math.log2(20); // 4.321928094887363
Math.max()
Returns the number with the highest value from one or more numbers. Can accept as many numbers as needed.
Math.max(9); // 9
Math.max(9, 20, 30, 39, 40, 50, 59); // 59
Math.min()
Returns the number with the lowest value from one or more numbers
Math.min(9); // 9
Math.min(9, -20, 30, 39, -40, 50, 59); // -40
Math.pow()
Calculates the exponent value of a number. It requires two parameters:
- The first parameter is the
base
number - The second parameter is the
exponent
number to raise thebase
Math.pow(5, 3); // 125
Math.random()
Returns a random number between 0 and 1. It doesn’t have any parameter
Math.random();
// 0.7641735276363635 but the value will always change
Math.round()
Rounds the parameter to the nearest integer. Will go up when the decimal value is .5
or higher
Math.round(6.4); // 6
Math.round(6.5); // 7
Math.sign()
Returns the sign of a number (checks whether the argument is positive, negative or zero)
- If the argument is positive, returns
1
. - If the argument is negative, returns
-1
. - If the argument is positive zero, returns
0
. - If the argument is negative zero, returns
-0
. - Otherwise,
NaN
is returned.
Math.sign(7); // 1
Math.sign(-7); // -1
Math.sign(0); // 0
Math.sin()
Returns the sine of the argument
Math.sin(9); // 0.4121184852417566
Math.sinh()
Returns the hyperbolic sine of the given number
Math.sinh(5); // 74.20321057778875
Math.sqrt()
Returns the square root of the argument you passed into it
Math.sqrt(9); // 3
Math.tan()
Returns the tangent of the given number
Math.tan(8); // -6.799711455220379
Math.tanh()
Returns the hyperbolic tangent of the argument
Math.tanh(1); // 0.7615941559557649
Math.trunc()
Returns the integer part of the argument. Works for numbers with decimal values.
Math.trunc(8.76); // 8
Math.trunc(9.7612938); // 9