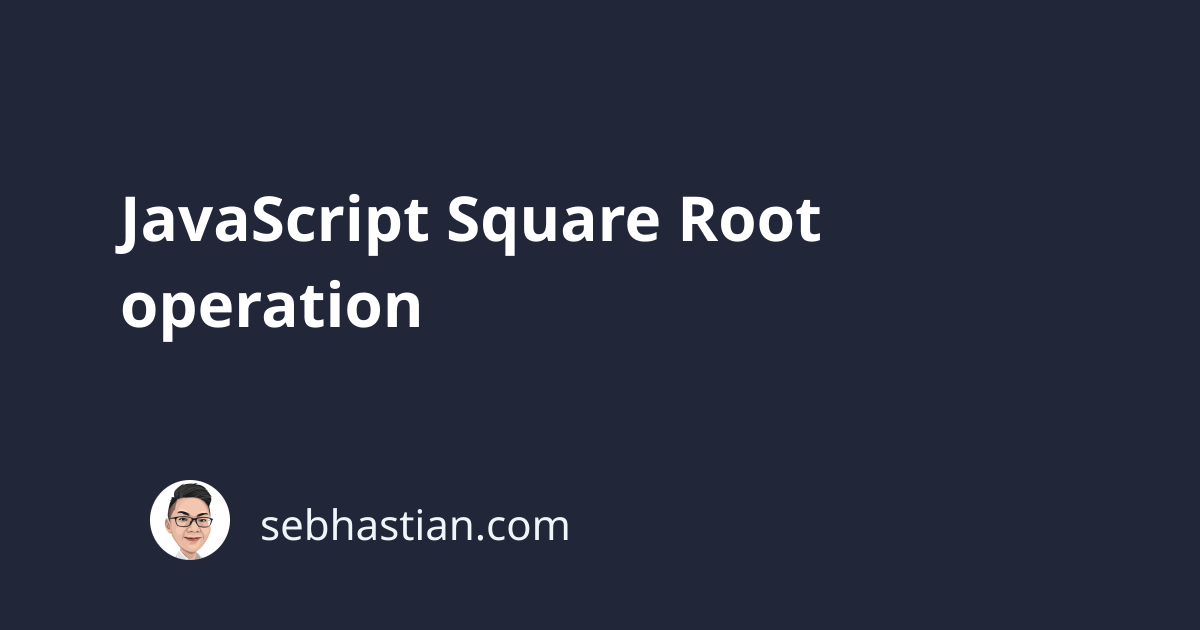
To find the Square Root value of a certain number, you can use the JavaScript Math.sqrt()
method. You need to pass the number into the method as follows:
Math.sqrt(9);
// returns 3
If you pass a negative number to the method, it will return NaN
instead of a number:
Math.sqrt(-9);
// returns NaN
The method only accepts one parameter. When you pass more than one parameter, the rest is ignored:
Math.sqrt(9, 18, 27);
// returns 3
Square Root constants in JavaScript
The Math object also has two static square root properties that you can use in your code immediately. They are:
Math.SQRT1_2
which returns the Square Root value of 1/2Math.SQRT2
which returns the Square Root value of 2
Since both are static properties, you can use them just like a normal variable:
console.log(`The Square Root of 1/2 is ${Math.SQRT1_2}`);
console.log(`The Square Root of 2 is ${Math.SQRT2}`);