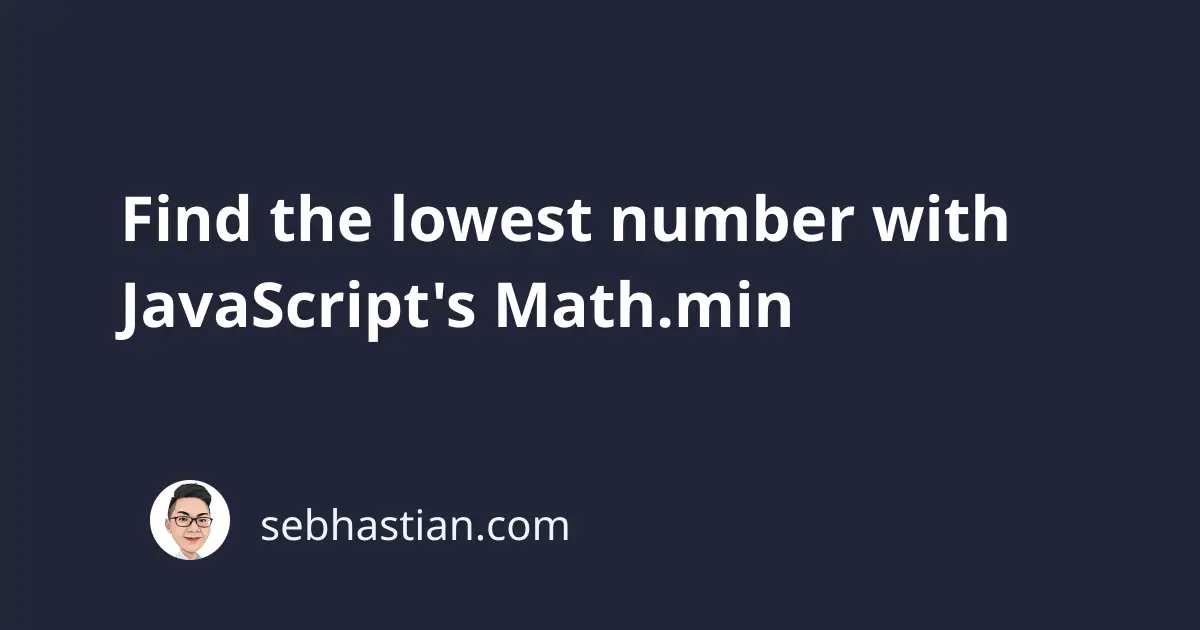
You can use JavaScript’s Math.min()
method in order to find the number with the lowest value from several numbers. The method accepts as many number arguments as you want to pass into it:
Math.min(20, 3, 10, 29);
// returns 3
You can even pass only one number, though the method will just return that number:
Math.min(2);
// returns 2
You can also pass a negative number into the method:
Math.min(102, 8, -20, -39);
// returns -39
When you pass a non-number value, the method will return NaN
as value:
Math.min(102, "string", -20, -39, "lion");
// returns NaN
Finding the minimum value in an array
The Math.min()
method only accepts numbers. If you need to find the lowest number inside an array, you need to use the JavaScript spread operator (...
) to pull out the numbers from the array (also known as expanding the object):
let scores = [18, 7, 2, 44];
Math.min(...scores);
// also the same as Math.min(18, 7, 2, 44);
// returns 2
Math.min(...[2, 4, -9]);
// returns -9