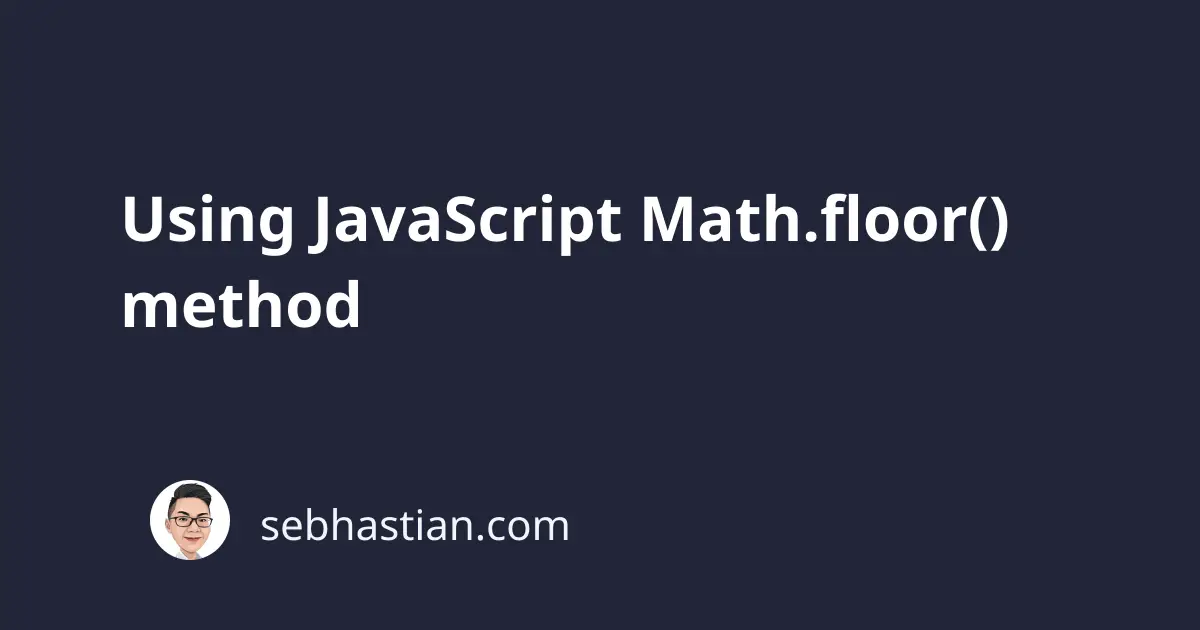
The JavaScript Math.floor()
method is a part of the Math object that you can use to round a decimal number down. For example, a number variable of 7.2
will be rounded down to 7
when you pass it into the method.
The syntax for this method looks as follows:
Math.floor(7.2); // returns 7
You can pass the initial number as a variable:
let score = 7.2;
Math.floor(score); // returns 7
Or store the returned number in a variable like this:
let score = 7.2;
let roundedScoreDown = Math.floor(score);
console.log(roundedScoreDown); // 7
The method accepts only one parameter of number
value. If you pass a string
, an object
, or an array
to the method, it will return NaN
which stands for Not a Number
:
Math.floor("Hello there!");
Math.floor([1, 2, 3]);
Math.floor({ name: "Andy" });
// returns NaN
When you pass a boolean
value of true
or false
it will return 1
for true
and 0
for false
:
Math.floor(true);
// returns 1
Math.floor(false);
// returns 0
Remember to use dot (.
) when you pass in a decimal number because a comma (,
) in JavaScript parameters is an indicator of separate variables. In the following example, JavaScript will simply floor the first number and ignore the second when you pass two numbers:
Math.floor(9, 2);
// returns 9
Finally, the Math.floor() method will round a number down no matter if the decimal value is .5
or higher:
Math.floor(8.5);
// returns 8
Using Math.floor() on negative numbers
The method will also round a negative number down. Negative numbers get smaller the farther they are from zero, so the number value of -55.2
will be rounded to -56
and not -55
:
Math.floor(-55.2)
// returns -56
Other Math rounding method
Finally, if you want to round the number up when its decimal value is higher than .5
then you need to use Math.round()
method.
When you want to round the number up instead of down (from 7.2
to 8
) then you need to use [the Math.ceil()
method]( {{ <ref “/18-javascript-math-ceil”> }} ).