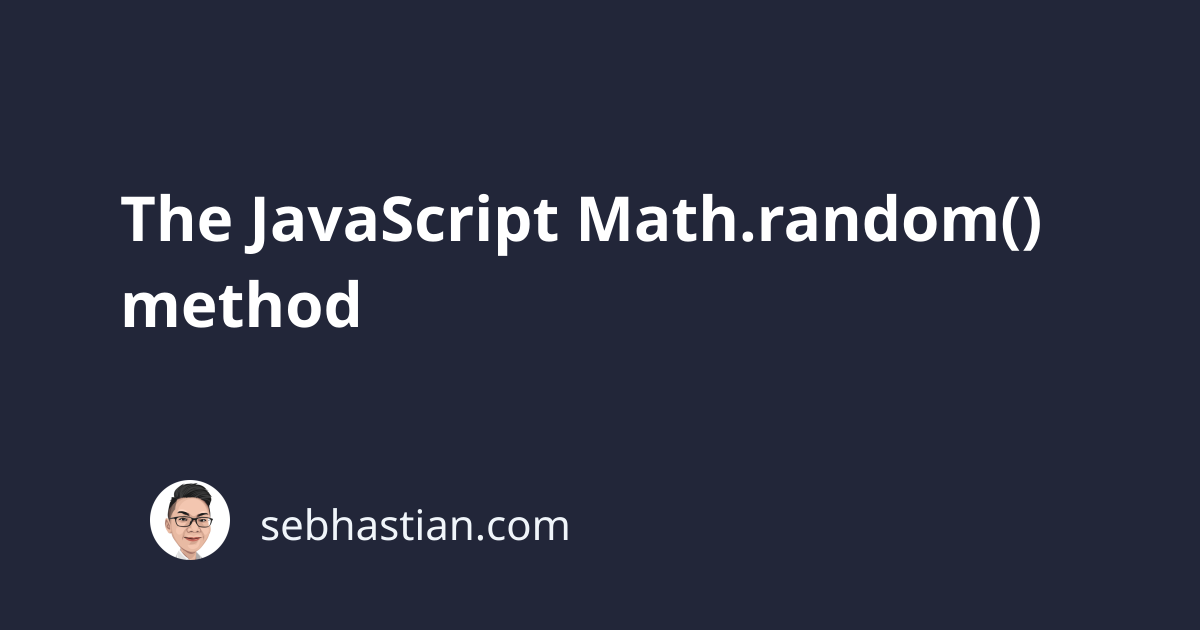
The Math.random()
method is one of JavaScript’s Math object method that returns a positive random number that’s lower than 1, meaning it can generate a random number between 0
and 0.99999999999999999
console.log(Math.random());
// 0.35574907184159277
console.log(Math.random());
// 0.7612336564594773
console.log(Math.random());
// 0.669688248313085
console.log(Math.random());
// 0.8246898327750964
This method doesn’t receive any parameters.
Generating integer numbers within a range
This number returned by this method can be used as the spark to generate a random number between a specified range of your choosing. For example, let’s say you want to generate a random number between 50
to 60
. Here’s how you do it:
const randomNum = Math.random();
const randomBetween = randomNum * (6 - 50) + 50;
The math formula is as follows:
randomNum * (max - min) + min
However, since the random number generator can only generate numbers lower than 1, this means you will only receive numbers between 50
and 59.99...
to include the maximum number into the random generator, you need to add a + 1
into the formula:
randomNum * (max - min + 1) + min
But we’re not done yet here! The formula above will return a number between 50
and 60.99...
0.999 * (60 - 50 + 1) + 50 = 60.98900
The random number will never return 61
, so all you need to do is to round the number down with the Math.floor()
method:
Math.floor(0.999 * (60 - 50 + 1) + 50);
// returns 60
The complete formula is as follows:
const randomNum = Math.random();
const min = 50;
const max = 60;
const randomBetween = randomNum * (max - min + 1) + min;
const randomInteger = Math.floor(randomBetween);
Now you can generate a random number between 50
to 60
, although you can’t generate a decimal number this way.
Generating decimal numbers within a range
If you want your random generator to also allows decimal numbers, you need to replace the generated number with max
only when the randomBetween
value is higher than max
:
const randomNum = Math.random();
const min = 50;
const max = 60;
let randomBetween = randomNum * (max - min + 1) + min;
if (randomBetween > max) {
randomBetween = max;
}
Now you can have decimal numbers between the specified range.