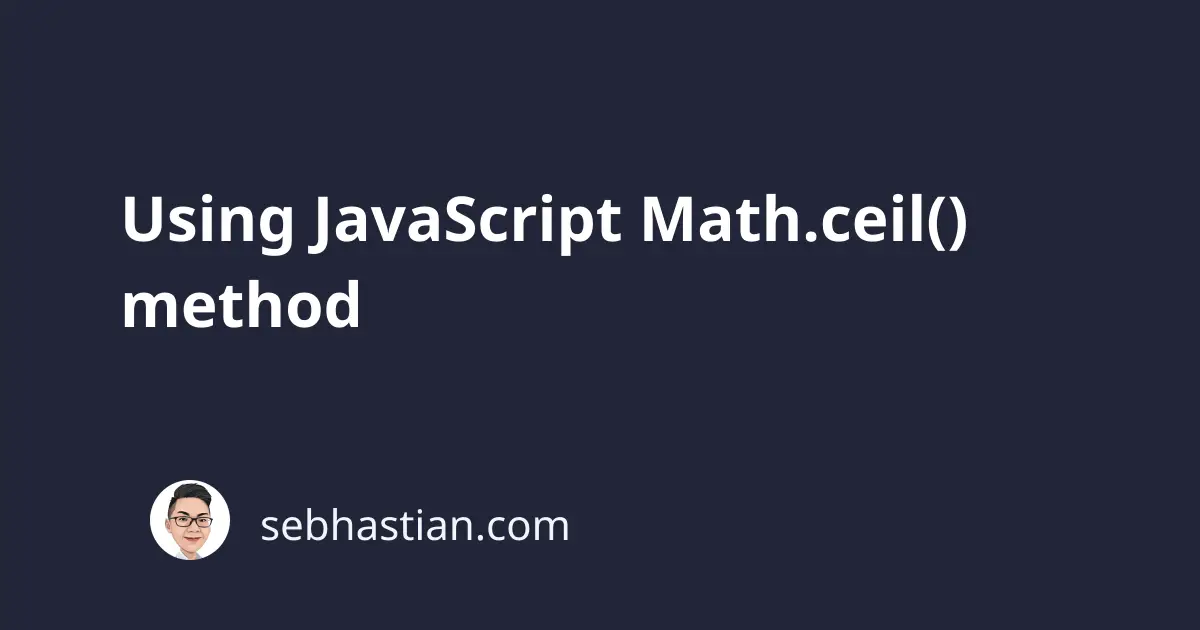
The JavaScript Math.ceil()
method is a method from Math object that’s used to round a decimal number up to the closest round number. For example, a number variable of 7.2
will be rounded up to 8
when you pass it into the method.
The syntax for this method looks as follows:
Math.ceil(7.2); // returns 8
You can pass the initial number as a variable:
let score = 7.2;
Math.ceil(score); // returns 8
The returned number can also be stored in a variable like this:
let score = 7.2;
let roundedScoreUp = Math.ceil(score);
console.log(roundedScoreUp); // 8
The method accepts only one parameter of number
value. If you pass a string
, an object
, or an array
to the method, it will return NaN
which stands for Not a Number
:
Math.ceil("Hello there!");
Math.ceil([1, 2, 3]);
Math.ceil({ name: "Andy" });
// returns NaN
When you pass a boolean
value of true
or false
it will return 1
for true
and 0
for false
:
Math.ceil(true);
// returns 1
Math.ceil(false);
// returns 0
When you pass more than one parameter, JavaScript will simply ignore the next parameters and operate on the first one:
Math.ceil(5.5, 2, 80);
// returns 6
Using Math.ceil() on negative numbers
The method will also round a negative number up. Negative numbers get smaller the farther they are from zero, so the number value of -55.7
will be rounded to -55
and not -56
:
Math.ceil(-55.7);
// returns -55
Other Math rounding method
If you want to round the number up when its decimal value is .5
or higher and round the number down when its decimal value is lower than .5
then you need to use Math.round()
method.
When you want to round the number down instead of up (from 7.8
to 7
) then you need to use the Math.floor()
method.