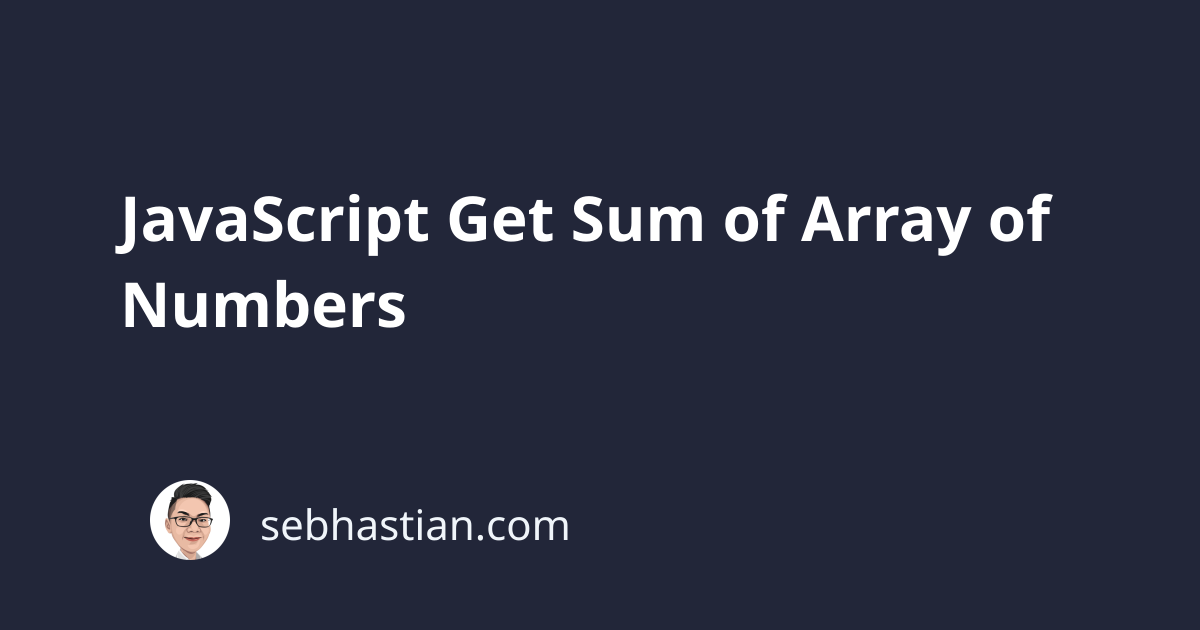
Hello friends! Today I will be showing you how to get the sum of an array of numbers in JavaScript.
A JavaScript array can store a collection of multiple values under one variable name. When you have an array of numbers, you can sum those numbers by using either the for
loop or the reduce
method.
Let me show you how to use these two solutions to sum an array of numbers.
1. Sum an array of numbers using a for loop
The for
loop can be used to sum an array of numbers. You need to iterate over each item in the array by using the array’s length
value as the condition of the loop.
You also need to declare a variable that will hold the sum of the array. Let’s call that variable sum
as follows:
let myNumbers = [1, 3, 5, 8];
// declare a variable that will hold the sum
let sum = 0;
// iterate over each item in the array
for (let i = 0; i < myNumbers.length; i++) {
sum += myNumbers[i];
}
console.log(sum); // 17
In the example above, I created an array named myNumbers
which contain the numbers I want to sum.
Next, I declared the sum
variable, initialized as 0
. This variable will store the result of the calculations later.
After that, I created a for
loop to iterate over the elements of the myNumbers
array. I used the array length as the condition of the loop; as long as the value of i
is less than the array’s length, run the loop.
Inside the loop, the value of the sum
variable is added by the value of the array’s element using the addition assignment operator.
Once the loop is finished, the sum
variable would have the total sum of the myNumbers
array, as shown above.
You can learn more about the for
loop here
Next, let’s see how to use the reduce()
method to sum an array of numbers.
2. Sum an array of numbers using the reduce() method
The reduce()
method is used to iterate over an array of elements, accumulating each value up until the last element in the array.
The method returns a single value as the result of the accumulation process. You can use this method to sum an array of numbers as shown below:
let myNumbers = [1, 3, 5, 8];
// declare a variable that will hold the sum
let sum = myNumbers.reduce(
(accumulator, currentValue) => accumulator + currentValue
);
console.log(sum); // 17
In the above example, the reduce()
method is called on the myNumbers
array. The reduce()
method accepts a callback function which gets called on each element of the array.
This callback function require two parameters:
accumulator
is the variable that stores the value returned from the previous callcurrentValue
is the variable that represents the current array element
When the reduce()
method finished running, the accumulator
value will be returned as the result of the method. In this example, the value is assigned to the sum
variable.
And that’s how you get the sum of array of numbers using the reduce()
method. You can learn more about the method here
Conclusion
This article has shown you how to sum an array of numbers using a for
loop and the reduce()
method. I have more articles related to JavaScript array here:
- Understanding JavaScript 2D array
- JavaScript Filter Array Elements With Multiple Criteria or Conditions
- also, How To Find the Largest Number in a JavaScript Array
I know these tutorials will be useful in your coding journey. Until next time! 👋