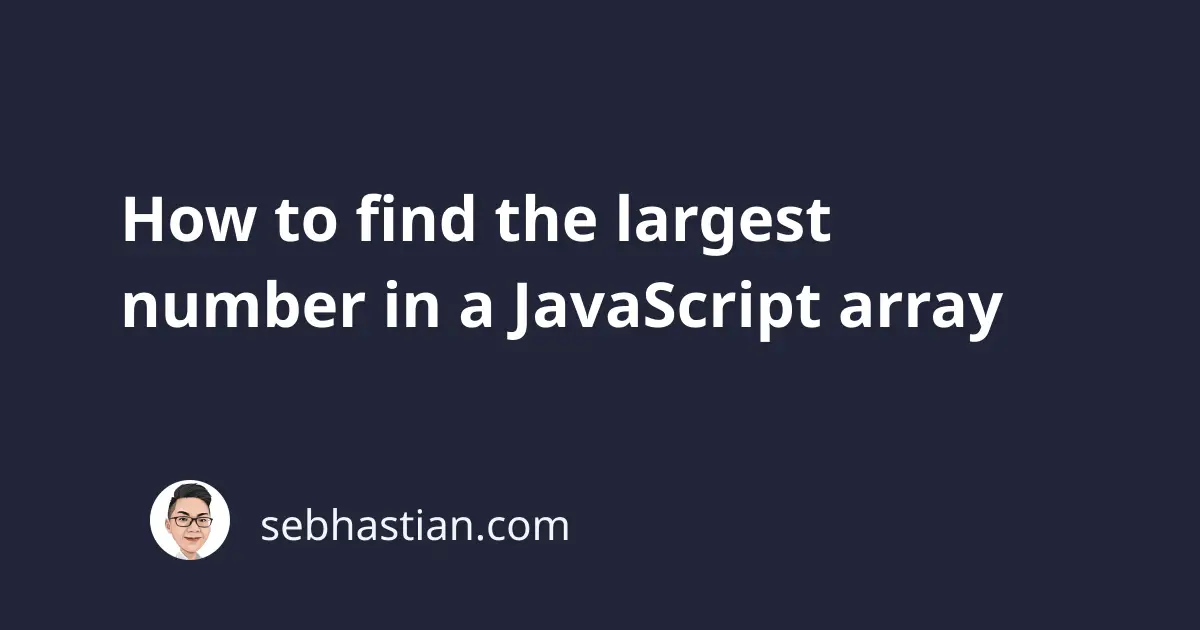
There are two ways you can find the largest number from a JavaScript array:
- Using the
forEach()
array method - Using the
reduce()
array method
This tutorial will help you learn how to do both. Let’s start with using the forEach()
method first.
Finding the largest number in an array using forEach()
The Array forEach()
method allows you to loop over an array in order from the first index to the last index.
It accepts a callback
function from which it passes the value contained inside the array for you to process.
Array.forEach(function callbackFn(element, index, array) {
// do something for each element
}, thisArg);
Here’s an example of a forEach()
method in action:
let arr = [4, 5, 6, 7, 8, 9, 10];
arr.forEach((element) => {
console.log(element);
});
The code above should show the following logs:
4
5
6
7
8
9
10
Knowing how the forEach()
method works, Here’s how you can find the largest number in an array:
- Initialize a variable with the value
0
calledtemp
- Compare and see if the value of
temp
is smaller than the current element inside the loop - If
temp
is smaller, then assign the current element value intotemp
Here’s a working example of the steps above:
let arr = [4, 5, 6, 7, 8, 9, 10];
temp = 0;
arr.forEach((element) => {
if (temp < element) {
temp = element;
}
});
console.log(`The largest number in the array: ${temp}`);
The resulting log will be as follows:
The largest number in the array: 10
Let’s test the method by creating a randomized array of numbers:
let arr = [5, 2, 67, 37, 85, 19, 10];
temp = 0;
arr.forEach((element) => {
if (temp < element) {
temp = element;
}
});
console.log(`The largest number in the array: ${temp}`);
The result of the code above will be 85
And that’s how you use the forEach()
method to find the largest number in an array.
Let’s see how you can use the reduce()
method to achieve the same goal next.
Finding the largest number in an array using reduce()
The reduce()
method allows you to execute a reducer function for each element in your array.
A reducer function takes the current accumulated value and the current value to produce a new value.
For example, suppose you have the following array:
let arr = [5, 2, 67, 37, 85, 19, 10];
You can call the reduce method on the array as shown below:
arr.reduce(function (accumulatedValue, currentValue) {
// reducer function content
});
The value you return
from the function will be the accumulatedValue
used for the next iteration of the reduce()
method.
For the first iteration, the accumulatedValue
will be assigned with the value at index zero. When the iteration is finished, the reduce()
method will return a single value, which is the accumulatedValue
.
Knowing this, you can call the Math.max()
method inside the reducer function to compare the numbers between the accumulatedValue
and the currentValue
.
The returned value will be the largest number from the array as shown below:
let arr = [5, 2, 67, 37, 85, 19, 10];
let largestNum = arr.reduce(function (accumulatedValue, currentValue) {
return Math.max(accumulatedValue, currentValue);
});
console.log(`The largest number: ${largestNum}`);
// The largest number: 85
And that’s how you find the largest number in an array using the reduce()
method. You can learn more about the use of reduce()
method here: