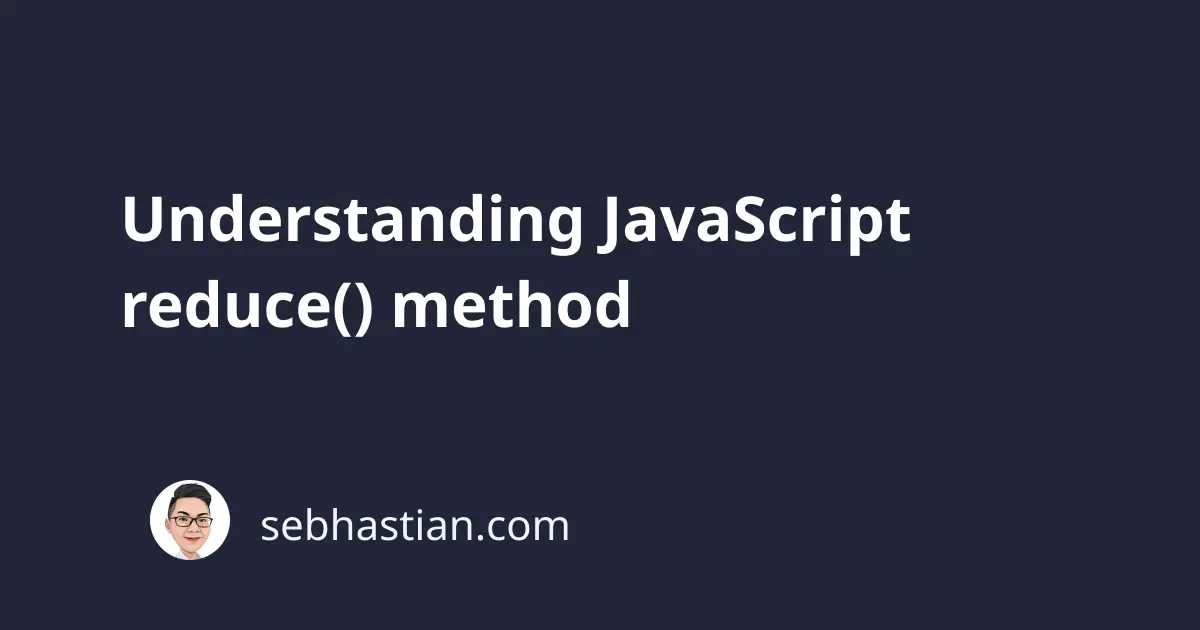
The JavaScript reduce()
is a method of Array class that you can call on any arrays that you have in your JavaScript code. The method will execute a reducer function that you need to provide as the first argument.
But what’s a reducer function? It is simply a function that gradually accumulates your array values, combining them into a single value.
Understanding reduce()
in theory is hard, so let me show you a very basic example of using the method.
Reduce use case: sum of array values
You need to find the sum of all the values in a single array. Here’s the array:
let scores = [3, 4, 8];
You need to find the sum of these three numbers, which is 16
. Here’s how you do it with the reduce
method:
let scores = [3, 4, 8];
let sum = scores.reduce(function (accumulator, currentValue) {
console.log(`accumulator is ${accumulator}
and currentValue is ${currentValue}`);
return accumulator + currentValue;
});
When you run the code above on the browser or Node, you will have the following console logs:
accumulator is 3
and currentValue is 4
accumulator is 7
and currentValue is 8
First, the accumulator is 3
because that’s the value at index zero in your array. You can also provide the initial value for the accumulator
by passing it as an argument after the callback/ reducer function.
Notice the initialValue
variable being passed after the callback function below:
let scores = [3, 4, 8];
let initialValue = 0;
let sum = scores.reduce(function (accumulator, currentValue) {
console.log(`accumulator is ${accumulator}
and currentValue is ${currentValue}`);
return accumulator + currentValue;
}, initialValue);
Your console will now log the following records:
accumulator is 0
and currentValue is 3
accumulator is 3
and currentValue is 4
accumulator is 7
and currentValue is 8
Summing it all up, the reduce
method simply iterates through your array in order to execute the reducer function that you passed as a callback into it.
The signature of reduce
method is as follows. I will explain it afterward:
array.reduce(reducerFunction, initialValue);
function reducerFunction(accumulator, currentValue, index, array) {
// what you do here is up to you
// but you must have a return statement
}
The reduce
method will pass four arguments into your reducer function:
accumulator
- Thereturn
value of the last execution. It can be the initial value or the value at index0
depending on the code you write.currentValue
- The value of the current iteration. Starts from index0
to the last index.index
- Optional. The index of the current iteration. You may need this to perform some taskarray
- Optional. The original array where you callreduce()
The last two parameters are optional, so you may include them only when you need to. The reducer function will be called as many times as the length of your array, with the accumulator
value updated each time.
Finding maximum or minimum number with reduce
You can use the reduce
method to find the highest or the lowest number value in an array:
let scores = [7, 6, 5];
let maxValue = scores.reduce(function (a, b) {
return Math.max(a, b);
});
let minValue = scores.reduce(function (a, b) {
return Math.min(a, b);
});
Removing duplicate values with reduce
You can also remove duplicate values of an array with reduce()
. But since you want to return an array instead of a number, you need to define the initial value as an empty array. Take a look at the following code example:
let myScores = [1, 2, 7, 2, 5, 8, 7];
let initialValue = [];
let noDupeArray = myScores.reduce(function (accumulator, currentValue) {
if (!accumulator.includes(currentValue)) {
accumulator.push(currentValue);
}
return accumulator;
}, initialValue);
The accumulator
starts as an empty array. If the current value is not yet included in the accumulator
, you push
the value into it.
The reduce
method can return any data type that you want to return, as long as it’s a single value (a string, a number, an array or an object, or even boolean).