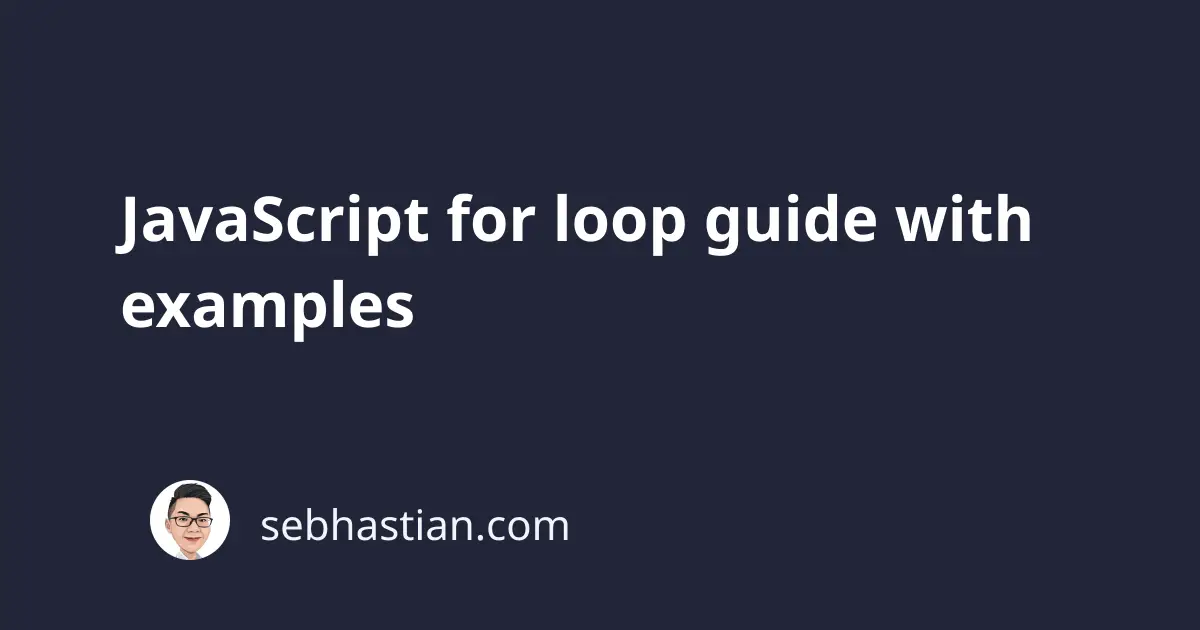
As you program an application in JavaScript, you will certainly find problems that needs you to write a piece of code that needs to be executed repeatedly.
Loop statement is a code statement that executes again and again until a certain, defined condition is achieved.
Let’s say you want to write a program that “prints number 1 to 10 into the console”. You can do it by using console.log
10 times:
console.log(1);
console.log(2);
console.log(3);
console.log(4);
console.log(5);
// and so on..
It works, but there is a better way to write this kind of repetitive code. With the loop statement for
, you can write it like this:
for (let x = 0; x < 10; x++) {
console.log(x);
}
There you go! The for
statement is followed by a parentheses (()
) which contains 3 expressions:
initialization
expression, where you declare a variable to be used as the source of the loop condition. Represented asx = 1
in the example.condition
expression, where the variable in initialization will be evaluated for a specific condition. Represented asx < 11
in the examplearithmetic
expression, where the variable value is either incremented or decremented by the end of each loop
These expressions are separated by a semicolon (;
)
After the expressions, the curly brackets ({}
) will be used to create a code block that will be executed by JavaScript as long as the condition
expression returns true
.
You can identify which expression is which by paying attention to the semicolon (;
) which ends the statement.
for ( [initialization]; [condition]; [arithmetic expression]) {
// As long as condition returns true,
// This block will be executed repeatedly
}
The arithmetic expression can be an increment (++
) or a decrement (--
) expression. It is run once each time the execution of the code inside the curly brackets end:
for (let x = 10; x >= 1; x--) {
console.log(x);
}
Or you can also use shorthand arithmetic operators like +=
and -=
as shown below:
// for statement with shorthand arithmetic expression
for (let x = 1; x < 20; x += 3) {
console.log(x);
}
Once the loop is over, JavaScript will continue to execute any code you write below the for
body:
for (let x = 1; x < 2; x++) {
console.log(x);
}
console.log("The for loop has ended");
console.log("Continue code execution");
When to use for loop
The for loop is useful when you know how many times you need to execute the loop.
For example, let’s say you’re writing a program to flip a coin. You need to find how many times the coin lands on heads when tossed 10 times. You can do it by using the Math.random
method:
- When the number is lower than
0.5
you need to increment thetails
counter - When the number is
0.5
and up you must increment theheads
counter
let heads = 0;
let tails = 0;
for (x = 1; x <= 10; x++) {
if (Math.random() < 0.5) {
tails++;
} else {
heads++;
}
}
console.log("Tossed the coin ten times");
console.log(`Number of heads: ${heads}`);
console.log(`Number of tails: ${tails}`);
The example above shows where the for
loop offers the most effective approach. Now let’s see an alternative exercise about coin flip where for
is not effective:
Find out how many times you need to flip a coin until it lands on head.
Alright, now you don’t know how many times you need to flip the coin. This is where you need to use the while loop statement:
let flipResult = "";
let tossCount = 0;
while (flipResult !== "heads") {
flipResult = Math.random() < 0.5 ? "tails" : "heads";
tossCount++;
}
console.log(`The random result is ${flipResult}`);
console.log(`The number of toss: ${tossCount}`);
Learn more about the while
loop here