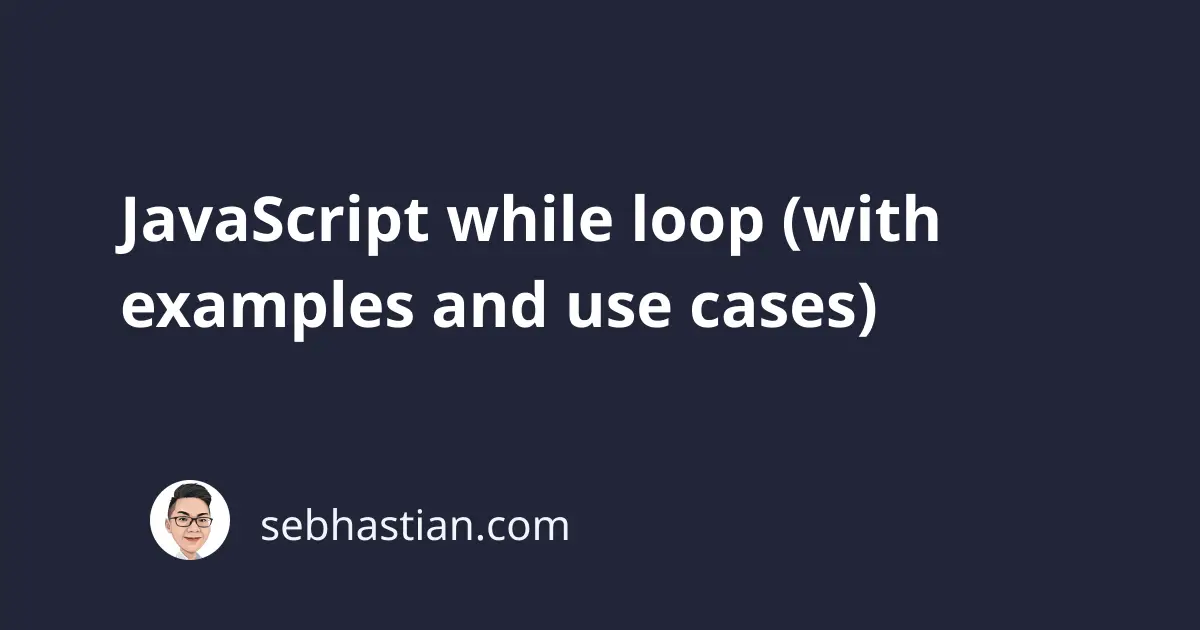
The while
statement is used to create a loop that continues to execute the statement as long as the condition evaluates to true
. You are required to write both the condition and the statement.
Here’s the basic structure of the code:
while (condition) {
statement;
}
Just like the for
statement, the while
loop is used to execute a piece of code over and over again until it reaches the desired condition.
The example below will keep executing the statement
block until the condition
expression returns false
:
let i = 0;
while (i < 6) {
console.log(`The value of i = ${i}`);
i++;
}
Keep in mind that you need to include a piece of code that eventually turns the evaluating condition to false
or the while
loop will be executed forever. The example below will cause an infinite loop:
let i = 0;
while (i < 6) {
console.log(`The value of i = ${i}`);
}
When to use while
over for
loop?
Seeing that both while
and for
can be used for executing a piece of code repeatedly, when should you use while
loop instead of for
?
An easy way to know when you should use while
is when you don’t know how many times you need to execute the code.
Here’s a perfect example of a loop that can only be done with while
:
You are required to random
a number between 1 and 10 until you get the number 8. You also need to show how many times you execute the random
method until number 8 is returned:
let randomResult = 0;
let loopCount = 0;
while (randomResult !== 8) {
randomResult = Math.floor(Math.random() * (10 - 1 + 1) + 1);
loopCount++;
}
console.log(`The random result: ${randomResult}`);
console.log(`The number of loops: ${loopCount}`);
Because you can’t know how many times you need to loop until you get the number 8, you need to use a while
loop instead of a for
loop.