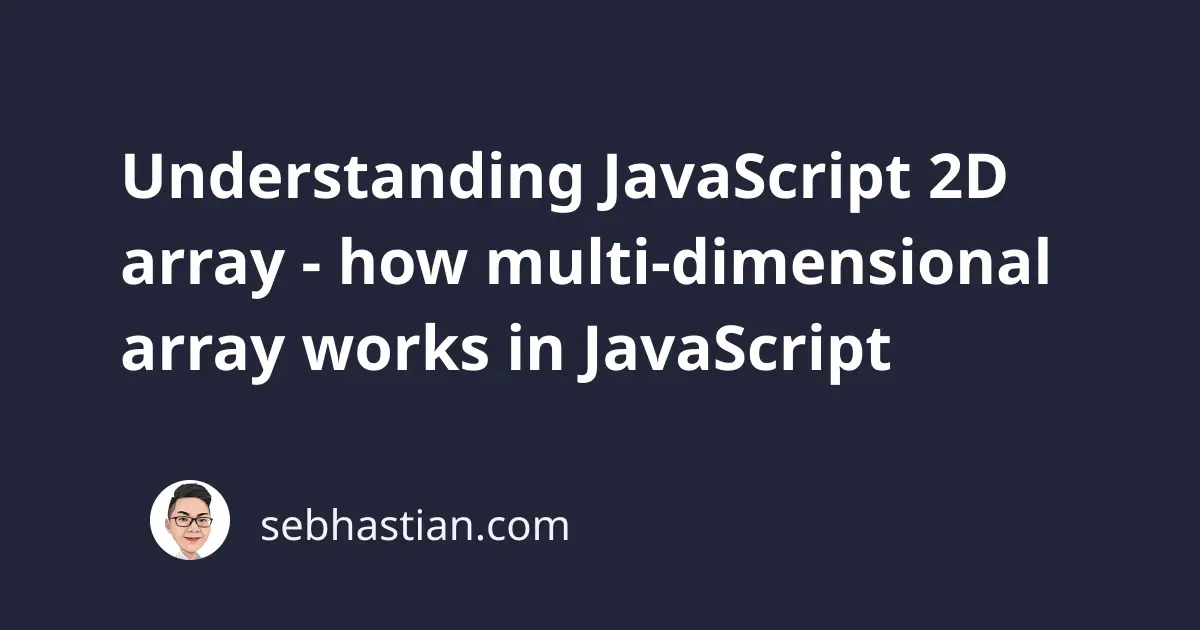
A multi-dimensional array is an array that contains another array. To create one, you simply need to write an array inside an array literal (the square bracket)
The following example shows how you can create a two-dimensional array:
let val = [[5, 6, 7]];
To access the array, you just need to call the variable with two array indices. The first index is for the outer array, and the second index is for the inner array:
let val = [[5, 6, 7]];
console.log(val[0][0]); // 5
console.log(val[0][1]); // 6
console.log(val[0][2]); // 7
As you can see from the example above, the array [5, 6, 7]
is stored inside index 0
of the outer []
array. You can add more elements inside the array as follows:
let val = [[5, 6, 7], [10], [20]];
console.log(val[1][0]); // 10
console.log(val[2][0]); // 20
A multi-dimensional array is not required to have the same array length as can be seen above. Although you can create even a three or four-dimensional array, it’s not recommended to create more than a two-dimensional array because it will be confusing.
Notice how difficult it is to read and access the value [23]
inside the three-dimensional array below:
let val = [[5, 6, 7, [23]]];
console.log(val[0][3][0]); // 23
Finally, you can still use JavaScript Array
object methods like push()
, shift()
, and unshift()
to manipulate the multi-dimensional array:
let val = [[5, 6, 7, [23]]];
val.push([50]);
console.log(val); // [[5, 6, 7, [23]], [50]]
val.shift();
console.log(val); // [[50]]
val.unshift("A string");
console.log(val); // ["A string", [50]]
A multi-dimensional array has no special methods compared with one-dimensional arrays. Often, it’s used to store a group of data as one array.
The following example shows how to group name
and age
value under a multi-dimensional array:
let users = [
["Nathan", 28],
["Jack", 23],
["Alex", 30],
];
Unless you are restricted to use an array, it’s better to use an array of objects to store your data:
let users = [
{ name: "Nathan", age: 28 },
{ name: "Jack", age: 23 },
{ name: "Alex", age: 30 },
];
And that will be everything about a multi-dimensional array.