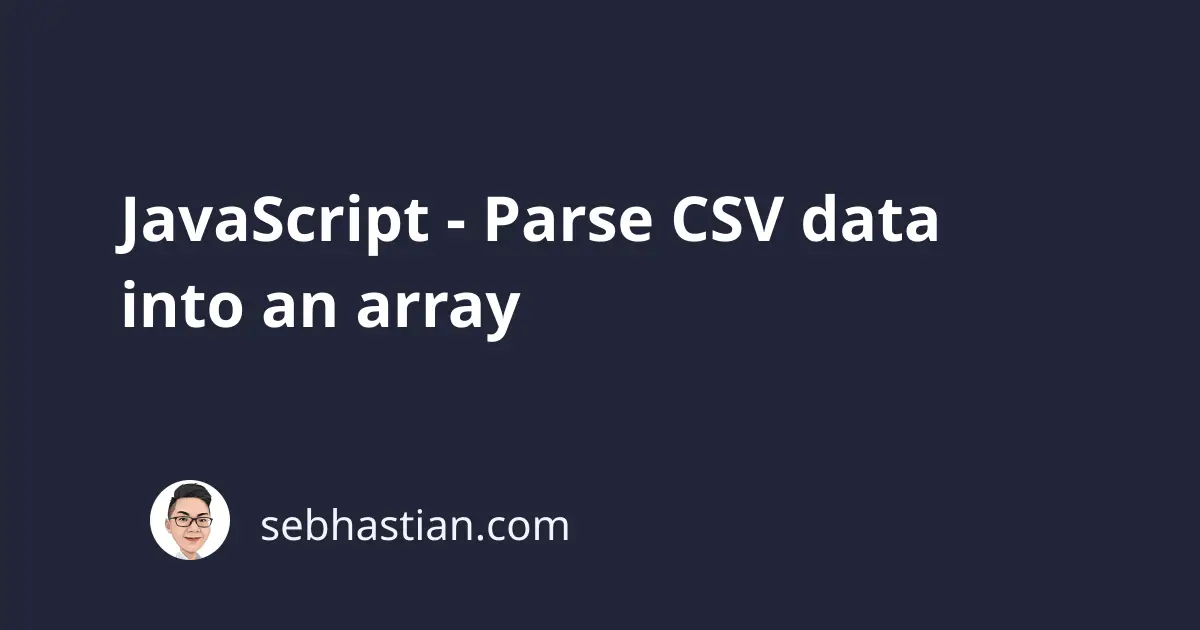
This tutorial will help you learn how to receive a CSV file using HTML <input>
element and parse the content as a JavaScript array.
To convert or parse CSV data into an array
, you need to use JavaScript’s FileReader
class, which contains a method called readAsText()
that will read a CSV file data and parse the result as a string
text.
The FileReader
class is a web API, so this solution only works in the browser. If you need to parse CSV file from Node.js, see my JavaScript read CSV guide.
Once you have the string
, you can create a custom function to turn the string into an array
.
For example, a CSV file with the following content:
name,role,country
Sarene,Help Desk Operator,Thailand
Olvan,Nurse Practicioner,China
Janos,Cost Accountant,China
Dolph,Assistant Manager,China
Ariela,Database Administrator I,Azerbaijan
Lane,Environmental Tech,Indonesia
Griselda,Senior Quality Engineer,Portugal
Manda,Physical Therapy Assistant,Brazil
Leslie,Information Systems Manager,Japan
Aleen,Cost Accountant,Canada
Will be converted into the following JavaScript array:
const arr = [
{ name: "Sarene", role: "Help Desk Operator", country: "Thailand" },
{ name: "Olvan", role: "Nurse Practicioner", country: "China" },
{ name: "Janos", role: "Cost Accountant", country: "China" },
{ name: "Dolph", role: "Assistant Manager", country: "China" },
{ name: "Ariela", role: "Database Administrator I", country: "Azerbaijan" },
{ name: "Lane", role: "Environmental Tech", country: "Indonesia" },
{ name: "Griselda", role: "Senior Quality Engineer", country: "Portugal" },
{ name: "Manda", role: "Physical Therapy Assistant", country: "Brazil" },
{ name: "Leslie", role: "Information Systems Manager", country: "Japan" },
{ name: "Aleen", role: "Cost Accountant", country: "Canada" },
];
The code for this tutorial is shared here.
First, let’s see how to accept a CSV file from the browser using HTML elements.
You need to have an HTML form that accepts a CSV file using an <input>
element. Here’s a simple way to create one:
<body>
<form id="myForm">
<input type="file" id="csvFile" accept=".csv" />
<br />
<input type="submit" value="Submit" />
</form>
</body>
Now that you have the HTML elements ready, it’s time to write a script that will listen for the form’s submit
event.
Just right under the <form>
tag, write a <script>
tag with the following content:
<script>
const myForm = document.getElementById("myForm");
const csvFile = document.getElementById("csvFile");
myForm.addEventListener("submit", function (e) {
e.preventDefault();
console.log("Form submitted");
});
</script>
First, you need the e.preventDefault()
code to prevent the browser’s default submit behavior, which will refresh the page. After that, you can write the code to execute when submit
event is triggered by the user.
You need to take the uploaded CSV file using JavaScript like this:
const myForm = document.getElementById("myForm");
const csvFile = document.getElementById("csvFile");
myForm.addEventListener("submit", function (e) {
e.preventDefault();
const input = csvFile.files[0];
});
Then, you need to create a new instance of FileReader
class using the following code:
const reader = new FileReader();
First, you need to define what happens when the reading operation has been completed with the onload
event handler. The result of the reading operation is passed to the event.target.result
property as follows:
const reader = new FileReader();
reader.onload = function (event) {
console.log(event.target.result); // the CSV content as string
};
Then you can instruct the reader
to read a specific file as follows:
reader.readAsText(file);
Now that you know how JavaScript FileReader
works, let’s put the code together to read the uploaded CSV file. The full HTML page code will be as follows:
<head> </head>
<body>
<form id="myForm">
<input type="file" id="csvFile" accept=".csv" />
<br />
<input type="submit" value="Submit" />
</form>
<script>
const myForm = document.getElementById("myForm");
const csvFile = document.getElementById("csvFile");
myForm.addEventListener("submit", function (e) {
e.preventDefault();
const input = csvFile.files[0];
const reader = new FileReader();
reader.onload = function (e) {
const text = e.target.result;
document.write(text);
};
reader.readAsText(input);
});
</script>
</body>
You can test the code using mock_data.csv
file provided in the GitHub repo.
You will see the CSV content rendered on your browser. This means the <script>
has been able to read the CSV file content as a string without any problem. You just need to parse this string as an array of objects next.
Parsing CSV string into an array
To parse a CSV string into an array, you need to write a code that will separate the string between CSV headers and CSV rows. Then, you need to put each row as one object element, using the headers as the property names and the row as the values.
First, create a new function called csvToArray()
that accepts two parameters:
- A string of CSV content
- The delimiter (or separator) of the CSV content, usually a comma
,
Here’s the function syntax:
function csvToArray(str, delimiter = ",") {}
In this function, you need to create two arrays called headers
and rows
. The headers
will contain the first row of the CSV file, while the rows
will contain all the values, from the second row to the last.
This can be achieved by first slicing the string, then use the split()
method to split a string into an array.
Here’s the code to do so:
function csvToArray(str, delimiter = ",") {
// slice from start of text to the first \n index
// use split to create an array from string by delimiter
const headers = str.slice(0, str.indexOf("\n")).split(delimiter);
// slice from \n index + 1 to the end of the text
// use split to create an array of each csv value row
const rows = str.slice(str.indexOf("\n") + 1).split("\n");
}
Once you have both the headers
and the rows
, it’s time to create the array of objects. First, you need to map the rows
array and split()
the values from each row into an array.
Then, you need to use the reduce()
method on the headers
array, returning an object with each header
as the property name and the data from values
at the same index as the property value.
Finally, you just need to return each mapped row as the array element. The full function code is as follows:
function csvToArray(str, delimiter = ",") {
// slice from start of text to the first \n index
// use split to create an array from string by delimiter
const headers = str.slice(0, str.indexOf("\n")).split(delimiter);
// slice from \n index + 1 to the end of the text
// use split to create an array of each csv value row
const rows = str.slice(str.indexOf("\n") + 1).split("\n");
// Map the rows
// split values from each row into an array
// use headers.reduce to create an object
// object properties derived from headers:values
// the object passed as an element of the array
const arr = rows.map(function (row) {
const values = row.split(delimiter);
const el = headers.reduce(function (object, header, index) {
object[header] = values[index];
return object;
}, {});
return el;
});
// return the array
return arr;
}
With that, your csvToArray()
function is finished. You just need to call the function from the onload
event:
reader.onload = function (e) {
const text = e.target.result;
const data = csvToArray(text);
document.write(JSON.stringify(data));
};
You can view the full HTML code in the GitHub repo
Conclusion
You’ve just learned how to create a JavaScript array out of a CSV file uploaded through the HTML <input>
form. At other times, you may want to parse a CSV array fetched from an API or remote URL as an array.
Depending on the data returned from your request, you can either use the FileReader
to read the CSV content as a string first, or you just need to parse the string as an array if you already receive a string from your API.
Also, pay attention to the delimiter
of your CSV file. The csvToArray()
function already has a sensible default delimiter
which is a comma, but you may use other symbols. If that’s so, you can pass the right delimiter
as the second argument to the function call.
Feel free to modify the code examples to fit your requirements 😉