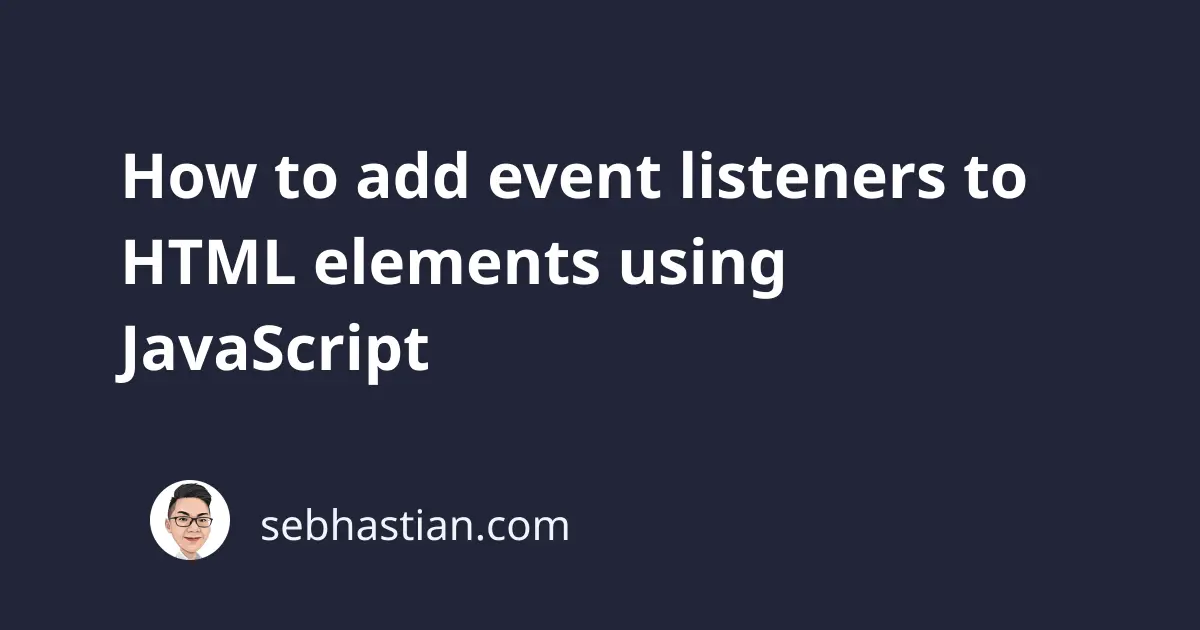
JavaScript allows you to attach event listeners to HTML elements so that you can run a certain function when an event is triggered. Some examples of events are when a button is “clicked” or when the mouse pointer is moved to touch the element.
The built-in method addEventListener()
allows you to attach an event listener to an HTML element. It accepts two parameters: the event type
to listen to and the callback
function to run when the event is triggered:
Element.addEventListener(type, callbackFn);
For example, suppose you want to display an alert()
box when a <button>
element is clicked. Here’s how you do it:
<body>
<button id="save">Save</button>
<script>
let button = document.getElementById("save");
function fnClick(event) {
alert("Button save is clicked");
}
button.addEventListener("click", fnClick);
</script>
<body></body>
</body>
First, the button element is selected using document.getElementById()
method, then the method addEventListener()
is called on the element. First, you specify the type
of event to listen, which is click
in this case. Next, you specify the callback
function reference.
In the code above, the fnClick
function will be called when the click
event is triggered. You can also add multiple event listeners to the same element without overwriting the previous event listener.
The following example shows how the click
event will execute both event listeners, ordered from top to bottom. The fnClick
function will be called first, then the exampleClick
function:
<body>
<button id="save">Save</button>
<script>
let button = document.getElementById("save");
function fnClick(event) {
alert("Button save is clicked");
}
function exampleClick(event) {
alert("Hello World!");
}
button.addEventListener("click", fnClick);
button.addEventListener("click", exampleClick);
</script>
<body></body>
</body>
The full list of HTML event type can be found here
You can also remove event listeners you’ve attached to elements using the removeEventListener()
method.