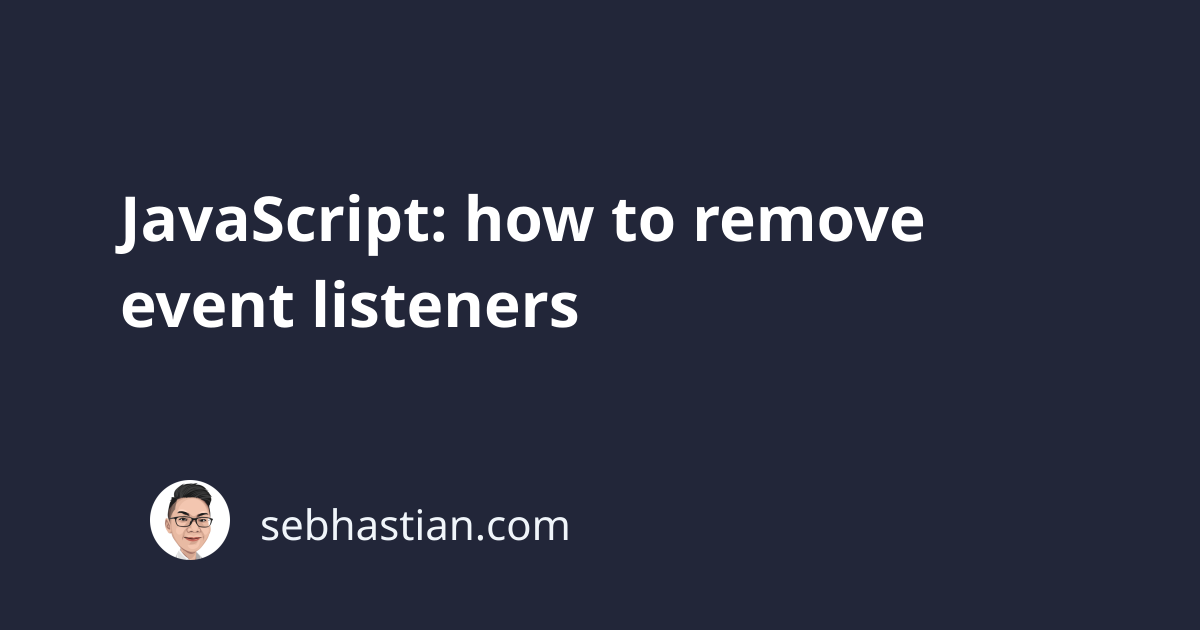
JavaScript provides a built-in function called removeEventListener()
that you can use to remove event listeners attached to HTML elements. Suppose you have an event listener attached to a <button>
element as follows:
<body>
<button id="save">Save</button>
<script>
let button = document.getElementById("save");
function fnClick(event) {
alert("Button save is clicked");
}
button.addEventListener("click", fnClick);
</script>
<body></body>
</body>
To remove the "click"
event listener attached from the <script>
tag above, you need to use the removeEventListener()
method, passing the type
of the event and the callback
function to remove from the element:
button.removeEventListener("click", fnClick);
The above code should suffice to remove the "click"
event listener from the button
element. Notice how you need to call the removeEventListener()
method on the element while also passing the function fnClick
reference to the method.
To correctly remove an event listener, you need a reference both to the element with a listener and the callback
function reference.
This is why it’s not recommended to pass a nameless callback
function to event listeners as follows:
button.addEventListener("click", function(event){
alert("Button save is clicked");
})
Without the callback
function name as in the example above, you won’t be able to remove the event listener.
Removing event listener after click
Sometimes, you may also want to disable the button element and remove the event listener to prevent a double-click from your users. You can do so by writing the removeEventListener()
method inside the addEventListener()
method as shown below:
<body>
<button id="save">Save</button>
<script>
let button = document.getElementById("save");
function fnClick(event) {
alert("Button save is clicked");
button.disabled = true; // disable button
button.removeEventListener("click", fnClick); // remove event listener
}
button.addEventListener("click", fnClick);
</script>
</body>
In the code above, the button
element will be disabled and the event listener will be removed after a "click"
event is triggered.
And that’s how you remove JavaScript event listeners attached to HTML elements.
You need to keep references to the element you want to remove the listener from, the type
of the event, and the callback
function executed by the event so that you can remove the event listener without any error.