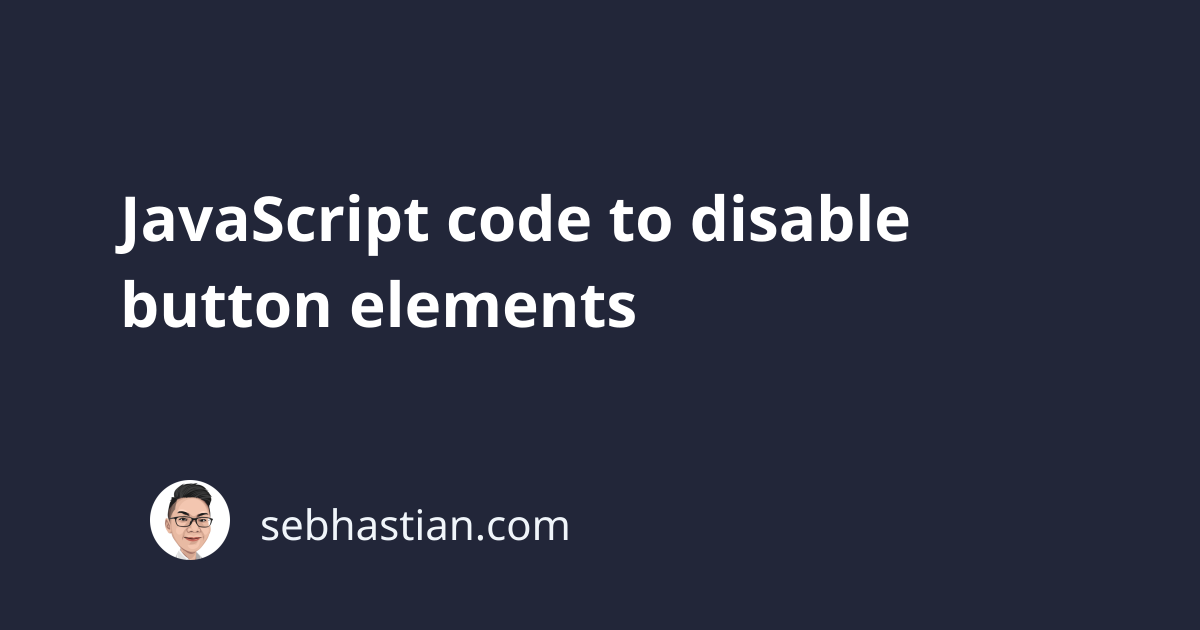
When you need to disable buttons using JavaScript, you can update the disabled
attribute of <button>
elements to true
.
Every HTML <button>
element has the disabled
attribute which is set to false
by default, even when the <button>
doesn’t have a disabled
attribute present. You can test this by running the following HTML code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>JavaScript disable button</title>
</head>
<body>
<button id="testBtn">Click me</button>
<script>
console.log(document.getElementById("testBtn").disabled); // false
</script>
</body>
</html>
That’s why when you need to disable or enable a <button>
element, all you have to do is change the value of the disabled
attribute:
document.getElementById("testBtn").disabled = true; // disable the button
Disabling multiple buttons with JavaScript
The same technique also applies when you want to disable many buttons on your HTML page. First, You need to select the buttons using the document.getElementsByTagName()
method:
const buttons = document.getElementsByTagName("button");
The getElementsByTagName()
method will return a collection of Then, you need to iterate through the buttons and set the disabled
attribute of each button to false
. You can use the for
loop to do so:
const buttons = document.getElementsByTagName("button");
for (let i = 0; i < buttons.length; i++) {
buttons[i].disabled = true;
}
Here’s an example of HTML <body>
tag that you can run on your browser:
<body>
<button>Log out</button>
<button>Profile</button>
<button>friends</button>
<script>
const buttons = document.getElementsByTagName("button");
for (let i = 0; i < buttons.length; i++) {
buttons[i].disabled = true;
}
</script>
</body>
Disable button after click
The last thing you may want to do is to disable the <button>
after the user clicked it to prevent double clicks. You just need to wrap the disabling code in a function
and pass it to the onclick
attribute of your <button>
element:
<body>
<button id="saveBtn" onclick="disableBtn()">Click me!</button>
<script>
function disableBtn() {
document.getElementById("saveBtn").disabled = true;
}
</script>
</body>
The code above will disable the saveBtn
once it has been clicked. Feel free to modify it to suit your requirements.