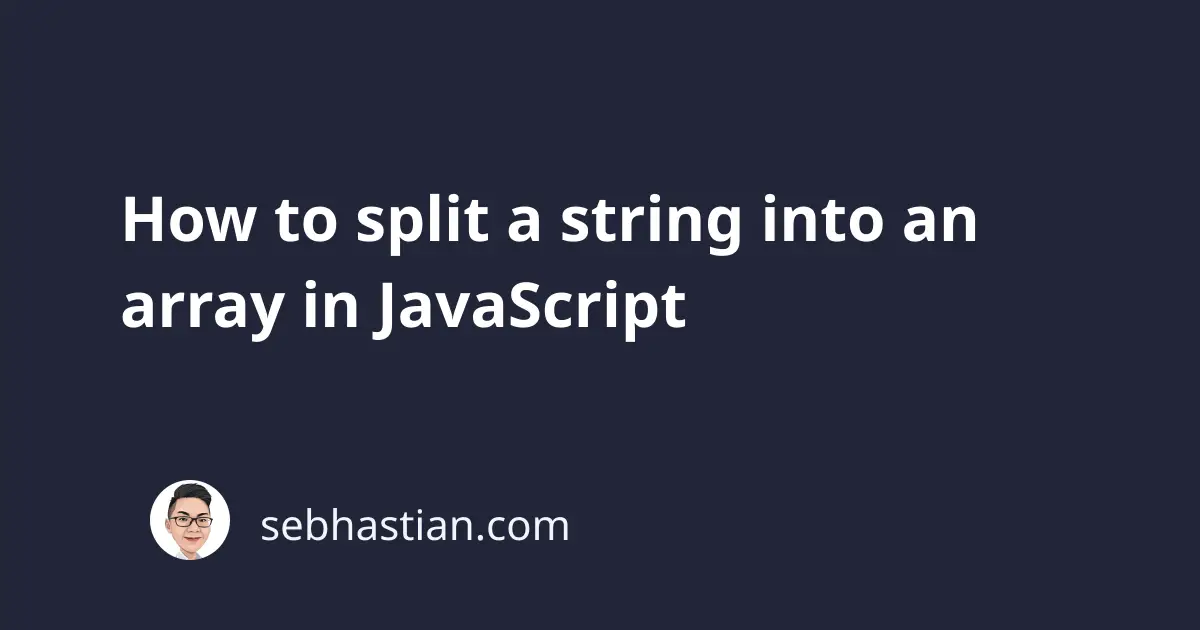
Sometimes, you need to create an array that represents a string in your JavaScript code.
You can split a string into an array with the split()
method, which is a built-in method of the String
object.
The split()
method accepts two arguments:
separator
— Optional — specifies the character or regular expression used for splitting the string. If not present, the method will return a single arraylimit
— Optional — specifies the integer used for limiting the returned array. The array length for the returned value will be capped at this number
This article will show you examples of using the split()
method.
1. Split a string for each character in the string
If you pass an empty string as an argument to the split()
method, it will split the string for each character in that string:
let str = "Banana";
let arr = str.split("");
console.log(arr);
Output:
> Array ["B", "a", "n", "a", "n", "a"]
2. Other examples of splitting a string into an array
You can use the split()
method for any kind of value as long as it’s a string:
"Apple".split(); // ["Apple"]
"Apple".split("", 3); // ["A", "p", "p"]
true.split(""); // Uncaught TypeError: true.split is not a function
If you have a string with commas, you can pass them as the separator
argument for the split
method:
let arr = "Banana,Apple,Grape,Melon".split(",");
console.log(arr); // ["Banana", "Apple", "Grape", "Melon"]
Or if your string is separated by white spaces, you can put that as the method argument:
let arr = "Banana Apple Grape Melon".split(" ");
console.log(arr); // ["Banana", "Apple", "Grape", "Melon"]
Now you’ve learned how to split a string into an array in JavaScript. Great work! 😉
If you want to know how you can convert an array into a string, I’ve written another article showing different ways you can convert a JavaScript array to string.
Happy coding! 👋