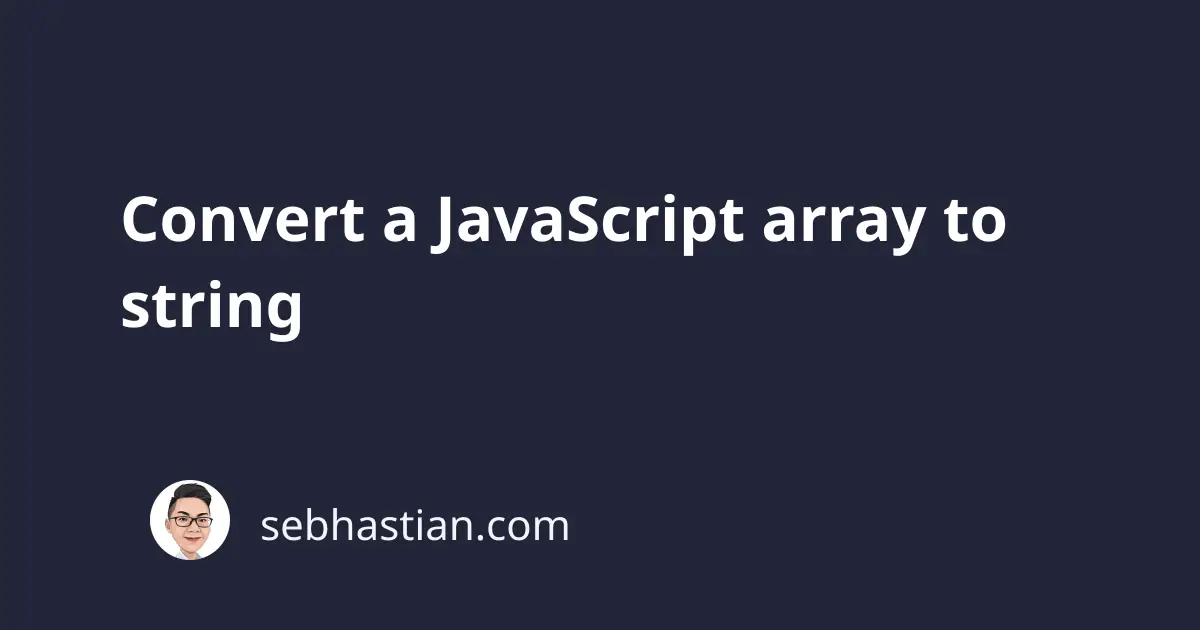
There will be times when you need to convert an array of values into a single string in JavaScript.
This article will help you learn the easy ways you can convert an array to string in JavaScript:
1. Using the toString() method
To easiest way to convert a JavaScript array to string is to use the built-in Array
method called toString()
.
This method will return a string that represents the elements stored in your array as follows:
let numbers = [0, 1, 2, 3];
let numbersToString = numbers.toString();
console.log(numbersToString);
// output is "0,1,2,3"
The toString()
method is able to convert an array of strings into a single string as well:
let fruits = ["Orange", "Grape", "Apple"];
let newString = fruits.toString();
console.log(newString);
// output is "Orange,Grape,Apple"
2. Convert an array of objects to string with JSON.stringify()
But keep in mind that the toString()
method can’t be used to convert an array of objects.
when you call the toString()
method on an array of objects, it will return [object Object]
instead of the actual values:
let users = [{ name: "John" }, { name: "Lisa" }];
let usersToString = users.toString(); // ❌
console.log(usersToString);
// output: [object Object],[object Object]
To convert an array of objects into a string, you need to use the JSON.stringify
method as shown below:
let users = [{ name: "John" }, { name: "Lisa" }];
let usersToString = JSON.stringify(users); // ✅
console.log(usersToString);
// '[{"name":"John"},{"name":"Lisa"}]'
It’s not really a representation of the array values because the return value will still have the square and curly brackets.
Still, this is the best way you can convert an array of objects to a string in JavaScript.
3. Convert JavaScript array to string with join()
Sometimes, you want to convert an array into a string without commas.
Since the toString()
method can’t do this, you need to use join()
method instead.
The join()
method is a built-in method of the Array
class used to join array elements as one string.
This method accepts one string
parameter which will serve as the separator of the returned output.
Here are some examples of using the join()
method to convert an array to a string:
// Normal join with comma separator:
["a", 2, 3].join(); // "a,2,3"
// Using + as separator:
["a", 2, 3].join("+"); // "a+2+3"
// Using white space as separator:
["a", 2, 3].join(" "); // "a 2 3"
// use empty string for no separator:
["a", 2, 3].join(""); // "a23"
As you can see, you can specify an empty string to get a string without commas.
4. Convert a nested array to a string in JavaScript
You can convert a nested array into a string in JavaScript using the toString()
method.
The toString()
method will flatten your array so that all elements will be joined as a single string:
let nestedArray = ["Jack", ["James", "Nathan"]];
let arrayToString = nestedArray.toString();
console.log(arrayToString); // Jack,James,Nathan
As you can see, the child array that contains ["James", "Nathan"]
is flattened and put into a single string by the toString()
method.
5. Convert a string back into an array
When you need to convert a JavaScript string into an array, you can use a built-in String
method called split()
.
As the name implies, the split()
method is used to split a JavaScript string into an array.
You can pass a separator as an argument of split()
to let JavaScript know what character is used to split the string.
See the following code:
let myString = "Jack,James,Nathan"
let stringToArray = myString.split(",");
console.log(stringToArray);
// [ 'Jack', 'James', 'Nathan' ]
As you can see, converting a string into an array is very easy in JavaScript.
If you want to learn more about the split()
method, check out my other article on How to split a string into array in JavaScript.
Conclusion
As you can see from the examples in this article, there are multiple ways you can convert a JavaScript array to a string.
It all depends on your needs and preferences. Sometimes you need to convert an array of objects. Other times you might want to use other than a comma as your string separator.
You can also convert a string back to an array using the split()
method.
I hope this article was helpful. See you in other articles! 👍