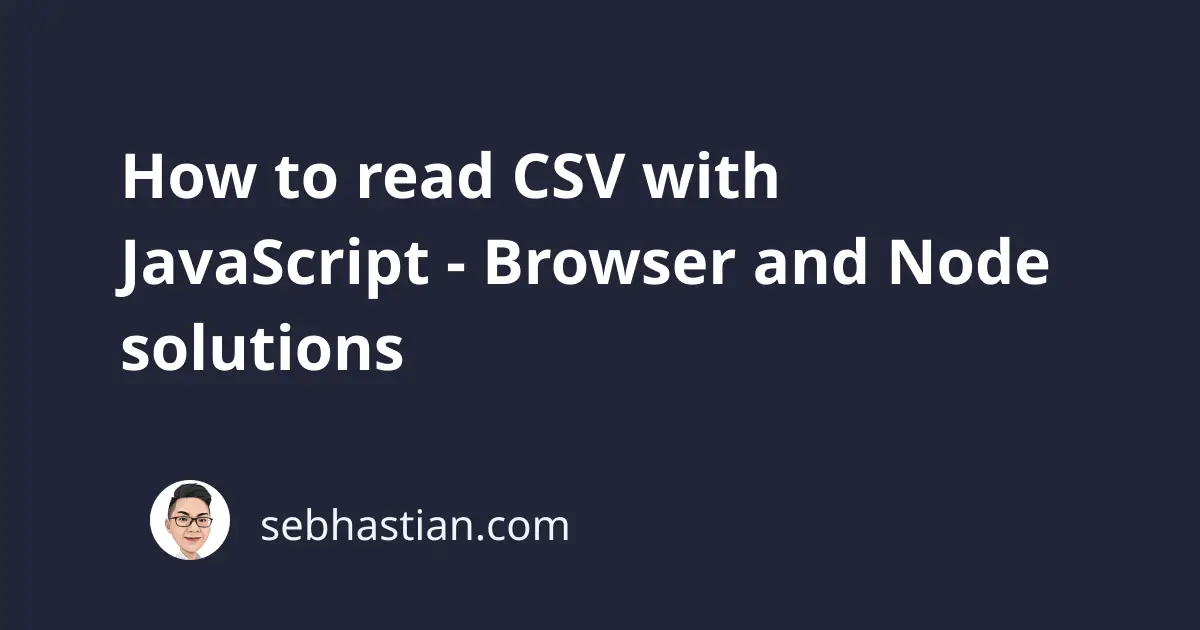
CSV files are text files that contain comma-separated values. The CSV format is flexible and language-independent, so it can be processed using any programming language.
This tutorial will help you learn how to read CSV data with JavaScript code.
There are two ways to read CSV based on where you read the file from:
- From the Node.js environment
- From the browser
You will learn how to read CSV files using both third-party and native modules.
Let’s see how to read CSV files and parse them into data from the Node.js environment first.
Read CSV data in Node.js environment (using csv-parse)
To read a CSV file from Node.js environment, you can use the csv-parse
module from npm.
The csv-parse is a reliable CSV parser module that has been used for several years by Node developers. It’s a part of the node-csv module, which is a set of libraries used to manipulate CSV files using Node.js.
You will also need the native fs
module to help you get a file from the file system.
First, install csv-parse
on your project with npm:
npm install csv-parse
Next, import both fs
and csv-parse
modules into your JavaScript file:
const fs = require("fs");
const { parse } = require("csv-parse");
To read a CSV file using csv-parse
, you need to create a readable stream using the fs.createReadStream()
function.
The following example code shows how to create a readable stream with fs
. Replace the ./example.csv
parameter with the path to your CSV file:
fs.createReadStream("./example.csv");
Next, pipe the stream data as an input to the parse()
function as shown below:
fs.createReadStream("./example.csv")
.pipe(parse({ delimiter: ",", from_line: 2 }))
.on("data", function (row) {
console.log(row);
})
.on("error", function (error) {
console.log(error.message);
})
.on("end", function () {
console.log("finished");
});
The .on("data")
event is where each row in your CSV file will be read.
The row
is an array filled with your CSV data. Suppose you have an example.csv
file with the following content:
Name, Age, Country
Jack, 22, Australia
Jane, 27, United Kingdom
Andrew, 29 , United States
Mary, 19 , France
When you run the JS file using node, here’s the result:
$ node index.js
[ 'Jack', ' 22', ' Australia' ]
[ 'Jane', ' 27', ' United Kingdom' ]
[ 'Andrew', ' 29 ', ' United States' ]
[ 'Mary', ' 19 ', ' France' ]
finished
Alternatively, you can generate an object for each CSV row where the data is formatted as key-value pair. The first row (header) will be used as the keys.
To do so, you only need to change the options passed to the parse()
method as shown below:
const fs = require("fs");
const { parse } = require("csv-parse");
fs.createReadStream("./example.csv")
.pipe(
parse({
delimiter: ",",
columns: true,
ltrim: true,
})
)
By adding the columns
option, the first line will be considered as column names, while subsequent lines will be the values.
The ltrim
option is used to trim the whitespace from your data.
Next, create an empty array variable named data
, and push each row into the array.
Once all data has been processed, log the result:
const data = [];
fs.createReadStream("./example.csv")
.pipe(
parse({
delimiter: ",",
columns: true,
ltrim: true,
})
)
.on("data", function (row) {
// 👇 push the object row into the array
data.push(row);
})
.on("error", function (error) {
console.log(error.message);
})
.on("end", function () {
// 👇 log the result array
console.log("parsed csv data:");
console.log(data);
});
The result will be as follows:
$ node index.js
parsed csv data:
[
{ Name: 'Jack', Age: '22', Country: 'Australia' },
{ Name: 'Jane', Age: '27', Country: 'United Kingdom' },
{ Name: 'Andrew', Age: '29 ', Country: 'United States' },
{ Name: 'Mary', Age: '19 ', Country: 'France' }
]
And that’s how you read a CSV file using JavaScript in Node.js environment.
Let’s see how you can read CSV files without csv-parse
next.
Read CSV file with Node.js native module
To read a CSV file without using a module like csv-parse
, you need to use the native Node modules fs
and readline
.
To read the whole file input at once, use the fs.readFile()
method as shown below:
const fs = require("fs");
fs.readFile("./example.csv", "utf8", function (err, data) {
console.log(data);
});
// 👇 the CSV string output:
// Name, Age, Country
// Jack, 22, Australia
// Jane, 27, United Kingdom
// Andrew, 29 , United States
// Mary, 19 , France
The output will be a string
that you can further process whether as an array or object.
You can also read the file a row at a time by using fs.createReadStream()
and readline
modules.
In the following code example, a readable stream is sent into the readline.createInterface()
method:
const fs = require("fs");
const readline = require("readline");
const stream = fs.createReadStream("./example.csv");
const reader = readline.createInterface({ input: stream });
let data = [];
reader.on("line", row => {
// 👇 split a row string into an array
// then push into the data array
data.push(row.split(","));
});
reader.on("close", () => {
// 👇 reached the end of file
console.log(data);
});
// 👇 log output:
// [
// [ 'Name', ' Age', ' Country' ],
// [ 'Jack', ' 22', ' Australia' ],
// [ 'Jane', ' 27', ' United Kingdom' ],
// [ 'Andrew', ' 29 ', ' United States' ],
// [ 'Mary', ' 19 ', ' France' ]
// ]
The readline
interface will read each line and trigger the line
event.
When the reading is done, readline will execute the close
event function.
Although you can read a CSV file without using a third-party module, you will need extra work to format the output of the read process.
In the example above, the header row is processed just like the data rows. You need to write more code to format the CSV text better.
What’s more, the native code won’t work when you have edge cases, such as no line break between values (only one row in the file)
A module like csv-parse
has been tested and refined through the years to handle parsing CSV well.
Unless you really have to write your own solution, it’s better to use csv-parse
.
Read CSV file from the browser with D3.js
To read a CSV file from the browser, you need to use a different library named D3.js.
D3.js is a data manipulation library used to process data using JavaScript. One of its features is to read CSV files.
To use D3.js, you can download the latest release or import the script from a CDN.
Call the csvParse()
method and pass a string as shown below:
<script src="https://cdn.jsdelivr.net/npm/d3@7"></script>
<script>
const res = d3.csvParse("Name,Age\nJane,29\nJoe,33");
console.log(res);
</script>
The output will be an array of objects like this:
[
{ Name: "Jane", Age: 29 },
{ Name: "Joe", Age: 33 },
];
But if you’re parsing CSV file from the browser, then you usually need to receive a CSV file using the HTML <input>
element.
You can use the FileReader
API and D3.js to read a CSV file using the following code:
<body>
<form id="myForm">
<input type="file" id="csvFile" accept=".csv" />
<br />
<input type="submit" value="Submit" />
</form>
<script src="https://cdn.jsdelivr.net/npm/d3@7"></script>
<script>
const myForm = document.getElementById("myForm");
const csvFile = document.getElementById("csvFile");
myForm.addEventListener("submit", function (e) {
e.preventDefault();
const input = csvFile.files[0];
const reader = new FileReader();
reader.onload = function (e) {
const text = e.target.result;
const data = d3.csvParse(text);
document.write(JSON.stringify(data));
};
reader.readAsText(input);
});
</script>
</body>
First, you need to receive a file using the HTML <form>
:
<form id="myForm">
<input type="file" id="csvFile" accept=".csv" />
<br />
<input type="submit" value="Submit" />
</form>
Then, write an event listener for when the form is submitted using JavaScript.
Here, the uploaded csv file will be processed using D3.js:
const myForm = document.getElementById("myForm");
const csvFile = document.getElementById("csvFile");
myForm.addEventListener("submit", function (e) {
e.preventDefault();
const input = csvFile.files[0];
const reader = new FileReader();
// 👇 executed when a file is loaded
reader.onload = function (e) {
// 👇 get the text from CSV file
const text = e.target.result;
// 👇 parse it using D3.js
const data = d3.csvParse(text);
// 👇 write the output to the browser
document.write(JSON.stringify(data));
};
// 👇 load the input file to the reader
reader.readAsText(input);
});
This is a standard implementation of D3.js to read CSV files from the browser.
For more information, you can look into the csvParse documentation.
Now you’ve learned how to parse CSV file using JavaScript and D3.js from the browser.
For a native solution in the browser, you can see my JavaScript CSV to array guide.
Thanks for reading. I hope this tutorial has been useful for you. 🙏