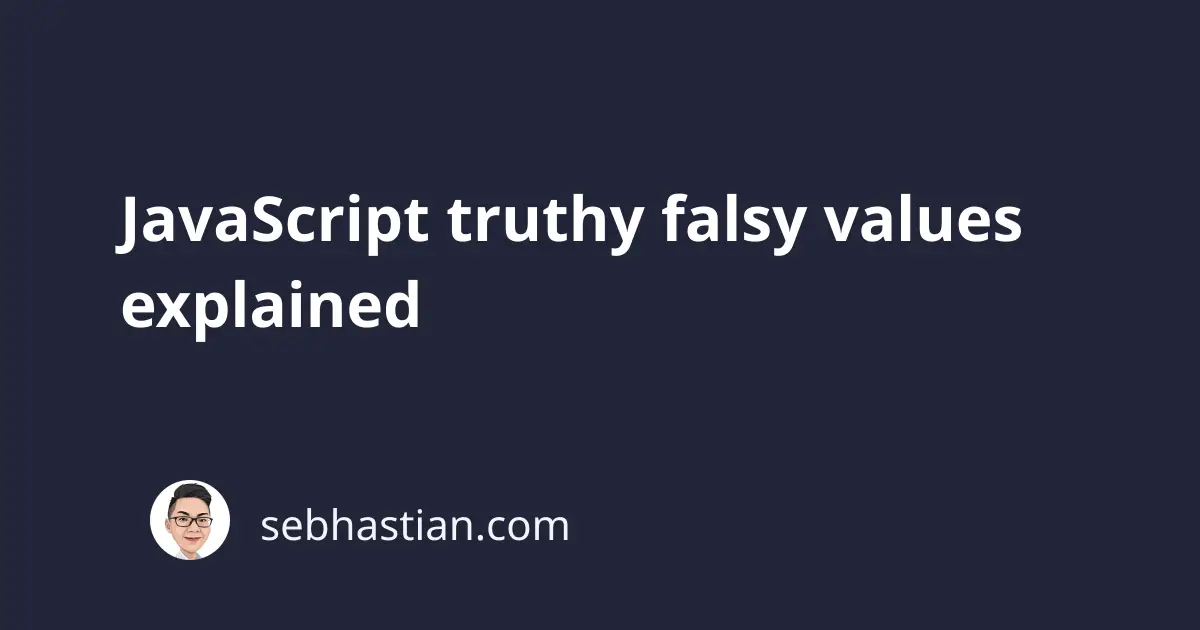
Every value you declare in JavaScript has an inherent Boolean value. Some values will be coerced, or converted to true
and some convert to false
.
You can easily know which one is which when you use if
statement to evaluate the value. For example, an empty string will be coerced as false
if(''){
console.log("The value is truthy");
} else {
console.log("The value is falsy");
}
The console will print “The value is falsy”. But if you put even one character, the value will be true
:
if('a'){
console.log("The value is truthy");
} else {
console.log("The value is falsy");
}
The console will print out “The value is truthy”.
JavaScript value coercion happens in special cases where you need to evaluate the value as either true or false in order to continue the process, such as when you call on an if
statement or the conditional (ternary) operator.
Another example, when you want to find out whether 4
is an even number, you write:
let isEvenNumber = 4%2 === 0 ? true: false;
The equality operator ===
already evaluates to true
or false
, so there’s no coercion here. But what if you just write 4
before the question mark without any equality operator?
let isTrue = 4 ? true: false;
This is when value coercion happens, and 4
is a value that coerces, or converts, to true
Keep in mind that coercion doesn’t change the value of the variable. If you store the value 4
under a variable, that variable is still 4
. It only changes when JavaScript needs a true
or false
value
(for example, the return
statement for JavaScript filter)
let myNumber = 4;
let isTrue = myNumber ? true: false;
console.log(myNumber);
// output is 4 not true
There are only 8 falsy values in JavaScript:
false
0
-0
0n // This is BigInt. It follows the same rule as a Number
""
null
undefined
NaN
The rest of the values are truthy.