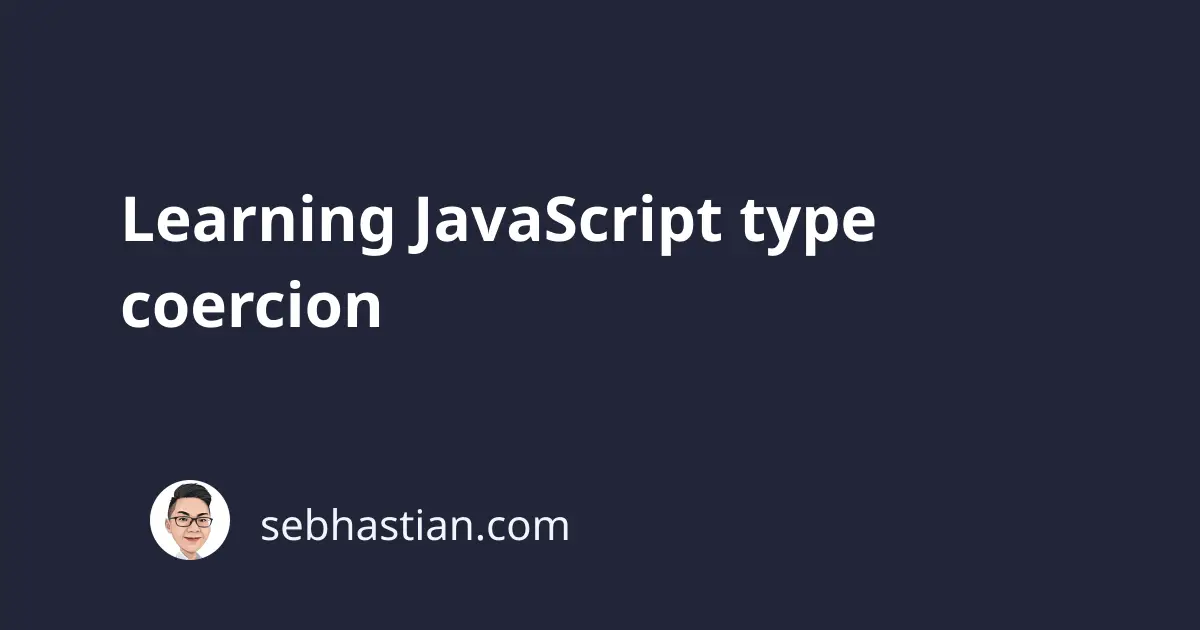
Type coercion in JavaScript is a process where a value of one type is implicitly converted into another type.
This is automatically done by JavaScript so that your code won’t hit any errors. But as you’ll see in this post, type coercion can actually be the cause for bugs.
To understand type coercion well, you need to learn about the type system in general, so we’ll start with that.
What is a type system?
A type system is a set of logical rules that assigns a type (or data type) into various constructs of a computer program.
You can read a lot about type systems on other websites, but suffice to say that the JavaScript type system is the reason why it knows that "1"
is a string
and 1
is a number
:
In the following example, the type system will identify the right data types for each variable accordingly:
let myNumber = 1; // a number
let myString = "1"; // a string
Now, let’s consider what happens when you perform an addition between a number
and a string
:
console.log(1 + "1");
Different programming languages will respond differently to the code above, depending on the rules written in their type system.
For example, programming languages like Ruby or Python will respond by crashing your program and giving an error as feedback. Something along the line of “Cannot perform addition between a number and a string”.
But JavaScript will see this and said: “I cannot do the operation you requested as it is, but I can do it if the number 1
is converted to a string
, so I’ll do just that.”
And that’s exactly what type coercion is. JavaScript notices that it doesn’t know how to execute your code, but it doesn’t stop the program and responds with an error.
Instead, it will change the data type of one of the values without telling you.
In short: the JavaScript type system allows it to perform type coercion instead of responding with an error in certain situations.
Type coercion rules
Type coercion rules are never stated clearly anywhere, but I did find some rules through trying various silly operations myself.
It seems that JavaScript will first convert data types to string
when it finds different data types:
1 + "1" // "11"
[1 ,2] + "1" // "1,21"
true + "1" // "true1"
But the order of the values matter when you have an object. Writing object first always returns numeric 1
:
{ a: 1 } + "1" // 1
"1" + { a: 1 } // "1[object Object]"
true + { a: 1 } // "true[object Object]"
{ a: 1 } + 1 // 1
Here are some more JavaScript coercions that I found. JavaScript can calculate between boolean and numeric types because boolean true
and false
implicitly has the numeric value of 1
and 0
true + 1 // 1+1 = 1
false + 1 // 0+1 = 1
[1,2] + 1 // "1,21"
Type coercion is always performed implicitly. When you assign the value as a variable, the variable type will never change outside of the operation:
let myNumber = 1;
console.log(myNumber + "1"); // prints 11
console.log(myNumber); // still prints number 1 and not string
You can try to find some on your own, but you certainly understand what type coercion is and how it works by now.
Why you should avoid type coercion
JavaScript developers are generally divided into two camps when talking about type coercion:
- Those who think it’s a feature
- Those who think it’s a bug
You are always free to form your own opinion, but if you ask me, I would recommend you to avoid using type coercion in your code all the time.
The reason is that I never found a problem where type coercion is required for the solution, and when I need to convert one type into another, it’s always better to do so explicitly:
let price = "50";
let tax = 5;
let totalPrice = Number(price) + Number(tax);
console.log(totalPrice);
You may think that type coercion allows you to write less code, but type coercion will convert the tax
value into string
instead of converting price
into number
in the code above.
I’ve written another article explaining about JavaScript explicit type conversion if you want to learn more.
Now you’ve learned how type coercion works in JavaScript. Good work!