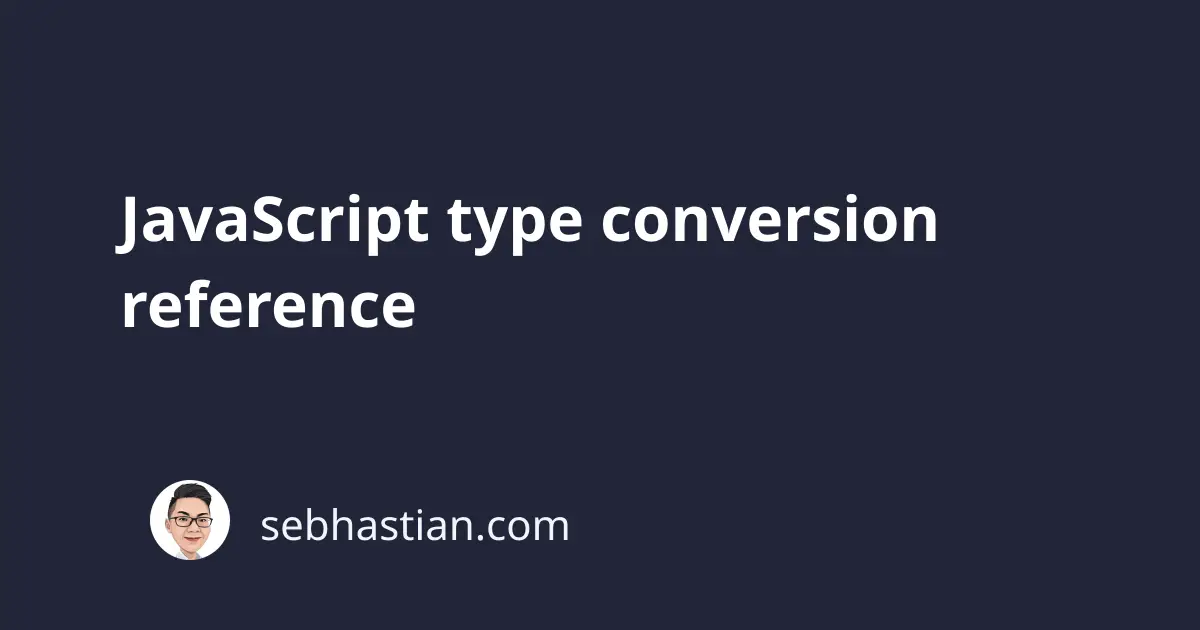
JavaScript provides you with built-in methods to convert values from one type to another. Type conversion is commonly required when you want to perform some kind of operation to the values you have in your program. There are two kinds of type conversion in JavaScript based on how they are performed:
- Implicit conversion, also called type coercion
- Explicit conversion, which is what you will learn in this tutorial
For example, suppose you want to perform an addition between the price
and the tax
variable, but they are declared as a string
type instead of number
type, causing the addition to concatenate the values instead of adding to it:
let price = "20";
let tax = "5";
console.log(price + tax); // "205"
Of course, you can simply change the declaration of both variables as number
types, but for this tutorial, let’s pretend they are unchangeable. What you can do for this situation is to convert those string
type variables into number
types by calling the Number
method.
Here’s how you do it:
let price = "20";
let tax = "5";
console.log(Number(price) + Number(tax)); // 25
And that’s what explicit type conversion is all about. Generally, you can convert data or values from one type to another using one of three built-in JavaScript methods:
Number()
String()
Boolean()
The result of these conversion methods will depend on the value you passed as the argument. In the example above, the string
variables are correctly converted into number
types because they are representative of those numbers. JavaScript will return NaN
as value when you passed letters, words, or sentences into the Number
variable:
Number("hello"); // NaN
Number(false); // 0
Number(true); // 1
String(2); // "2"
String(true); // "true"
String(false); // "false"
Boolean(0); // false
Boolean(1); // true
Boolean(2); // true
Here’s a JavaScript type conversion table for your reference. Please keep in mind that:
- Values wrapped in double quotation marks indicate a
string
type. - Values wrapped in braces indicate an
object
type. - Values wrapped in square brackets indicate an
array
type. true
orfalse
indicates real Boolean values
Value | String Conversion | Number Conversion | Boolean Conversion |
---|---|---|---|
1 | "1" | 1 | true |
0 | "0" | 0 | false |
"1" | "1" | 1 | true |
"0" | "0" | 0 | true |
"ten" | "ten" | NaN | true |
true | "true" | 1 | true |
false | "false" | 0 | false |
null | "null" | 0 | false |
undefined | "undefined" | NaN | false |
'' | "" | 0 | false |
' ' | " " | 0 | true |
[ ] | 0 | "" | true |
[20] | 20 | "20" | true |
[10,20] | NaN | "10,20" | true |
["twenty"] | NaN | "twenty" | true |
["ten","twenty"] | NaN | "ten,twenty" | true |
{} | "[object Object]" | NaN | true |
{name:"Jack"} | "[object Object]" | NaN | true |