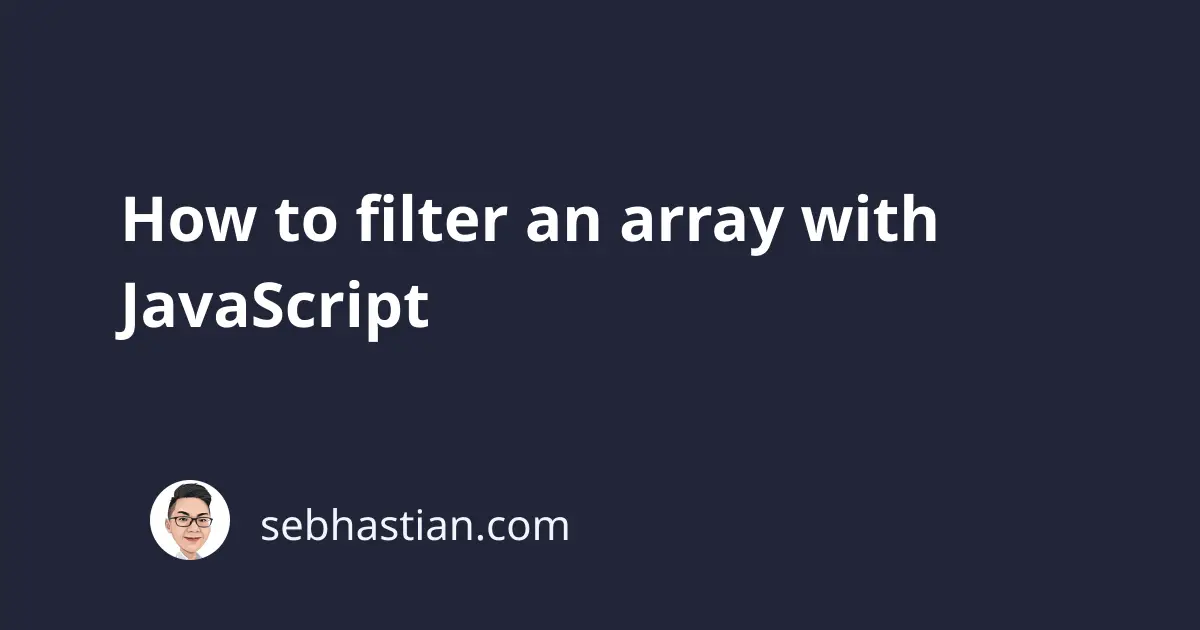
The .filter()
method is a built-in method available for JavaScript array objects that can help you in filtering an array. The syntax of the method is as follows:
arrayObject.filter(callback, thisContext);
The method has two parameters:
callback
- Required - The filtering function that will be executed for each array valuethisContext
- Optional - The value ofthis
keyword inside thecallback
The thisContext
parameter is optional and usually not needed. You only need to define the callback
function, which will accept three arguments:
- The
currentElement
being processed into the method - The
index
of the element that starts from0
- and the
array
object where you callfilter()
filterCallback(currentElement, index, array){
// ...
}
The callback function must include a validation pattern that returns either true
or false
.
Filter method examples
Let’s see an example of .filter()
in action. Let’s say you have an array of stock prices as follows:
let stockPrices = [3, 7, 2, 15, 4, 9, 21, 14];
You want to filter the prices to display only those higher than 5
Here’s how you do it with .filter()
:
let stockPrices = [3, 7, 2, 15, 4, 9, 21, 14];
let filteredPrices = stockPrices.filter(function (currentElement) {
return currentElement > 5;
});
console.log(filteredPrices);
The value of filteredPrices
will be [7, 15, 9, 21, 14]
The filter
method simply evaluates the currentElement
and returns either true
or false
.
If your callback function returns true
, the currentElement
will be added to the result array:
- During the first iteration, the
currentElement
is3
so the callback returnsfalse
- During the second iteration, the
currentElement
is7
so the callback returnstrue
and the value is pushed into the result array - The iteration will continue to the last element
- The resulting array is assigned to
filteredPrices
variable
And that’s how the .filter()
works. You can also filter an array of objects with this method.
Filtering an array of objects
Let’s see another example. Suppose you have an array of stock prices, but this time it’s an object:
let stocks = [
{
code: "GOOGL",
price: 1700,
},
{
code: "AAPL",
price: 130,
},
{
code: "MSFT",
price: 219,
},
{
code: "TSLA",
price: 880,
},
{
code: "FB",
price: 267,
},
{
code: "AMZN",
price: 3182,
},
];
Now you need to filter the stock prices to include only those with prices lower than 1000
Here’s how you do it:
let filteredStocks = stocks.filter(function (currentElement) {
return currentElement.price < 1000;
});
The value of filteredStocks
will be as follows:
0: {code: "AAPL", price: 130}
1: {code: "MSFT", price: 219}
2: {code: "TSLA", price: 880}
3: {code: "FB", price: 267}
Finally, you can also write the callback function using ES6 arrow functions:
let filteredStocks = stocks.filter(
(currentElement) => currentElement.price < 1000
);
Manual filter implementation
Some old browsers like IE8 doesn’t support filter
method, so you need to write a manual filter by using a for
loop and array length
property as follows:
let filteredStocks = [];
for (let i = 0; i < stocks.length; i++) {
if (stocks[i].price < 1000) {
filteredStocks.push(stocks[i]);
}
}
console.log(filteredStocks);