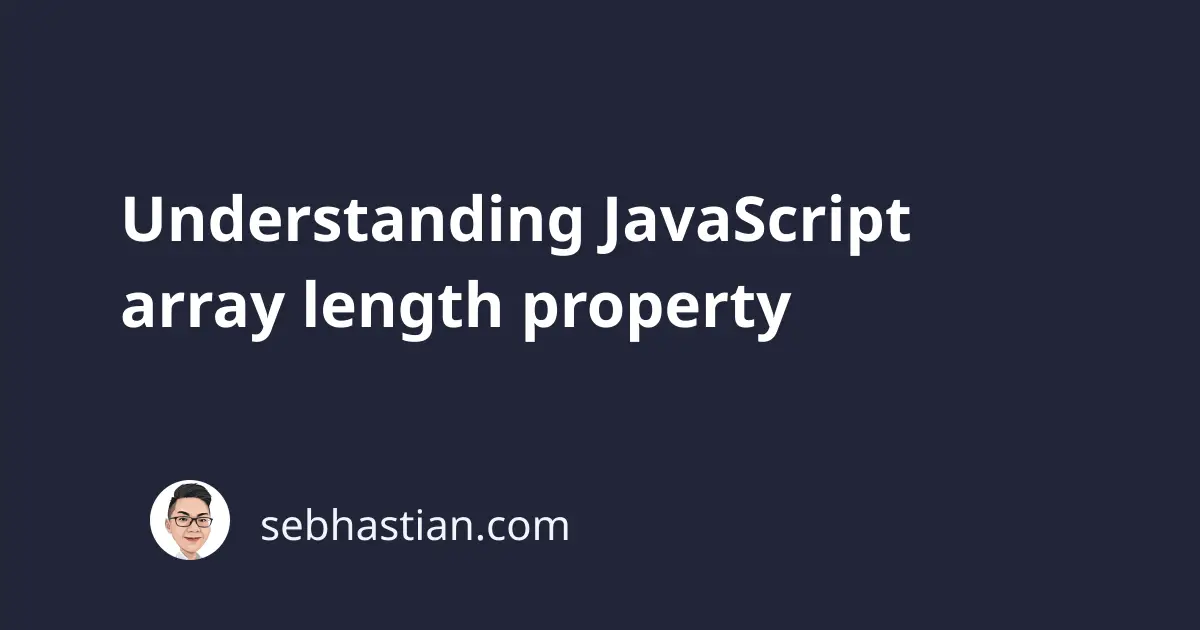
The JavaScript array length
property returns a number that represents how many elements (or values) are currently stored in a JavaScript array.
To use it, you just need to access the property like any other object properties:
let students = ["Joseph", "Marco", "Sarah", "Lisa"];
console.log(students.length);
// output is 4
This property is immediately available for all array objects that you declare in your script.
You don’t even need to assign your array object into a variable to use it:
["Joseph", "Marco", "Sarah", "Lisa"].length;
// 👆 returns 4 too
Iterate over an array with for loop and length
By utilizing the length
property, you can iterate over an array and perform operations on its elements. Here’s an example where every element of the array is doubled:
let myNumbers = [1, 2, 3];
for (let i = 0; i < myNumbers.length; i++) {
myNumbers[i] = myNumbers[i] * 2;
}
console.log(myNumbers);
// output is [2, 4, 6]
Since the array index starts from 0
, you can start the for
loop at 0
too by using let i = 0;
.
Set the length property manually
The array length
property can also be used to set the size of an array by assigning a new number to it.
Consider the following example:
// 👇 truncate an array
let students = ["Joseph", "Marco", "Sarah", "Lisa"];
students.length = 1;
console.log(students); // ['Joseph']
When you set the array length
property to be lower than the number of elements present, then the array will be truncated.
But you can’t set length
to a negative number value. It will cause an error:
let students = ["Joseph", "Marco", "Sarah", "Lisa"];
students.length = -4;
// Error: Invalid array length
You can also extend the length
of the array by assigning a higher number to the property.
The empty slot created by this assignment will return undefined
as its value:
let students = ["Joseph", "Marco"];
students.length = 4;
console.log(students);
// output is ["Joseph", "Marco", undefined, undefined]
If you push
a new element into the array, it won’t replace the undefined
elements. JavaScript will place the new element after those undefined elements instead:
let students = ["Joseph", "Marco"];
students.length = 4;
students.push("Martha");
console.log(students);
// ["Joseph", "Marco", undefined, undefined, "Martha"]
You need to replace the undefined
elements using the index:
let students = ["Joseph", "Marco"];
students.length = 4;
students[2] = "Martha";
console.log(students);
// ["Joseph", "Marco", "Martha", undefined]
Finally, when you loop over the array with forEach
method, the undefined
elements will be skipped:
let students = ["Joseph", "Marco"];
students.length = 4;
students.push("Martha");
students.forEach(element => console.log(element));
// "Joseph"
// "Marco"
// "Martha"
But when you do a manual loop with for
and length
, the undefined
elements will be included:
let students = ["Joseph", "Marco"];
students.length = 4;
students.push("Martha");
for (let i = 0; i < students.length; i++) {
console.log(students[i]);
}
// "Joseph"
// "Marco"
// undefined
// undefined
// "Martha"
This is one of the weird parts about JavaScript, but don’t worry too much because you will rarely need to assign the length
property manually like this.
Now you’ve learned how the JavaScript array length
property works. Nice work!