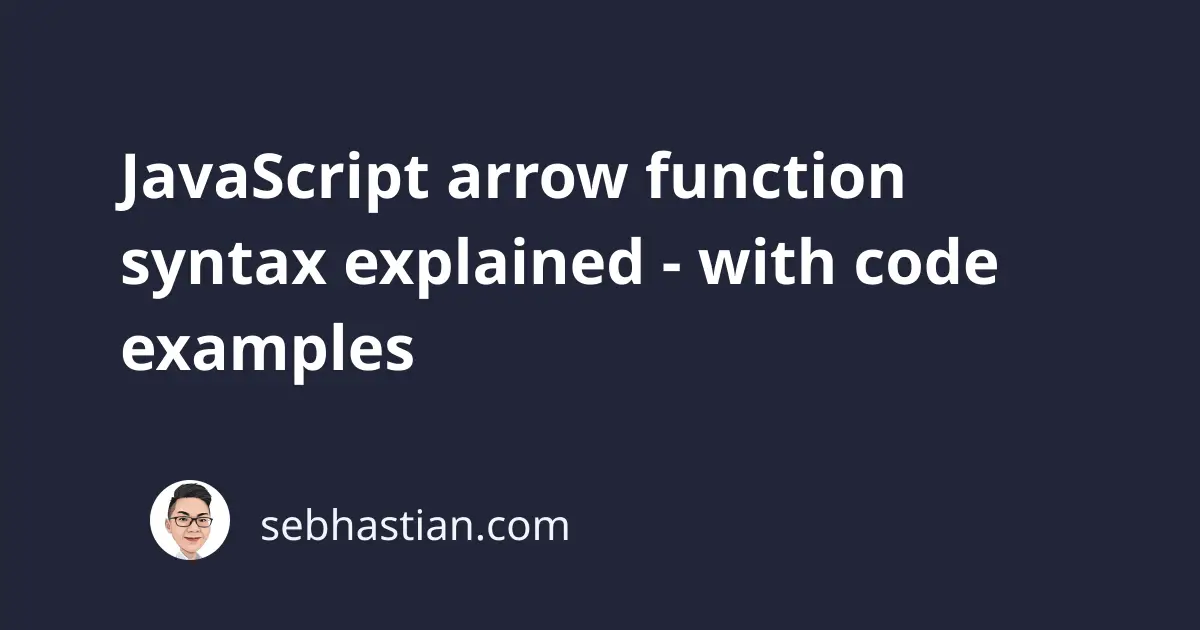
The JavaScript arrow function syntax allows you to write a JavaScript function with a shorter, more concise syntax.
When you need to create a function in JavaScript, the primary method is to use the function
keyword followed by the function name as shown below:
function greetings(name) {
console.log(`Hello, ${name}!`);
}
greetings("John"); // Hello, John!
The arrow function syntax allows you to create a function expression that produces the same result as the code above.
Here’s the greetings()
function using the arrow syntax:
const greetings = (name) => {
console.log(`Hello, ${name}!`);
};
greetings("John"); // Hello, John!
When you create a function using the arrow function syntax, you need to assign the expression to a variable so that the function has a name.
Basically, the arrow function syntax looks as follows:
const fun = (param1, param2, ...) => {
// function body
}
In the code above,
fun
is the variable that holds the function. You can call the function asfun()
later in your code(param1, param2, ...)
is the function parameters. You can define as many parameters as required by the function- Then you have the arrow
=>
to indicate the beginning of the function
The code above is equal to the following:
const fun = function(param1, param2, ...) {
// function body
}
The arrow function syntax doesn’t add any new ability to the JavaScript language.
Instead, it offers improvements to the way you write a function in JavaScript.
At first, it may seem weird as you are used to the function
keyword.
But as you start using the arrow syntax, you will see that it’s very convenient and easier to write.
Single and multiline arrow function
The arrow function provides you a way to write a single line function where the left side of the arrow =>
is returned to the right side.
When you use the function
keyword, you need to use the curly brackets {}
and the return
keyword as follows:
function plusTwo(num) {
return num + 2;
}
Using the arrow function, you can omit both the curly brackets and the return
keyword, creating a single line function as shown below:
const plusTwo = (num) => num + 2;
Without the curly brackets, JavaScript will evaluate the expression on the right side of the arrow syntax and return it to the caller.
The arrow function syntax also works for a function that doesn’t return
a value as shown below:
const greetings = () => console.log("Hello World!");
When using the arrow function syntax, the curly brackets are required only when you have a multiline function body:
const greetings = () => {
console.log("Hello World!");
console.log("How are you?");
};
Arrow function without round brackets
The round brackets ()
are used in JavaScript functions to indicate the parameters that the function can receive.
When you use the function
keyword, the round brackets are always required:
function plusThree(num) {
return num + 3;
}
On the other hand, the arrow function allows you to omit the round brackets when you have exactly one parameter for the function:
The following code example is a valid arrow function expression:
const plusThree = num => num + 3;
As you can see, you can remove the round and curly brackets as well as the return
keyword.
But you still need the round brackets for two conditions:
- When the function has no parameter
- When the function has more than one parameter
When you have no parameter, then you need round brackets before the arrow as shown below:
const greetings = () => console.log("Hello World!");
The same applies when you have more than one parameter.
The function below has two parameters: name
and age
.
const greetings = (name, age) => console.log("Hello World!");
The arrow syntax makes the round brackets optional when you have a single parameter function.
The this keyword context
When you write a function using the function
keyword, the this
keyword will refer to the object that called the function.
You can see this by calling a function assigned as an object’s property:
const myObj = {
name: "Jack",
myFun: function () {
console.log(this.name);
},
};
myObj.myFun(); // "Jack"
As you can see, the this
keyword in the myFun()
function refers to the object myObj
.
By contrast, when you use the arrow function syntax for the myFun
property, the this
keyword refers to the global object.
Because the name
property is not defined in the global object, JavaScript will return undefined
as shown below:
const myObj = {
name: "Jack",
myFun: () => console.log(this.name),
};
myObj.myFun(); // undefined
It’s important to remember that you can’t change the value of this
inside an arrow function.
If you need the this
keyword to refer to the object that called the function, then you need to use the old function
keyword.
Arrow function doesn’t have arguments binding
When using the function
keyword to define a function, you can access the arguments you pass to the function using the arguments
keyword like this:
const printArgs = function () {
console.log(arguments);
};
printArgs(1, 2, 3);
// [Arguments] { '0': 1, '1': 2, '2': 3 }
The arguments
keyword in the code above refers to the object that stores all the arguments you passed into the function.
By contrast, the arrow function doesn’t have the arguments
object and will throw an error when you try to access it:
const printArgs = () => console.log(arguments);
printArgs(1, 2, 3);
//Uncaught ReferenceError: arguments is not defined
You can use the JavaScript spread syntax to imitate the arguments
binding as follows:
const printArgs = (...arguments) => console.log(arguments);
printArgs(1, 2, 3);
// [1, 2, 3]
By using the spread syntax, the arguments you passed to the arrow function will be stored in an array.
Note that you need the round brackets even though you are passing just one argument to the function.
You can access the given arguments
with the array index notation as arguments[0]
, arguments[1]
, and so on.
Easy way to convert a normal function to an arrow function
You can follow the three easy steps below to convert a normal function to an arrow function:
- Replace the
function
keyword with the variable keywordlet
orconst
- Add
=
symbol after the function name and before the round brackets - Add
=>
symbol after the round brackets
The code below will help you to visualize the steps:
function plusTwo(num) {
return num + 2;
}
// step 1: replace function with let / const
const plusTwo(num) {
return num + 2;
}
// step 2: add = after the function name
const plusTwo = (num) {
return num + 2;
}
// step 3: add => after the round brackets
const plusTwo = (num) => {
return num + 2;
}
The three steps above are enough to convert any old JavaScript function syntax to the new arrow function syntax.
When you have a single line function, there’s a fourth optional step to remove the curly brackets and the return
keyword as follows:
// from this
const plusTwo = num => {
return num + 2;
};
// to this
const plusTwo = num => num + 2;
When you have exactly one parameter, you can also remove the round brackets:
// from this
const plusTwo = (num) => num + 2;
// to this
const plusTwo = num => num + 2;
But the last two steps are optional. Only the first three steps are required to convert any JavaScript function
and use the arrow function syntax.
You’ve just learned how the JavaScript arrow function syntax works, as well as the three easy steps to convert a regular function to an arrow function.
Thank you for reading! I hope this tutorial has been useful for you. 😉