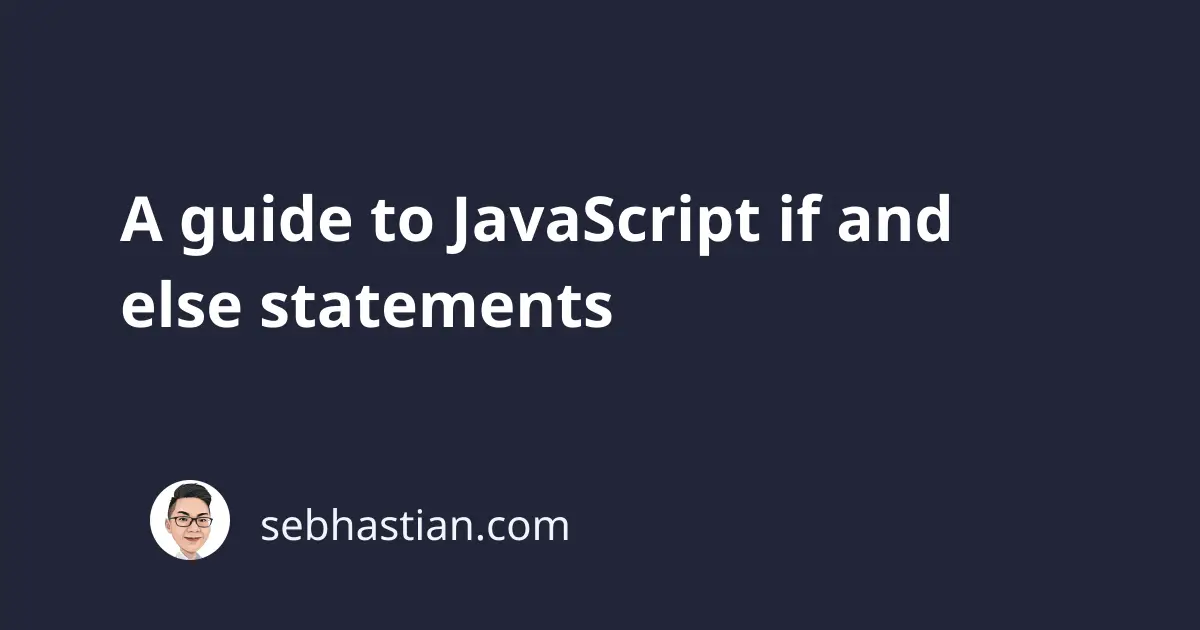
As you write your code, you will often find that you want to execute a block of your code according to some defined conditions.
For example, you may want to grant different responses for students depending on their test scores:
- If a student got an
A
score, you want to write “Congratulations! You did very well.” - If a student got a
B
score, you want to write “You did great! Keep improving your skills.”
You can write a program for the above requirements by using the if
statement:
let score = "A";
if (score === "A") {
console.log("Congratulations! You did very well.");
}
if (score === "B") {
console.log("You did great! Keep improving your skills.");
}
The syntax of the if
statement started with the keyword if
followed by parentheses (()
) for the condition, and then braces ({}
) for the block of code to be executed when the condition evaluates to true
:
if (condition) {
// The code to run when condition is true
// You can have multiple lines
// As long as they are inside the braces
}
Here is another example of an if
statement in action. Imagine you have a circus ticket price discount for children below the age of 10. You can write an if
statement like this:
let price = 20;
let age = 9;
if (age < 10) {
price = 15;
console.log(`The price of the ticket for children below 10 is ${price} USD`);
}
The if
statement can also be paired with the else
statement, which is useful to grant you even more control to the flow of your code.
The else statement
The else
statement is a conditional statement that allows you to execute a piece of code only when the if
statement before it returns false
:
if (condition) {
// Run this code when condition is true
} else {
// Run this code when condition is false
}
For example, you can further improve the circus ticket price calculator above by adding an else
statement that prints the regular ticker price:
let price = 20;
let age = 12;
if (age < 10) {
price = 15;
console.log(`The price of the ticket for children below 10 is ${price} USD`);
} else {
console.log(`The price of the ticket is ${price} USD`);
}
You can write if (age >= 10)
to replace the else
statement above, but since you only have two conditions, it’s better to use else
.
Using the else if statement
Finally, you can also use the else if()
statement to write another test condition to your code.
For example, let’s say you have another ticket discount for children below 15. You can use the else if
statement to perform a second check before the else
statement:
let price = 20;
let age = 12;
if (age < 10) {
price = 15;
console.log(`The ticket price for children below 10 is ${price} USD`);
}
else if (age < 15) {
price = 17;
console.log(`The ticket price for children below 15 is ${price} USD`);
}
else {
console.log(`The price of the ticket is ${price} USD`);
}
You can write the else
and else if
statement right after the closing brace }
or you can write them on the new line. JavaScript will treat them as the same.
Nested if statements
What’s more, you can even write an if
statement inside another if
statement as follows:
let price = 20;
let age = 8;
if(age < 10){
if (price > 15){
price = 15;
console.log(`Ticket price is now ${price} USD`);
}
}
But just because you can doesn’t mean you should. Multiple nested if
statements will increase the complexity level of your code and may even make it hard to read. When you need to control the flow of your code with if
and else
statements, take a moment to think through the code and make it as simple to read as possible.
When you require to write multiple if
s, consider using the switch
statement instead.
)