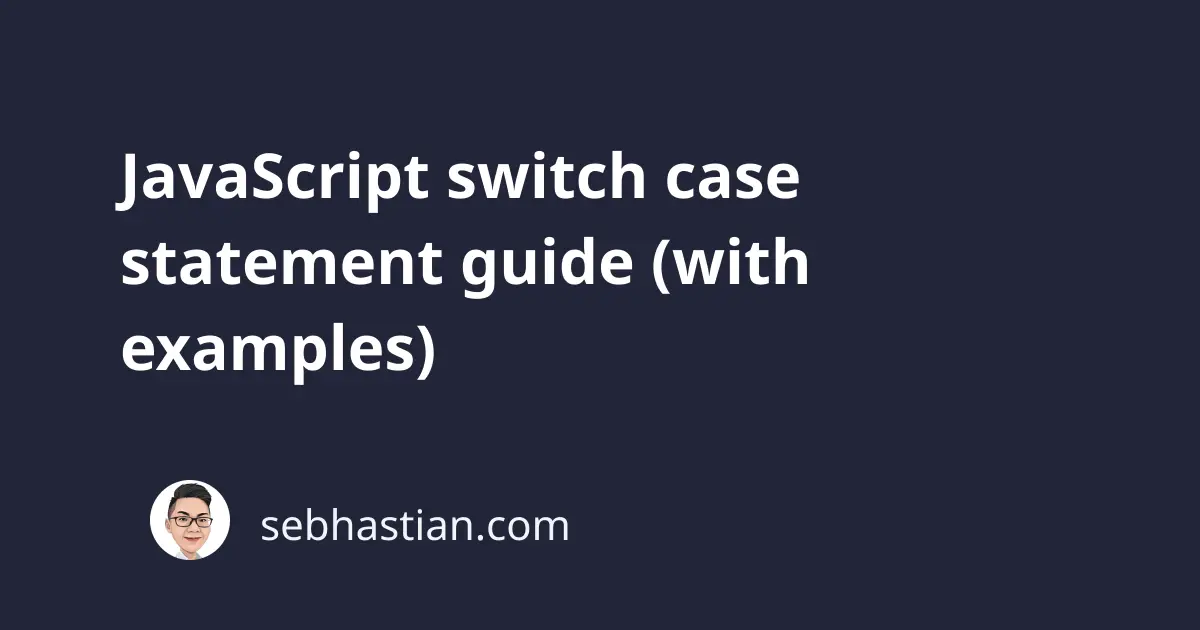
The switch
statement is a part of core JavaScript syntax that allows you to control the execution flow of your code.
It’s often thought of as an alternative to the if..else
statement that gives you a more readable code, especially when you have many different conditions to assess.
Here’s an example of a working switch
statement. I will explain the code below:
let age = 15;
switch (age) {
case 10:
console.log("Age is 10");
break;
case 20:
console.log("Age is 20");
break;
default:
console.log("Age is neither 10 or 20");
}
First, you need to pass an expression to be evaluated by the switch
statement into the parentheses. In the example above, the age
variable is passed as an argument for evaluation.
Then, you need to write the case
values that the switch
statement will try to match with your expression. The case
value is immediately followed by a colon (:
) to mark the start of the case block:
case "apple":
Keep in mind the data type of the case
value that you want to match with the expression. If you want to match a string
, then you need to put a string
. switch
statement won’t perform type coercion when you have a number
as the argument but put a string
for the case:
switch (1) {
case "1":
console.log("Hello World!");
break;
}
// The number expression doesn't match the string case value
// JavaScript won't log anything
The same also happens for boolean values. The number 1
won’t be coerced as true
and the number 0
won’t be coerced as false
:
switch (0) {
case true:
console.log("Hello True!");
break;
case false:
console.log("Bonjour False!");
break;
default:
console.log("No matching case");
}
Switch statement body
The switch
statement body is composed of three keywords:
case
keyword for starting a case blockbreak
keyword for stopping theswitch
statement from running the nextcase
default
keyword for running a piece of code when no matchingcase
is found.
When your expression found a matching case
, JavaScript will execute the code following the case
statement until it finds the break
keyword. If you omit the break
keyword, then the code execution will continue to the next block.
Take a look at the following example:
switch (0) {
case 1:
console.log("Value is one");
case 0:
console.log("Value is zero");
default:
console.log("No matching case");
}
When you execute the code above, JavaScript will print the following log:
> "Value is zero"
> "No matching case"
This is because without the break
keyword, switch
will continue to evaluate the expression against the remaining cases even when a matching case is already found.
Since the case evaluation will compare your expression value with the case value, your evaluation may never match more than one case, so the break
is commonly used to prevent the default
block and checking more cases than required.
Finally, you can also put expressions as case
values:
switch (20) {
case 10 + 10:
console.log("value is twenty");
break;
}
But you need to keep in mind that the value for a case
block must exactly match the switch
argument. One of the most common mistakes when using the switch
statement is that people think case
value gets evaluated as true
or false
.
The following case
blocks won’t work in switch
statements:
let age = 5;
switch (age) {
case age < 10:
console.log("Value is less than ten");
break;
case age < 20:
console.log("Value is less than twenty");
break;
default:
console.log("Value is twenty or more");
}
You need to remember the differences between the if
and case
evaluations:
if
block will be executed when the test condition evaluates totrue
case
block will be executed when the test condition exactly matches the givenswitch
argument
Switch statement use cases
The rule of thumb when you consider between if
and switch
is this:
You only use
switch
when the code is cumbersome to write usingif
For example, let’s say you want to write a weekday name based on the weekday number
Here’s how you can write it:
let weekdayNumber = 1;
if (weekdayNumber === 0) {
console.log("Sunday");
} else if (weekdayNumber === 1) {
console.log("Monday");
} else if (weekdayNumber === 2) {
console.log("Tuesday");
} else if (weekdayNumber === 3) {
console.log("Wednesday");
} else if (weekdayNumber === 4) {
console.log("Thursday");
} else if (weekdayNumber === 5) {
console.log("Friday");
} else if (weekdayNumber === 6) {
console.log("Saturday");
} else {
console.log("The weekday number is invalid");
}
I don’t know about you, but the code above sure looks cumbersome to me! Although there’s nothing wrong with the code above, you can make it more pretty with switch
:
let weekdayNumber = 1;
switch (weekdayNumber) {
case 0:
console.log("Sunday");
break;
case 1:
console.log("Monday");
break;
case 2:
console.log("Tuesday");
break;
case 3:
console.log("Wednesday");
break;
case 4:
console.log("Thursday");
break;
case 5:
console.log("Friday");
break;
case 6:
console.log("Saturday");
break;
default:
console.log("The weekday number is invalid");
}
When you have lots of condition to evaluate for the same block, you’d probably combine multiple if
conditions using the logical operator AND (&&
) or OR(||
):
let myBrowser = "Chrome";
if (myBrowser === "Edge" || myBrowser === "Firefox") {
console.log("Stop using other browsers. Download Chrome today.");
}
if (myBrowser === "Safari") {
console.log("Safari is good, but Chrome is better.");
}
You can replace the code above using the switch statement. The key is you need to stack multiple cases
as one just like this:
let myBrowser = "Chrome";
switch (myBrowser) {
case "Edge":
case "Firefox":
console.log("Stop using other browsers. Download Chrome today.");
break;
case "Safari":
console.log("Safari is good, but Chrome is better.");
break;
}
Unfortunately, switch
can’t replace multiple if
conditions that use &&
operator because of the way the case
evaluation works. You need to use if
statements for such “cases”.