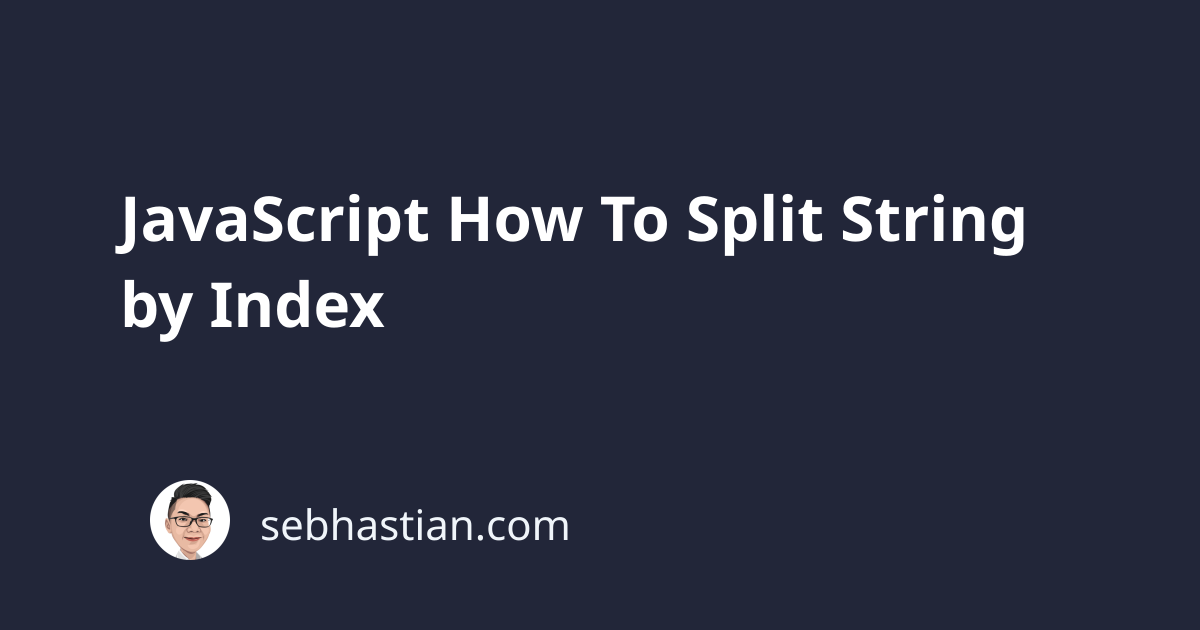
Hey friends, back to another article with me, Nathan. The other day I have a task to split a string by index value using JavaScript.
After a bit of research, I found that the easiest way to split a string by index is to use the substring()
or slice()
method, depending on the given string.
For example, suppose you need to split the text ‘HelloJohn’ into ‘Hello’ and ‘John’.
The index of the letter ‘J’ in the example is 5
, so you can use the substring()
method as follows:
let myString = 'HelloJohn';
// determine the index to split the string
let splitIndex = 5;
// split the string
let str1 = myString.substring(0, splitIndex);
let str2 = myString.substring(splitIndex);
// check the result
console.log(str1); // Hello
console.log(str2); // John
And that’s how you split a string by using index value. You can also replace the substring()
method with the slice()
method as follows:
let myString = 'HelloJohn';
// determine the index to split the string
let splitIndex = 5;
// split the string using slice
let str1 = myString.slice(0, splitIndex);
let str2 = myString.slice(splitIndex);
// check the result
console.log(str1); // Hello
console.log(str2); // John
Here, you can see there’s no difference between using the substring()
and slice()
methods.
If you want to join the string back, you can concatenate the returned values as shown below:
let myString = 'HelloJohn';
// determine the index to split the string
let splitIndex = 5;
// split the string using slice
let str1 = myString.slice(0, splitIndex);
let str2 = myString.slice(splitIndex);
// concatenate and modify the returned strings
let newString = str1 + ", Dear " + str2;
console.log(newString); // Hello, Dear John
You can also store the returned strings as an array like this:
let myString = 'HelloJohn';
// determine the index to split the string
let splitIndex = 5;
// split the string and store as an array
let arrayString = [myString.slice(0, splitIndex), myString.slice(splitIndex)];
console.log(arrayString); // [ 'Hello', 'John' ]
It’s really up to you what to do with the returned strings.
Split a string by multiple indices
Sometimes, you need to split a single string into many strings using multiple indices.
To do so, you need to create a custom function that accepts a string and an array of indices, then call the map()
method on each element inside the indices array.
In each iteration of the map()
method, we slice the string from the start index (the current element in the indices
array) until the stop index (the next element in the indices
array)
See the splitAt()
function below:
function splitAt(str, ...indices) {
return [0, ...indices].map((startIndex, index, arr) => {
return str.slice(startIndex, arr[index + 1]);
});
}
let splitString = splitAt('abcdefghij', 2, 4, 6);
console.log(splitString); // [ 'ab', 'cd', 'ef', 'ghij' ]
Here, you can see that the string ‘abcdefghij’ got split on indices 2, 4, and 6.
Anytime you need to split a string by multiple indices, you just need to call the splitAt()
function.
Conclusion
In this article, you’ve learned how to use the substring()
and slice()
methods to split a string by a specific index value.
You’ve also learned how to split a string using multiple indices, which can be done by using a custom function.
By the way, I have more articles related to JavaScript string manipulation here:
- JavaScript Replace All Numbers in a String
- How to Split a String Into an Array in JavaScript
- and JavaScript Format String With Variables and Expressions
I know these tutorials will be useful in your coding journey. Until next time! 👋