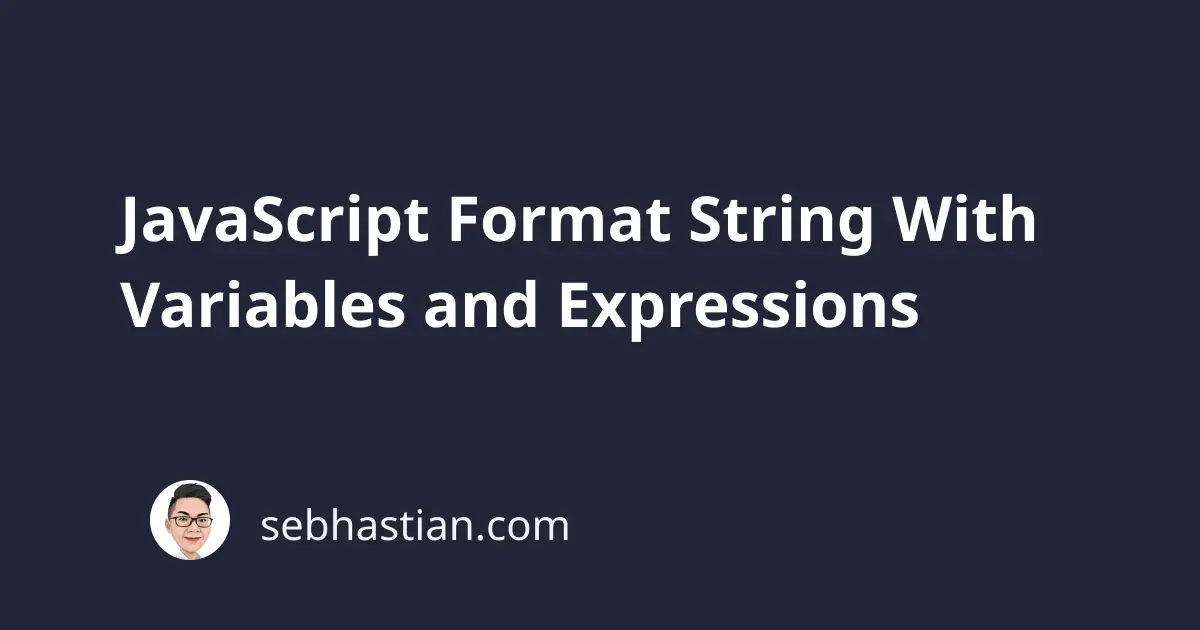
This tutorial will help you learn how to insert variables and expressions from your JavaScript code into a string.
JavaScript allows you to format a string with variables and expressions from your code in three ways:
- Using string concatenation with
+
symbol - Using template literals with backticks (
` `
) symbol - Using a custom string you define yourself
Let’s learn how to format a string using the three techniques above, starting with string concatenation.
Format JavaScript string with concatenation
When used in JavaScript strings, a plus +
symbol is treated as a concatenation operator that joins your variables and values as a string.
The code below shows how you can concatenate values together as a string:
let name = "Nathan";
let role = "Software Developer";
let aString = "Hello! My name is " + name + " and I'm a " + role;
console.log(aString);
// "Hello! My name is Nathan and I'm a Software Developer"
As you can see, the variable name
and role
are inserted into the string using the +
symbol.
But using the +
symbol can produce the wrong result if you’re using a more advanced format with expressions, such as number calculations and ternary conditionals.
For example, the following number expression produces the wrong output:
console.log("The sum of 5 + 2 is " + 5 + 2);
// "Sum of 5 + 2 is 52"
As you can see, adding the numbers 5 + 2
produces 52
instead of 7
.
To produce the correct output, you need to wrap the expression using round brackets as follows:
console.log("The sum of 5 + 2 is " + (5 + 2));
// "The sum of 5 + 2 is 7"
Next, using ternary operators also produce the wrong result as follows:
let married = false;
console.log("You are " + married == true ? "married" : "single");
// "single"
Like with the numbers, you need to wrap the ternary operation in a round bracket as follows:
console.log("You are " + (married == true ? "married" : "single"));
// "You are single"
And that’s how you can insert variables and values using the string concatenation technique.
Since 2015, a new syntax called template literals was added to the JavaScript language. This new syntax improves JavaScript’s ability to embed expressions into a string.
Format JavaScript string with template literals
The template literals are used for creating multi-line strings and inserting expressions into strings.
Template literals are also called template strings because they are used for creating strings with placeholders.
Template literals use the backtick symbol `
as the delimiters.
Here’s an example of a template literal in action:
let name = "Nathan";
let role = "Software Developer";
let aString = `Hello! My name is ${name} and I'm a ${role}`;
console.log(aString);
// "Hello! My name is Nathan and I'm a Software Developer"
Note how the aString
variable above starts with a backtick instead of a single or double quotes.
In a template string, you insert an expression into the string using the dollar sign and curly braces symbol (${}
).
You can also include numbers and ternary operations inside the ${}
symbol as shown below:
console.log(`The sum of 5 + 2 is ${5 + 2}`);
// "The sum of 5 + 2 is 7"
let married = false;
console.log(`You are ${married == true ? "married" : "single"}`);
// "You are single"
As you can see, it’s easier to format a string using template literals than string concatenations.
Since its release, the template literal has been the default technique used in formatting JavaScript strings.
Format JavaScript string with a custom function
In other programming languages like Python, the string has a format()
method that you can use to format a string with expressions.
The code below shows how the Python format()
method works:
name = "Nathan"
role = "Software Developer"
aString = "My name is {0}, I'm {1}".format(name, role)
print(aString)
# My name is Nathan, I'm Software Developer
In the example above, the {0}
and {1}
template is replaced with the expressions put in the format()
function call.
Following the Python implementation, you can add a format()
method to the JavaScript String
prototype as follows:
if (!String.prototype.format) {
String.prototype.format = function () {
var args = arguments;
return this.replace(/{(\d+)}/g, function (match, number) {
return typeof args[number] != "undefined" ? args[number] : match;
});
};
}
The format()
method above uses a regular expression to search and replace the template numbers like {0}
and {1}
with the arguments you pass into the method.
Once you’ve added the string method above, you can call it from any string you define in your code:
let name = "Nathan"
let role = "Software Developer"
let aString = "My name is {0}, I'm a {1}".format(name, role);
console.log(aString);
// My name is Nathan, I'm a Software Developer
In general, it’s not recommended to add a custom method to the prototype object unless you have a strong reason.
For most cases, using the template literal is enough to format a string with expressions and variables.
Now you’ve learned how to format a string with JavaScript in three different ways. Great job! 👍