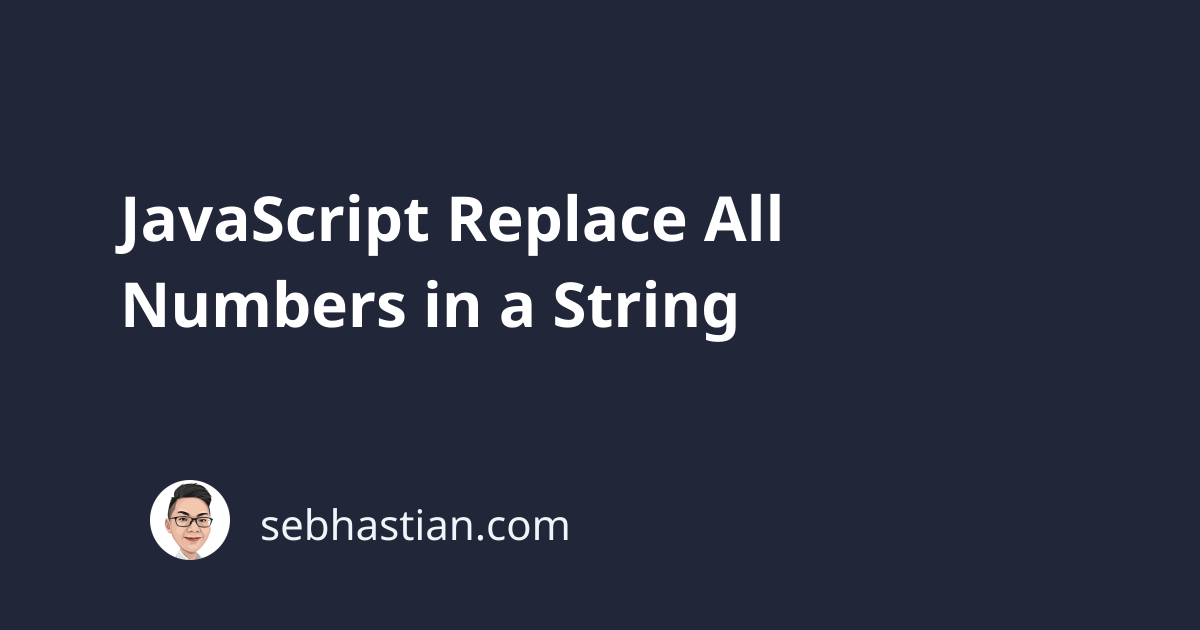
Hey friends! Today I’ll share a simple trick to replace all numbers from a string in JavaScript.
All you need to do is to use the String replace()
method and a regular expression to find all the numbers in your string, and then you replace them with a replacement string.
The following example code replaces the numbers with a dash:
const myStr = 'a123 b456 c789 d111';
// Replace each number in a string with a '-'
const newStr = myStr.replace(/[0-9]/g, '-');
console.log(newStr); // a--- b--- c--- d---
Here, you can see that the regular expression /[0-9]/g
will match all numbers in your string. The square brackets []
is also known as the character class. The character class [0-9]
will match digit characters from 0 to 9.
The g
option is also called global, and it will continue to match all digit characters in your string after the first match.
You can also replace the numbers with an empty string, effectively removing them from the string:
const myStr = 'a123 b456 c789 d111';
// Replace each number in a string with a ''
const newStr = myStr.replace(/[0-9]/g, '');
console.log(newStr); // a b c d
Here you see that the numbers are completely removed from the output string.
The replace()
method returns a new string where the matching pattern got replaced with the replacement you define as its arguments. The method takes the following parameters:
pattern
- The substring pattern to look for. Can be a string or a regular expression.replacement
- A string used to replace the substring match by the provided pattern.
You can also replace continuous matching numbers with a single character as follows:
const myStr = 'a123 b456 c789 d111';
// Replace each number in a string with a '-'
const newStr = myStr.replace(/[0-9]+/g, '-');
console.log(newStr); // a- b- c- d-
In the code above, a +
symbol is added after the character class, to repeat the matching pattern. This way, the continuous numbers are considered a single match, and so the number 123
got replaced by a single dash -
symbol.
You can also replace the character class [0-9]
with the \d
digit character:
const myStr = 'a123 b456 c789 d111';
// Replace each number in a string with a '-'
const newStr = myStr.replace(/\d/g, '-');
console.log(newStr); // a--- b--- c--- d---
The \d
symbol and the [0-9]
are the same, so just use which one you prefer.
And that’s how you replace all numbers in a string with JavaScript. I also have more articles related to string manipulation here:
- Replace Multiple Characters in a String Using JavaScript
- How to remove the last character from a JavaScript string
- and JavaScript Remove The First Character From a String
They might help you out. Happy coding and see you in other articles!