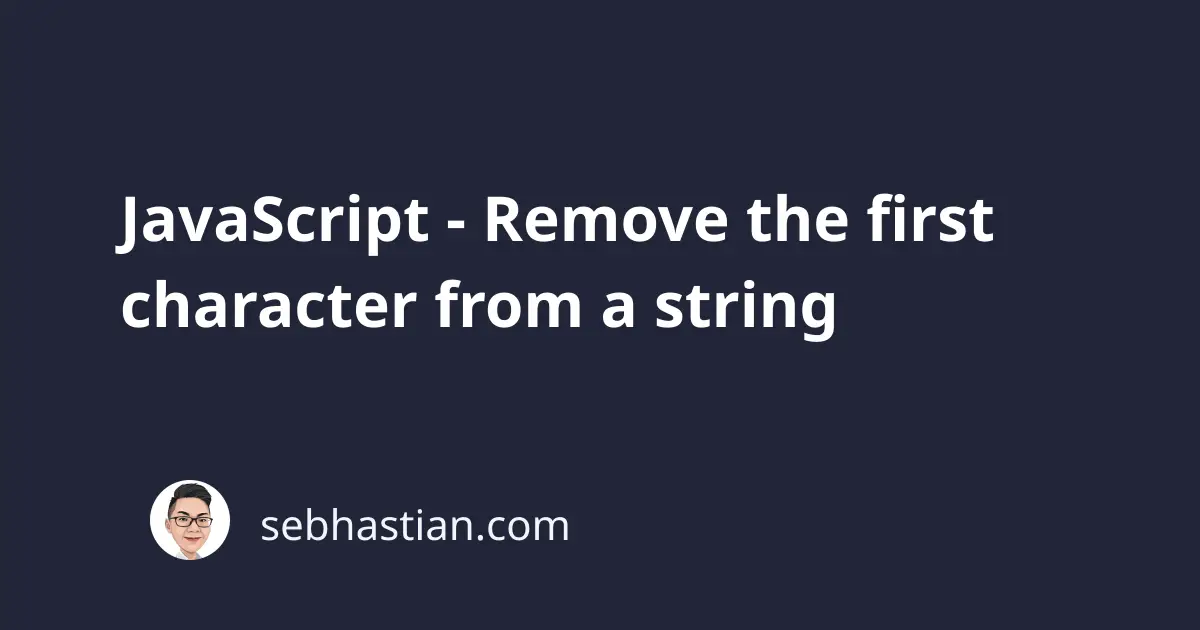
When you need to remove a character from a string, you can use the substring()
method.
The substring()
method extracts a part of a string and return it as a new string.
The method accepts two arguments: the start
and end
indexes.
String.substring(start, end);
The start
index is required by the method, while the end
index is optional.
When you omit the end
index, the method will not cut the string before the end.
Remove the first character from a string
When you need to remove the first character from a string, you can call .substring(1)
on your string.
See the code example below:
let str = "hello there";
let res = str.substring(1);
console.log(res);
// 👆 console log prints "ello there"
The res
variable above will exclude the first character h
from the str
variable.
The substring()
method returns a new string, so the original string will not be changed.
Remove only a certain first character
At times, you may want to remove the first character of a string only if it’s a certain character.
For example, let’s say you have a string representing your website’s URL:
let url = "/users";
In this case, suppose you only want to remove the forward slash (/
) character.
To do so, you need to check the first character using the charAt()
string method.
The charAt()
method extracts a character from a string at a specific index. Here’s an example of the charAt()
method in action:
let url = "/users";
console.log(url.charAt(0)); // prints "/"
console.log(url.charAt(4)); // prints "r"
By using the charAt()
method you can first check if the first character is something you want to remove.
Consider the code example below:
let url = "/users";
// 👇 remove first character if it's a "/"
if (url.charAt(0) === "/") {
url = url.substring(1);
}
console.log(url); // "users"
The charAt()
method allows you to check on the individual characters of a string through its index. You can also create a reusable function from this as follows:
let url = "/users";
let res = removeFirstChar(url);
console.log(res); // "users"
function removeFirstChar(str){
if(str.charAt(0) === "/"){
str = str.substring(1);
}
return str;
}
You can modify the removeFirstChar()
function above to suit your need.
And that’s how you remove the first character from a string in JavaScript.
Remove first N characters from a string
You can also the substring()
method to remove the first N characters from a string.
This is done by omitting the end
index, passing only the start
index.
For example, here’s how you remove the first 3 characters from a string:
let str = "Pokemon";
str.substring(3); // returns "emon"
And here’s how to remove the first 5 characters:
let str = "Pokemon";
str.substring(5); // returns "on"
And that will be all for this JavaScript tutorial. Thanks for reading!