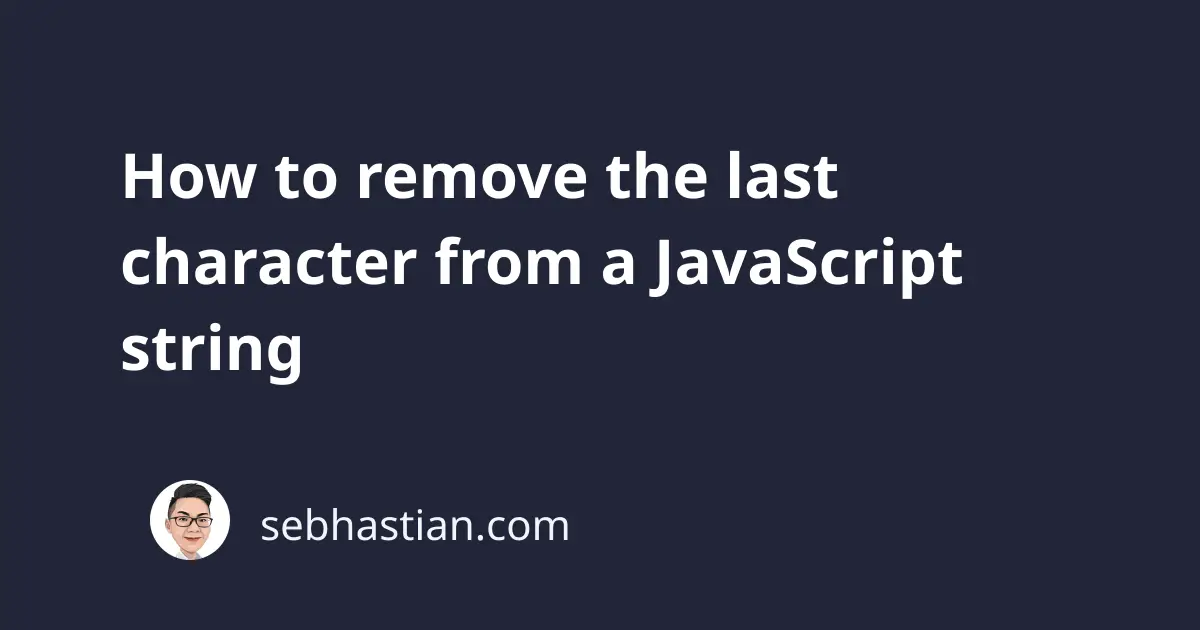
To remove the last character from a JavaScript string, you can use the built-in String.slice()
method which you can call from any string variable.
The slice
method returns a portion of the string from the indices that you specified as its arguments.
string.slice(startIndex, endIndex);
The method accepts two parameters as follows:
- The
startIndex
(optional) is the index number that specifies where to start the string extraction. - The
endIndex
(optional) is the index number that specifies where to end the string extraction. The index specified is excluded from the extraction.
Both parameters are optional, so if you pass nothing to the slice()
method, you will get a perfect copy of the original string:
let str = "Superhero";
let slicedStr = str.slice();
console.log(slicedStr); // Superhero
The index you passed into the endIndex
parameter is excluded from the returned string. The code below shows how the character r
at index 4
is excluded because it’s passed as the endIndex
parameter:
let str = "Superhero";
let slicedStr = str.slice(0, 4);
console.log(slicedStr); // Supe
Removing the last character using the slice()
method means you need to extract your string from the first index (at zero) to the last character.
The example below shows how to remove the character o
from the string Superhero
:
let str = "Superhero";
let slicedStr = str.slice(0, -1);
console.log(slicedStr); // Superher
You can either pass str.length - 1
or just -1
as the argument for the endIndex
parameter.
By using the slice()
method, you can remove the last character from any string variable that you have 😉