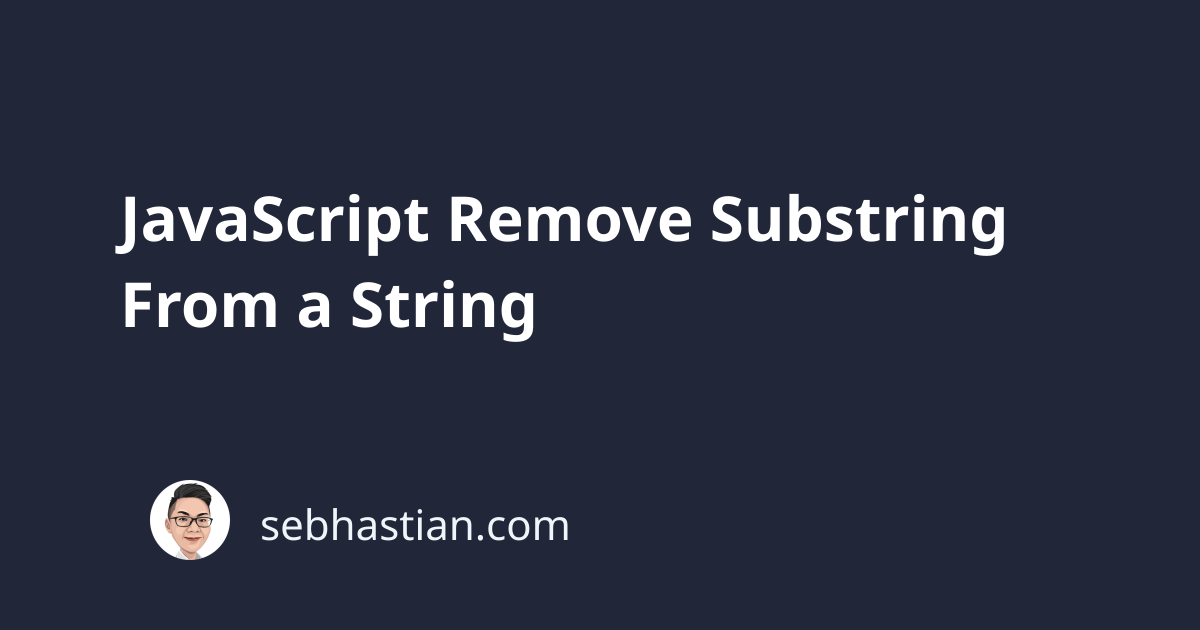
Hello friends! To remove a substring from a JavaScript string, you can use the replace()
method provided by the String prototype object.
The replace()
method is used to replace a single occurrence of a substring with a replacement string that you provide as its arguments. The method accepts 2 arguments as follows:
pattern
- The substring pattern to look for. Can be a string or a regular expression.replacement
- A string used to replace the substring match by the provided pattern.
You can remove a substring by passing an empty string as the replacement
value.
The following example shows how to remove the substring ‘abc’ from myString
below:
const myString = 'abcdef abcxys';
// Remove 'abc' substring
const newString = myString.replace('abc', '');
console.log(newString); // def abcxys
Here, you can see that the string ‘abc’ in front of the string is removed, but the second occurrence is ignored by the replace()
method. This is because replace()
only checks for a single occurrence of the substring from your string.
If you want to remove all occurrences of the substring, you need to use the replaceAll()
method instead. Here’s an example:
const myString = 'abcdef abcxys';
// Remove 'abc' substring
const newString = myString.replaceAll('abc', '');
console.log(newString); // def xys
In the above example, you can see that the ‘abc’ substrings are removed, leaving only ‘def’ and ‘xys’ on the string.
And that’s how you remove a substring from a string in JavaScript. I have more articles related to JavaScript string manipulation below:
- JavaScript Replace All Numbers in a String
- How to Split a String Into an Array in JavaScript
- and Javascript Check if String is Empty
These articles would come in handy to help you master JavaScript strings. Happy coding and I’ll see you in other articles! 👋