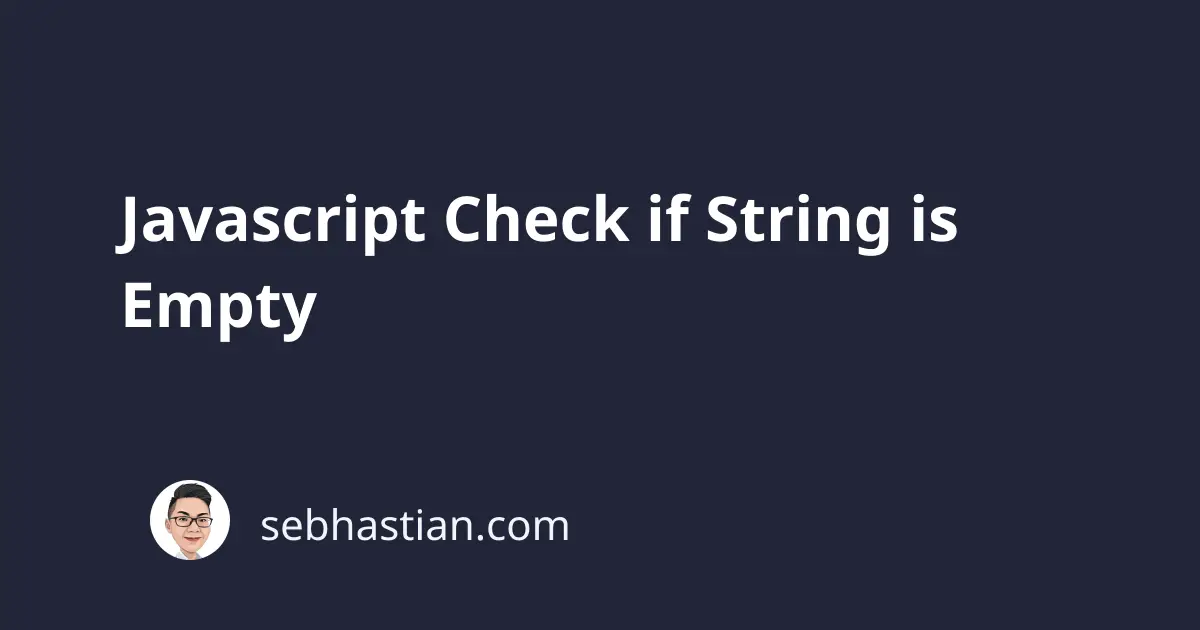
Sometimes you need to check if a string is empty or not in JavaScript. You might be retrieving data from an API endpoint, or receiving input from an HTML form.
This article will show you how to check if a string is empty, undefined or null with plenty of examples. You can decide which method is proper for your case later.
Empty VS Undefined VS Null String
Now before we start, I want to address the difference between an empty, undefined, and null string in JavaScript.
These three values are not the same in JavaScript, so you might have an empty string that is defined and not null.
Undefined is the default value assigned to a variable when you declare one without initializing it with a value. On the other hand, a null
is a special object representing nothing.
But an empty string is defined as a string variable containing no character. To tell these values apart, you need to check on the data type using the typeof
operator as follows:
let str1;
let str2 = null;
let str3 = "";
console.log(typeof str1); // "undefined"
console.log(typeof str2); // "object"
console.log(typeof str3); // "string"
The code above shows how JavaScript considers the three values to be different. Undefined is a special value, as is a null. For us humans, they look the same.
If you want to check for an empty string, you need to consider what kind of value you expect to receive.
How to Check for an Empty String in Javascript
To check for an empty string, you need to use the equality ===
operator and see if the variable value matches your definition of empty.
For example, here’s how to check if a string is undefined:
let str1;
console.log(str1 === undefined); // true
let str2 = null;
console.log(str2 === undefined); // false
Here, you can see that str1
is undefined, but str2
is not. To check for a null
value, specify it on the right side of the operand:
let str2 = null;
console.log(str2 === null); // true
There you go. The last one is to check for an empty string. I found the best way to get the job done is to compare the length
of the string. If it’s equal to 0, then it’s an empty string:
let str3 = '';
console.log(str3.length === 0); // true
But keep in mind that this comparison returns false
if you have white spaces in the string.
You need to use the trim()
method to remove empty spaces from the string:
let str3 = ' ';
console.log(str3.length === 0); // false
console.log(str3.trim().length === 0); // true
The trim()
method removes any white spaces at the beginning or the end of a string. Another way to check for an empty string is to compare the variable with an empty string as shown below:
let str3 = ' ';
console.log(str3 === ''); // false
console.log(str3.trim() === ''); // true
Don’t worry if you use double quotes to define your string. The validation above still works.
How to Check if a String is Empty or Null
Now that you know how to check for an empty string, you can combine the validation check using the logical OR ||
operator to check more than one specific value.
For example, you can check whether a string is empty or null with the following code:
let str1 = null;
if (str1 === null || str1.trim() === '') {
console.log('The string is empty!');
} else {
console.log('The string has a value!');
}
Please note that the order of the comparisons is important. If you try to check for an empty string first before checking for null, you’ll get an error that says Cannot read properties of null.
To avoid this error, check for null before checking for an empty string.
How to Check for Empty, Null, and Undefined Strings.
If you also want to check for undefined, you just need to add one more condition to the comparison:
let str1 = null;
if (str1 === undefined || str1 === null || str1.trim() === '') {
console.log('The string is empty!');
} else {
console.log('The string has a value!');
}
The code above will check if the string variable is undefined, null, or empty without issue.
Conclusion
Now you understand the differences between undefined, null, and empty strings and how to check if a string is any one of these values.
For you and me, the values all represent an empty string. But for JavaScript, these values have different meanings.
I hope this article is helpful. Happy coding and see you again! 👋