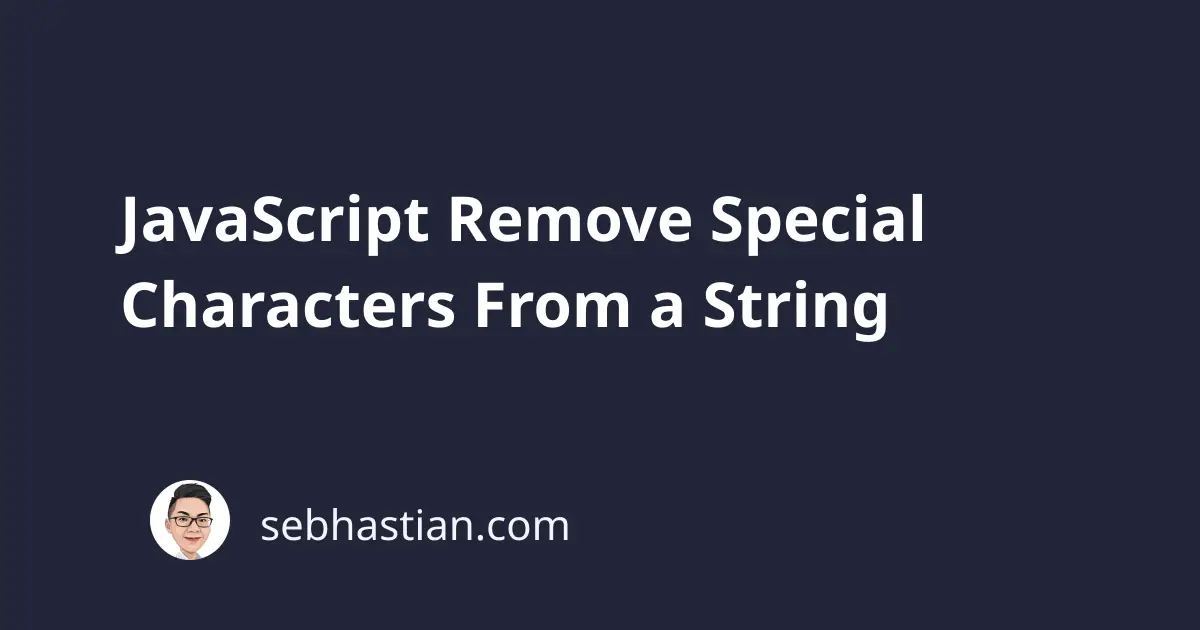
Hello friends! Back with me Nathan in a new article. Today I’m going to show you how to remove special characters from a string in JavaScript.
A special character is a character that’s not a letter or a number. To remove them from a string, you need to use the replace()
method that’s available for string values.
Add a regular expression as the part of string you want to replace, then pass an empty string as the replacement character as shown below:
const myString = 'Good !@#$%^Morning!?. 123';
const noSpecialChars = myString.replace(/[^a-zA-Z0-9 ]/g, '');
console.log(noSpecialChars); // 'Good Morning 123'
In the example above, the regular expression is filled with several symbols that have the following meanings:
- The square brackets
[]
part marks a character class, and we can specify the matching pattern inside - The caret
^
symbol inside the character class negates the character class, which means it matches any character that is not within the specified character set. - The characters
a-z
represents all lowercase alphabetic characters from ‘a’ to ‘z’. - The characters
A-Z
represents all uppercase alphabetic characters from ‘A’ to ‘Z’. - Next,
0-9
represents all decimal digits from ‘0’ to ‘9’. - The final character is a space.
Together, the regular expression will match any non alphabet, decimal, and whitespace characters. We remove the matching characters by replacing them with an empty string.
If you need help to modify the regular expression, check out the MDN cheat sheet for reference.
Specifying the Characters Manually
If you want to remove only specific special characters, you can specify the characters manually instead as shown below:
const myString = 'Good !@#$%^Morning!?. 123';
const noSpecialChars = myString.replace(/[&\/\\#,+()$~%.'":*?<>{}]/g, '');
console.log(noSpecialChars); // 'Good Morning 123'
In the above example, you can see that the special characters are manually written inside the square brackets []
. You can add and remove any special character as you need from the expression.
Using the \w Character Class
As an alternative, you can also use the \w
special character class in your regular expression. The \w
character class matches any alphabet, numeric, and the underscore characters.
Add the caret ^
symbol in front of this character class to remove any special characters:
const myString = 'Good _@#$%^Morning!?_ 123_';
const noSpecialChars = myString.replace(/[^\w]/g, '');
console.log(noSpecialChars); // 'Good_Morning_123_'
Notice that the underscores are not removed from the result string, while the white spaces are removed.
If you want to keep the white spaces, add it to the regular expression as follows:
const myString = 'Good _@#$%^Morning!?_ 123_';
const noSpecialChars = myString.replace(/[^\w ]/g, '');
console.log(noSpecialChars); // 'Good _Morning_ 123_'
If you want to remove the underscores, modify the regular expression with |_
after the square brackets as shown below:
const myString = 'Good _@#$%^Morning!?_ 123_';
const noSpecialChars = myString.replace(/[^\w ]|_/g, '');
console.log(noSpecialChars); // 'Good Morning 123'
Now the underscores are removed.
Remove the numbers from the string
If you want to remove the numbers from the string, you can use the first pattern above but remove the 0-9
part:
const myString = 'Good !@#$%^Morning123';
const noSpecialChars = myString.replace(/[^a-zA-Z ]/g, '');
console.log(noSpecialChars); // 'Good Morning'
You can see that the numeric characters are removed from the result string. Very nice!
Conclusion
To remove special characters from a JavaScript string, you need to use the replace()
method and define a regular expression to search for the special characters that need to be removed.
This article has shown you plenty of examples of how to write a regular expression that remove any special characters from a string.
And now you’ve learned how to removes special characters from a string in JavaScript. Congratulations!
I have some more articles related to JavaScript string below:
How to Split a String Into an Array in JavaScript
Javascript Check if String is Empty
Also, How to Remove a Part of JavaScript String From a Longer String
I know these articles will be useful in your journey as a JavaScript developer. See you again in other articles and have a nice day! 👋