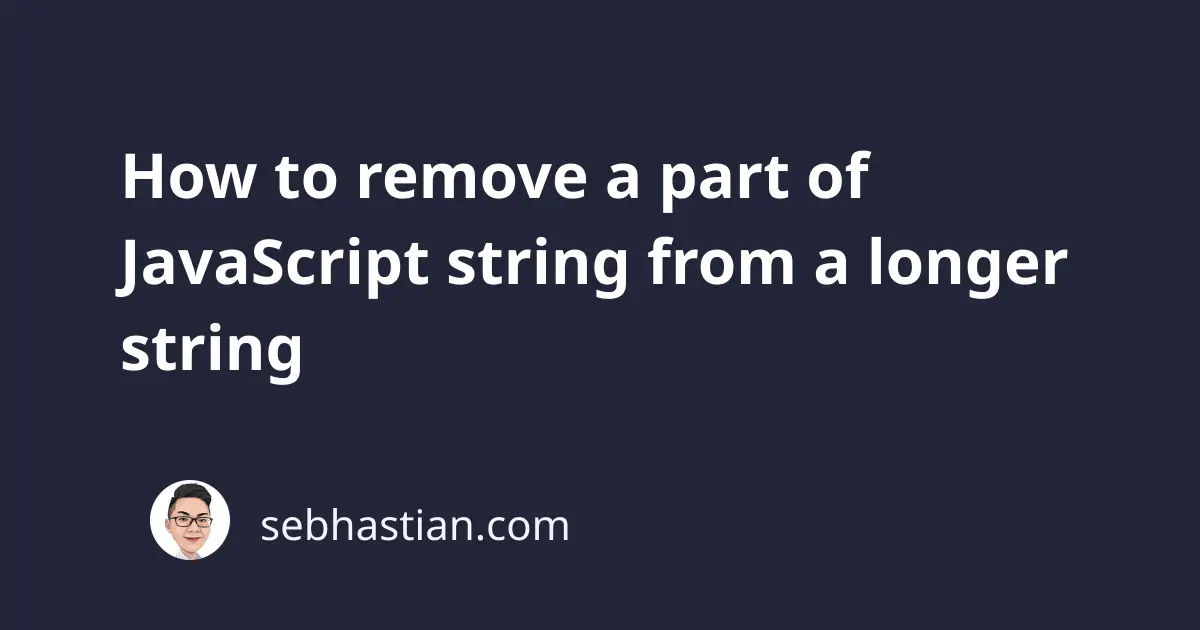
There are two methods in JavaScript that you can use to remove a part of a JavaScript string:
- The
String.replace()
method - The
String.substring()
method
This tutorial will help you learn both methods. Let’s start with String.replace()
method.
Remove a part of string using String.replace() method
The replace()
method is a built-in method of the String
type object that allows you to search your string for a specified string value and replace it with a new string value.
The syntax is as shown below:
String.replace(searchString, replaceString);
The method accepts two parameters:
- The
searchString
to find in the string. You can pass a regular string or a regular expression. - The
replaceString
to replace the found string.
You can use the replace()
method to remove a part of your string by passing an empty string (""
) as its second argument.
For example, the code below remove the word "Blue"
from "Blue Earth"
string:
let str = "Blue Earth";
let newStr = str.replace("Blue", "");
console.log(newStr); // " Earth"
Notice that the space in front of the "E"
letter is not removed from the newStr
variable. You need to add the space in the first argument when you want to remove it as well.
The replace()
method doesn’t modify the original string, so you need to store the string returned by the method in a different string.
The method only replaces the first occurence of the searchString
parameter:
let str = "Blue Earth Blue";
let newStr = str.replace("Blue", "");
console.log(newStr); // " Earth Blue"
If you want to remove all occurrences of the searchString
parameter, you need to use the replaceAll()
method.
The replaceAll()
method has the same syntax as the replace()
method:
let str = "Blue Earth Blue";
let newStr = str.replaceAll("Blue", "");
console.log(newStr); // " Earth "
// further remove the spaces
let nospaceStr = newStr.replaceAll(" ", "");
console.log(nospaceStr); // "Earth"
And that’s how you can remove a part of a string from string using the replace()
method.
Remove a part of string using String.substring() method
The substring()
method is a built-in method of the String
object that allows you to extract a specific string from a longer string.
The method uses the index of the characters in your string to determine where to start and end the extraction.
The substring()
method syntax is as shown below:
String.substring(startIndex, endIndex);
The method accepts two parameters:
- The
startIndex
to start the string extraction. - The
endIndex
to end the string extraction. The index you pass here is excluded from the end result.
When you omit the endIndex
parameter, then the string will be extracted to the end of the longer string.
A JavaScript string has number indices for each character in the string. It starts from left to right and begins from zero.
For example, here’s how to remove the string "Earth"
from "Blue Earth"
string:
let str = "Blue Earth";
let newStr = str.substring(0,4);
console.log(newStr); // "Blue"
And here’s how to remove the string "Blue"
from the same string:
let str = "Blue Earth";
let newStr = str.substring(5);
console.log(newStr); // "Earth"
Using the substring()
method, you need to specify the indices of the string that you want to keep instead of the one that you want to remove.
And those are the two methods you can use to remove a part of JavaScript string 😉