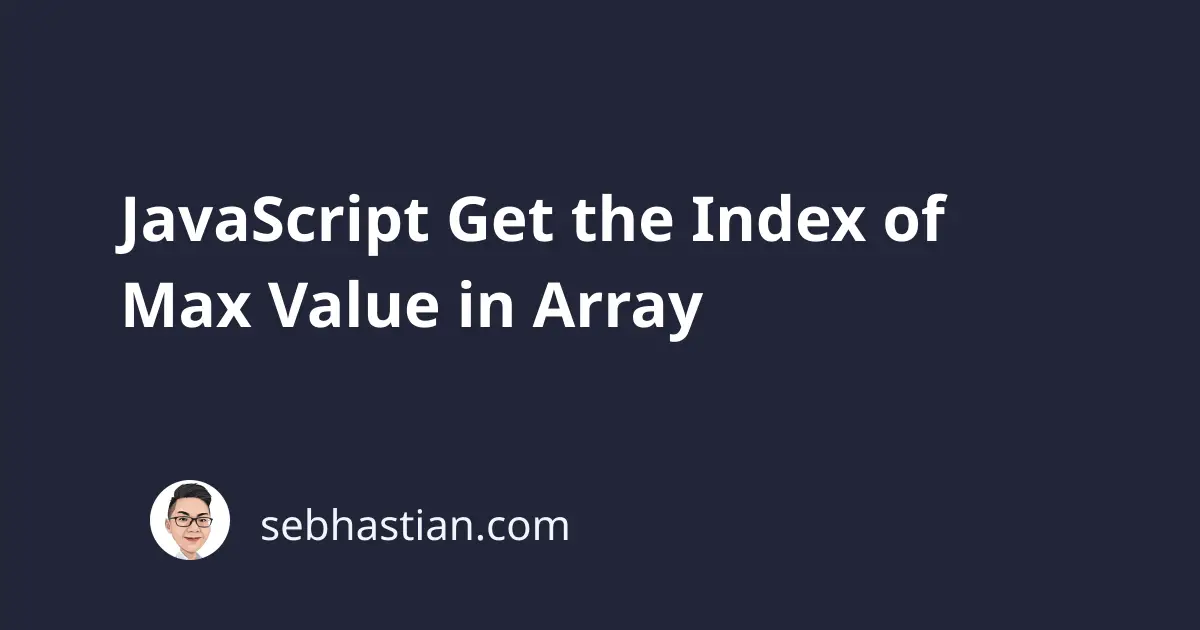
Hi friends! Today I want to show you how to get the index of the max value in an array using JavaScript.
When we work with an array of numbers, sometimes we need to find out what’s the maximum value, and then find its position in the array.
To do so, you need to use the Math.max()
method combined with the array.indexOf()
method.
Let me show you an easy example. Suppose you have an example array as shown below:
const myArray = [7, 35, 17, 64, 23, 91, 51, 22];
To find the greatest number in the array, we can call the Math.max()
method and pass the array as an argument to it.
When passing the array, use the spread operator ...
so that each number in the array gets passed as an argument:
const myArray = [7, 35, 17, 64, 23, 91, 51, 22];
const maxValue = Math.max(...myArray);
console.log(maxValue); // 91
Now that we have the max value of the array, the next step is to call the indexOf()
method from the array.
Pass the maxValue
variable as an argument to the indexOf()
method as shown below:
const myArray = [7, 35, 17, 64, 23, 91, 51, 22];
const maxValue = Math.max(...myArray);
const maxIndex = myArray.indexOf(maxValue);
console.log(maxIndex); // 5
Now we get that the index of the max value, which is 91, is 5. What you want to do with the data next is up to you.
Now you’ve learned how to get the max value of an array using Math.max()
and array.indexOf()
in JavaScript. Nice work!
I have some articles related to JavaScript array that would be useful to you:
JavaScript Array Not Includes a Certain Value
JavaScript Filter Array to Only Numbers
Merge Arrays Using JavaScript Array concat() Method
I’m sure they will be useful in your journey to master JavaScript. Until next time! 🙌