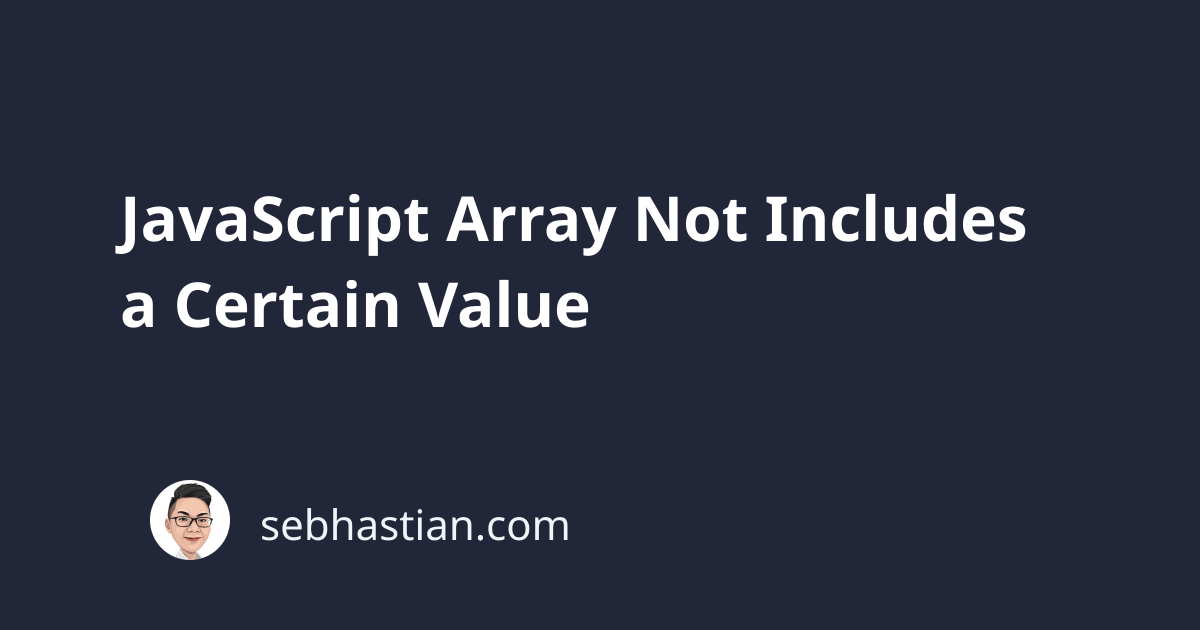
Hello there! Today, I will show you how to check if a JavaScript array doesn’t contain a specific value.
This is useful when you need to make sure that an array in your source code doesn’t contain a certain value.
To check that your array doesn’t contain a certain value, you need to use the logical NOT !
operator and negate the call to the includes()
method.
Inside the includes()
method, you pass the value you want to check. The following example shows how to check if an array doesn’t unclude the numerical value 1
:
const array1 = [1, 2, 3];
const array2 = [3, 4, 5];
console.log(!array1.includes(1)); // false
console.log(!array2.includes(1)); // true
When an array possess the element you specified in the includes()
method, the result is true
. Otherwise, you’ll get a false
.
And that’s how you check if an array doesn’t include a specific value. Let’s see how to check for multiple values next.
Check Array Not Include Multiple Values
To make sure that your array doesn’t include specific multiple values, you need to store the elements you want to check for in an array, then call the every()
method from the array with values to check.
The example below shows how to do it. I will explain what happens below:
const myArray = [1, 2, 3];
const valuesToCheck = [4, 5, 3];
const result = valuesToCheck.every(value => !myArray.includes(value));
console.log(result); // false
In this example, the specific values we want to check in an array are stored in an array called valuesToCheck
.
Next, we call the every()
method, which is used to test the elements of an array with a callback function.
In the callback function, we test if myArray
doesn’t include the values in the valuesToCheck
array.
Because myArray
contains 3
which is defined in valuesToCheck
array, the result is false
.
And that’s how you check if an array doesn’t include multiple values. Isn’t that simple?
Conclusion
This article has shown you how to check if an array doesn’t include a certain value. You’ve also learned how to check for multiple values.
I have some more articles related to JavaScript array here:
JavaScript Array Equality: A Smarter Way to Compare Two Arrays
Merge Arrays Using JavaScript Array concat() Method
Also, Javascript Convert Map Values to Array
I believe these articles will be useful in when you need to work with JavaScript arrays. Until next time! 👋