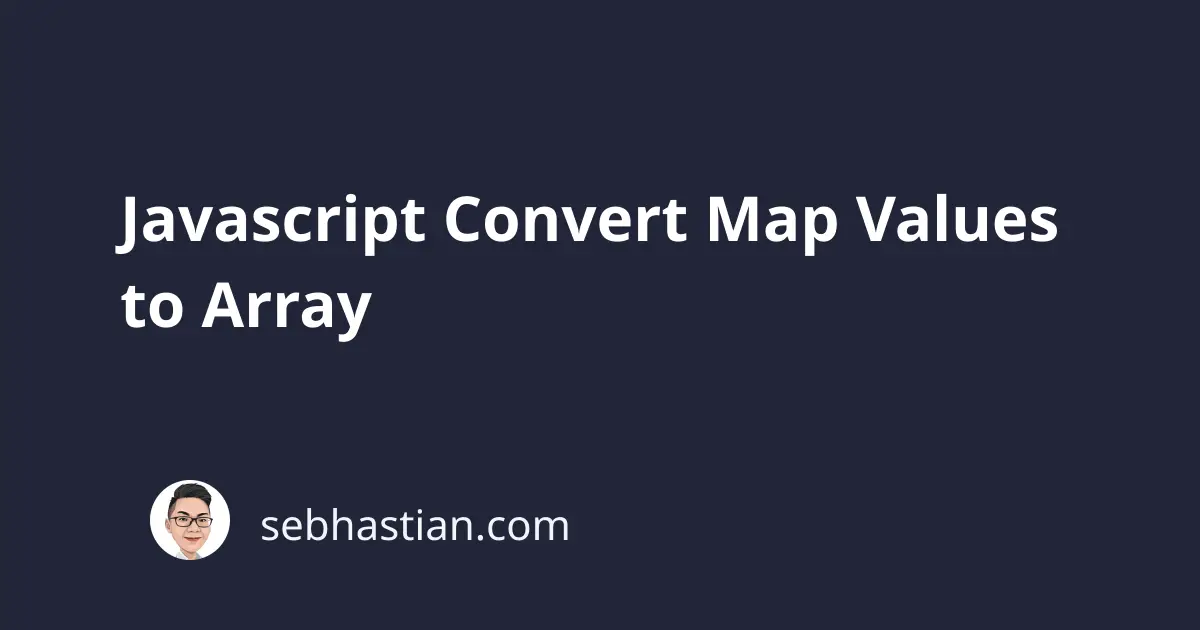
A Map object is a new type of object introduced in ES6 as an additional data structure you can use in your code.
The Map object is used to store a pair of key-value data in an ordered list, similar to a JavaScript object.
Sometimes you need to convert a Map object into an Array object. This article will show you how to:
- Convert Map values into an array
- Convert Map keys into an array
Ready to learn? Then let’s dive in.
Convert Map Values into an Array
To convert Map values into an Array, you need to use the Array.from()
and map.values()
method to create a new array.
The map.values()
method returns an iterator object containing all the values defined in a Map object. Since the Array.from()
method can accept an iterator object, you just pass it directly like this:
let myMap = new Map();
myMap.set('first_name', 'Nathan');
myMap.set('last_name', 'Sebhastian');
myMap.set('age', 30);
let myArray = Array.from(myMap.values());
console.log(myArray);
Here, the myArray
variable will have the values of myMap
as an array. The following output shows the conversion is successful:
[ 'Nathan', 'Sebhastian', 30 ]
Another way you can convert Map values into an Array is to use the spread operator to replace the Array.from()
method. Here’s an example:
let myMap = new Map();
myMap.set('first_name', 'Nathan');
myMap.set('last_name', 'Sebhastian');
myMap.set('age', 30);
let myArray = [...myMap.values()];
console.log(myArray); // [ 'Nathan', 'Sebhastian', 30 ]
In the code above, the spread operator expands the iterator object, and we use the array literal []
to capture the expanded values.
Now that you know how to convert Map values into an Array, the next step is to learn how to convert Map keys into an Array. It’s also very easy!
Convert Map Keys into an Array
To convert Map keys into an Array, you need to call the map.keys()
method, which creates an iterable object out of the keys stored in a Map object.
This iterable can be passed to the Array.from()
method to create an array, just like when converting Map values:
let myMap = new Map();
myMap.set('first_name', 'Nathan');
myMap.set('last_name', 'Sebhastian');
myMap.set('age', 30);
let myArray = Array.from(myMap.keys());
console.log(myArray);
// [ 'first_name', 'last_name', 'age' ]
Another way to convert Map keys into Array is to use the spread operator in place of the Array.from()
method:
let myMap = new Map();
myMap.set('first_name', 'Nathan');
myMap.set('last_name', 'Sebhastian');
myMap.set('age', 30);
let myArray = [...myMap.keys()];
console.log(myArray);
// [ 'first_name', 'last_name', 'age' ]
Now you can see that the keys are converted into an Array successfully.
Convert both Map keys and values into an Array
If you need to convert the key-value pair in your Map object into an Array, then you can call the Array.from()
method and pass the Map object as an argument to the method.
This will create a two-dimensional array, where each child array contains the key and the value of your Map object:
let myMap = new Map();
myMap.set('first_name', 'Nathan');
myMap.set('last_name', 'Sebhastian');
myMap.set('age', 30);
let myArray = Array.from(myMap);
console.log(myArray);
The code above will give the following output:
[
[ 'first_name', 'Nathan' ],
[ 'last_name', 'Sebhastian' ],
[ 'age', 30 ]
]
Here, you can see that the element at index 0 is the key, and the value is stored at index 1. There are three child arrays because we have three Map key-value pairs.
Conclusion
To convert Map values into an Array, you need to call the map.values()
method combined with Array.from()
method or the spread operator. The map.values()
method creates an iterable object that you can convert into an array using the Array.from()
method.
If you want to convert Map keys instead, use the map.keys()
method in place of map.values()
. If you want to convert a Map into an Array as key-value pairs, pass the Map object into the Array.from()
method.
I hope this tutorial is helpful. I have other articles related to JavaScript Array here:
- Parse CSV data into an array
- How to find the largest number in a JavaScript array
- Understanding JavaScript 2D array
They should help you better understand how to work with arrays in JavaScript. Happy coding!