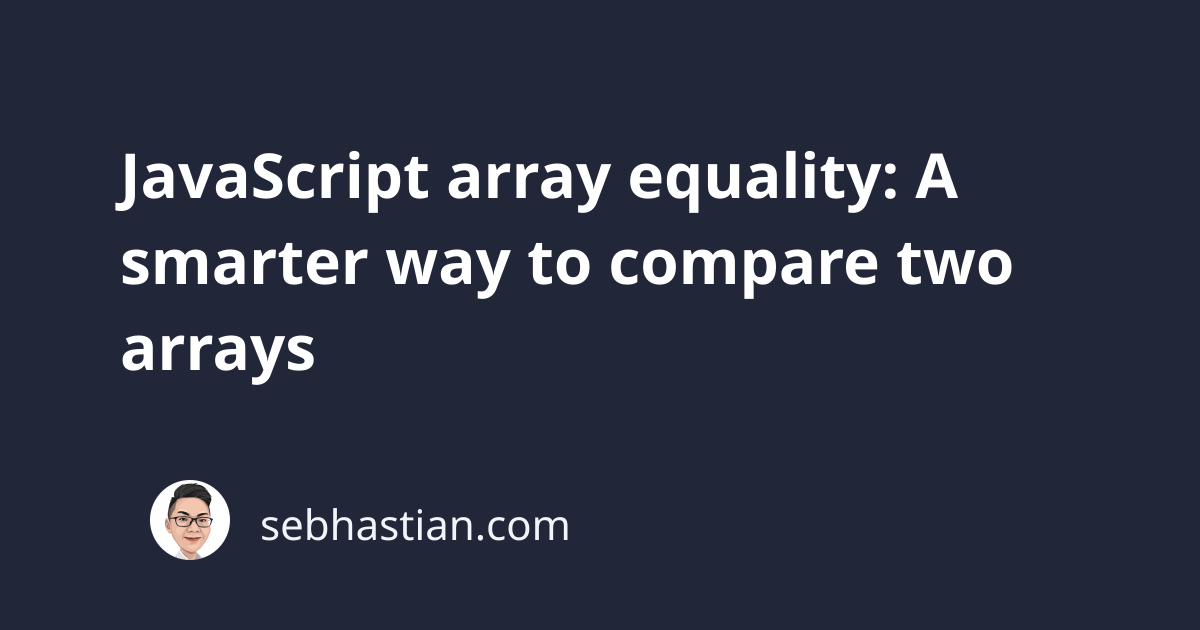
Since JavaScript array
type is actually a special object
type, comparing two arrays directly with ===
or ==
operator will always return false
:
let arrOne = [1, 2, 3];
let arrTwo = [1, 2, 3];
console.log(arrOne == arrTwo); // false
console.log(arrOne === arrTwo); // false
This is because JavaScript object
type compares the references for the variables instead of just the values. When you need to check for the equality of two arrays, you’ll need to write some code to work around the equality operators ==
and ===
result.
There are two ways you can check for array equality in JavaScript:
- Using
every()
andincludes()
method - Using a
for
loop and theindexOf()
method
This tutorial will show you how to do both. Let’s start with the first method
Checking array equality with every() and includes() method
To check if arrOne
is equal to arrTwo
, you can use the every()
method to loop over the first array and see if each element in the first array is included in the second array.
Take a look at the following example:
let arrOne = [1, 5, 6, 6];
let arrTwo = [1, 6, 5];
let result = arrOne.every(function (element) {
return arrTwo.includes(element);
});
console.log(result); // true
But since the code above only checks if elements of the first array is found in the second array, the result
will still be true
when either array has more elements than the other.
The following example shows how the equality check returns true even when arrOne
has more elements than arrTwo
:
let arrOne = [1, 5, 6, 6];
let arrTwo = [1, 6, 5];
let result = arrOne.every(function (element) {
return arrTwo.includes(element);
});
console.log(result); // true
To handle the length
differences, you need to check if the length
property of both arrays is equal as shown below:
let arrOne = [1, 5, 6, 6];
let arrTwo = [1, 6, 5];
let result =
arrOne.length === arrTwo.length &&
arrOne.every(function (element) {
return arrTwo.includes(element);
});
console.log(result); // false
And that’s how you can check for array equality using a combination of every()
and includes()
method. Next, you will learn how to do the same check manually with a for
loop and the indexOf()
method.
Checking array equality with for loop and indexOf() method
Another way to check for array equality is to replace the every()
method with the for
loop and use the indexOf()
method to see if the indexOf()
the first array elements are not -1
inside the second array.
First, you need to create the result
variable with false
as its initial value:
let arrOne = [1, 5, 6];
let arrTwo = [1, 6, 5];
let result = false;
Then, you need to create an if
block to check if the array lengths are equal. Only when the length properties are equal will you check on the array values:
let arrOne = [1, 5, 6];
let arrTwo = [1, 6, 5];
let result = false;
if (arrOne.length === arrTwo.length) {
for (let i = 0; i < arrOne.length; i++) {
// TODO: write the loop code
}
}
console.log(result);
Finally, you need to write the code inside the for
loop that will check for the existence of arrOne
elements inside arrTwo
using the indexOf()
method. The syntax will be arrTwo.indexOf(arrOne[i]) !== -1
.
The result of the indexOf()
method will return either true
or false
, and it will be assigned as the new value of result
variable. When the result
is false
, you must stop the iteration because there’s no point in checking the equality further.
Here’s the full code:
let arrOne = [1, 5, 6];
let arrTwo = [1, 6, 5];
let result = false;
if (arrOne.length === arrTwo.length) {
for (let i = 0; i < arrOne.length; i++) {
result = arrTwo.indexOf(arrOne[i]) !== -1;
if (result === false) {
break;
}
}
}
console.log(result); // true
And with that, you can check if your arrays are actually equal and having the same elements.