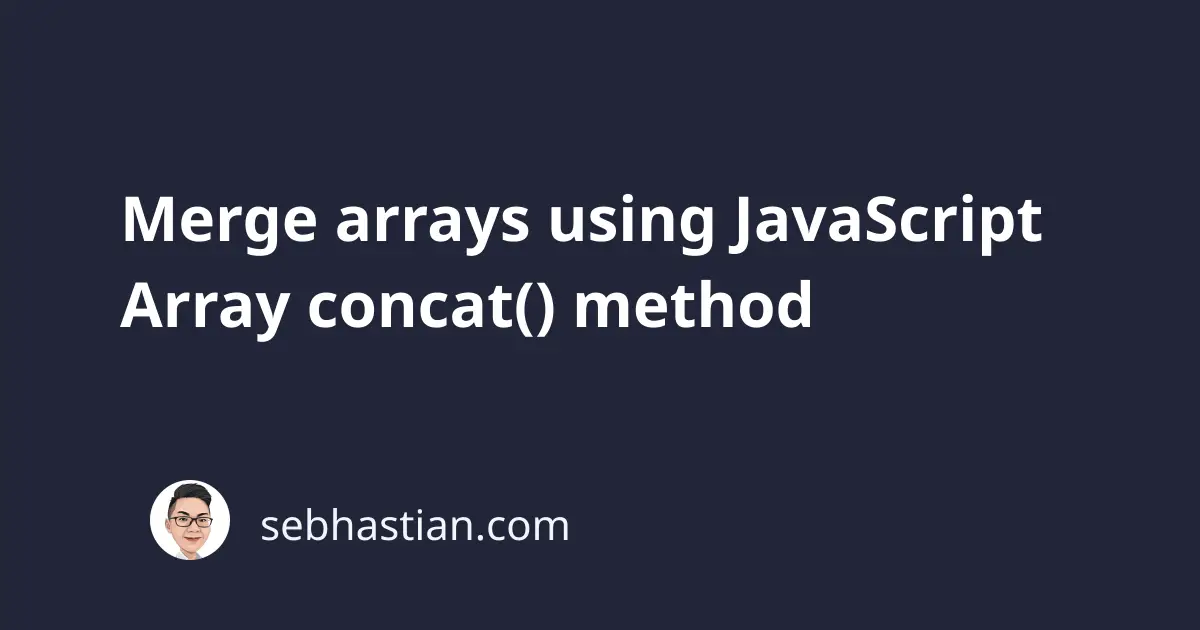
This tutorial will help you learn how to merge multiple arrays into a single array.
Merge arrays with the concat() method
The JavaScript concat()
method is a method of the Array
object that allows you to merge multiple arrays as a single array.
The method returns a new array instance, so it will not change the existing arrays won’t be modified.
The syntax of the concat()
method is as shown below:
[].concat(array1, array2, ..., arrayN);
You can merge as many arrays as you want using the concat()
method. Just pass the array into the method.
The code below shows how the method works:
const array1 = [1, 2, 3];
const array2 = ["a", "b", "c"];
const mergedArray = [].concat(array1, array2);
console.log(mergedArray);
// output:
// Array [1, 2, 3, "a", "b", "c"]
The order of the new array elements follow the order of the arrays provided as arguments to the concat()
method.
The concat()
method can also be used to merge non-array values into an array. This creates a new array that has both the array and non-array values.
The following code example shows how you can create new array and add string
values into it:
const myArray = [7, 8, 9];
const newArray = myArray.concat("z", "x", "y");
console.log(newArray);
// output:
// Array [7, 8, 9, "z", "x", "y"]
The concat()
method can be called from an existing array, or you can also use the array literal notation ([]
).
All values you passed as the method’s argument will be added to the array object where you call the method from.
Merge two arrays and remove duplicate items
Sometimes, you need to merge two arrays and remove duplicate items from the new array.
Unfortunately, the concat()
method will pass all original array values without removing duplicates as shown below:
const array1 = [1, 2, 3];
const array2 = [2, 3, 4];
const mergedArray = array1.concat(array2);
console.log(mergedArray);
// output:
// Array [1, 2, 3, 2, 3, 4]
There are two ways you can remove duplicates from the new merged array:
- Create a
Set
object from the array and then create an array from theSet
object - Use the
filter()
method to filter out duplicate elements
Let’s learn how to use these methods next.
Remove duplicate array elements using the Set object
The Set
object is used to store a collection of values that are unique.
You can create a Set
from an Array
to remove the duplicates like this:
const myArray = [1, 2, 3, 2, 3];
const mySet = new Set(myArray); // duplicates removed
console.log(Array.from(mySet));
// Array [1, 2, 3]
As you can see, creating a Set
from an Array
automatically removes the duplicate elements.
Then, you can create an array from the Set
using the Array.from()
method.
You can create a custom function to reuse the code above as follows:
function removeDupe(theArray) {
return Array.from(new Set(theArray));
}
Whenever you need to merge and remove duplicates, you can concat()
the arrays and then call the removeDupe()
function:
const array1 = [1, 2, 3];
const array2 = [2, 3, 4];
const mergedArray = array1.concat(array2);
console.log(mergedArray);
// output:
// Array [1, 2, 3, 2, 3, 4]
const uniqueArray = removeDupe(mergedArray);
console.log(uniqueArray);
// output:
// Array [1, 2, 3, 4]
Please note that the Set
object is only available from JavaScript ES6 version.
If you need to support older browsers, then you need to use the filter()
method instead.
Remove duplicate array elements using the filter method
The Array.filter()
method allows you to filter elements that don’t pass the test provided by the defined function.
You can use the filter()
method to remove duplicate elements in your array like this:
const myArray = [1, 2, 3, 2, 3];
const newArray = myArray.filter(function (element, index) {
return myArray.indexOf(element) === index;
});
console.log(newArray);
// output:
// Array [1, 2, 3]
The indexOf()
method returns the first index of the given element
that can be found in the array.
Any duplicate values will be filtered out because the index
position won’t be equal to the indexOf()
return value.
You can also create a reusable function using the filter()
method as follows:
function removeDupe(theArray) {
const newArray = theArray.filter(function (element, index) {
return theArray.indexOf(element) === index;
});
return newArray;
}
const myArray = [1, 2, 3, 2, 3];
const uniqueArray = removeDupe(myArray);
console.log(uniqueArray);
Anytime you need an array with unique values, just call the removeDupe()
function as defined above.
And that’s how you can merge and remove duplicates from an array in JavaScript. Nice work! 😉