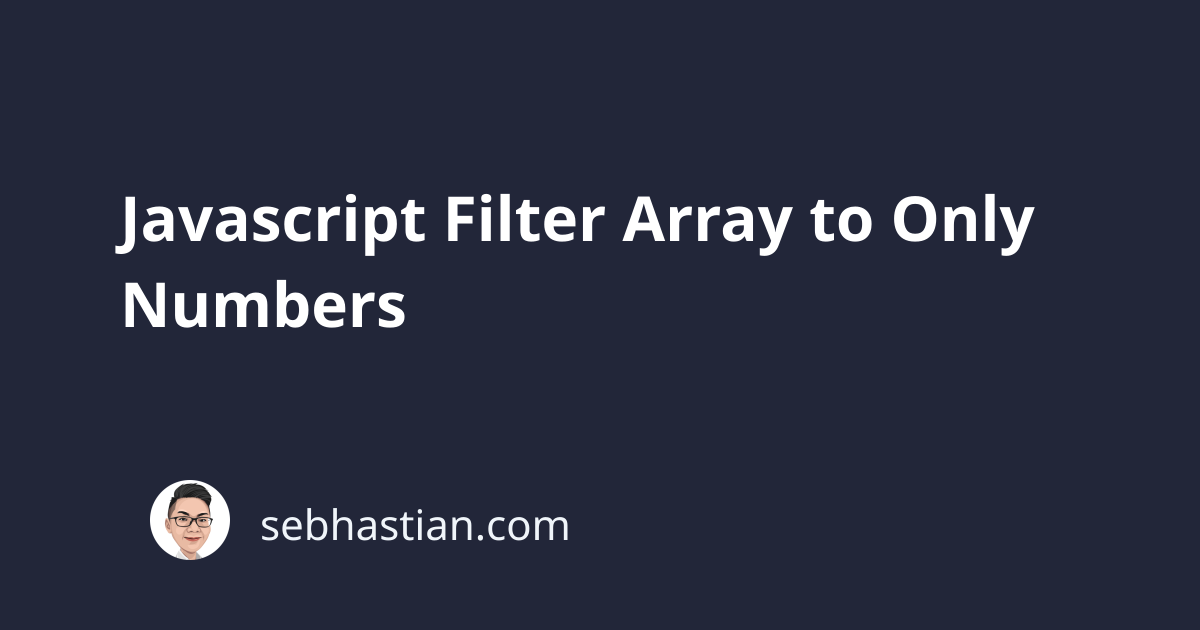
Hey friends! Today I’m going to show you how to filter an array in JavaScript so that only numbers will be included in the new array.
Sometimes, you have an array of mixed values and you need to filter that array to include only numbers. For example, you might have an array of strings and numbers as shown below:
let myArray = [1, 2, 'Andy', 8, 'John'];
To filter the array to only numbers, you can use the filter()
method and pass a callback function that checks the element’s type using the typeof
operator.
Check if the type of the element is a ’number’ as shown below:
let myArray = [1, 2, 'Andy', 8, 'John'];
let numbersArray = myArray.filter(element => typeof element === 'number');
console.log(numbersArray); // [ 1, 2, 8 ]
The filter()
method returns an array containing elements from the original array that returns true
when the callback function is executed.
The callback function will be called once for each element in the array. By using this solution, we can filter the array to only numbers.
Filter numbers in the form of strings
Other times, you might have an array that has numbers in the form of strings like this:
let myArray = ['1', 2, 'Andy', 8, 'John', 10, null];
In the above example, you can see that the number ‘1’ is defined as a string. If we use the typeof
solution, we’ll exclude the number from the returned array.
To include numbers in the form of strings in your new array, you need to pass the Number
constructor into the filter()
method as follows:
let myArray = ['1', 2, 'Andy', 8, 'John', 10, null];
let numbersArray = myArray.filter(Number);
console.log(numbersArray); // [ '1', 2, 8, 10 ]
In the above example, you can see that the number ‘1’ is included in the result.
This happens because when you pass the Number
constructor into the filter()
method, each element passed to the callback function will be passed into the Number
constructor.
The code above is the same as the one below:
// ---1
let numbersArray = myArray.filter(Number);
// ---2
let numbersArray = myArray.filter(element => Number(element));
The string element would return a NaN
value if it doesn’t have a number representation. A null
would return a 0
which is the equivalent of false
in JavaScript.
You can see the example results below:
console.log(Number('Andy')); // NaN
console.log(Number(null)); // 0
console.log(Number('1')); // 1
By using the Number()
constructor, you can get the numbers in the form of strings in your new array.
What if I want to convert the numbers into strings?
In that case, you need to call the map()
method before you call the filter()
method on the array.
The map()
method will execute the callback function you passed to it on each element and return the result.
You can pass the Number
constructor as the callback function to the map()
method to get the number representation of your original elements:
let myArray = ['1', '2', 'Andy', 8, 'John', 10, null];
let numbersArray = myArray.map(Number);
console.log(numbersArray); // [ 1, 2, NaN, 8, NaN, 10, 0 ]
numbersArray = numbersArray.filter(Number);
console.log(numbersArray); // [ 1, 2, 8, 10 ]
After the map()
method, call the filter()
method on the array as shown above, and you’ll get the result you want.
And that’s how you filter an array to include only numbers in JavaScript. I have more articles related to JavaScript array here:
- JavaScript Get Sum of Array of Numbers
- How to Shuffle Elements of an Array in Javascript
- also, Check if a Javascript Array Is Empty With Examples
I’m sure these articles will be useful in your journey as a web developer. Until next time, this is Nathan writing. Goodbye! 👋