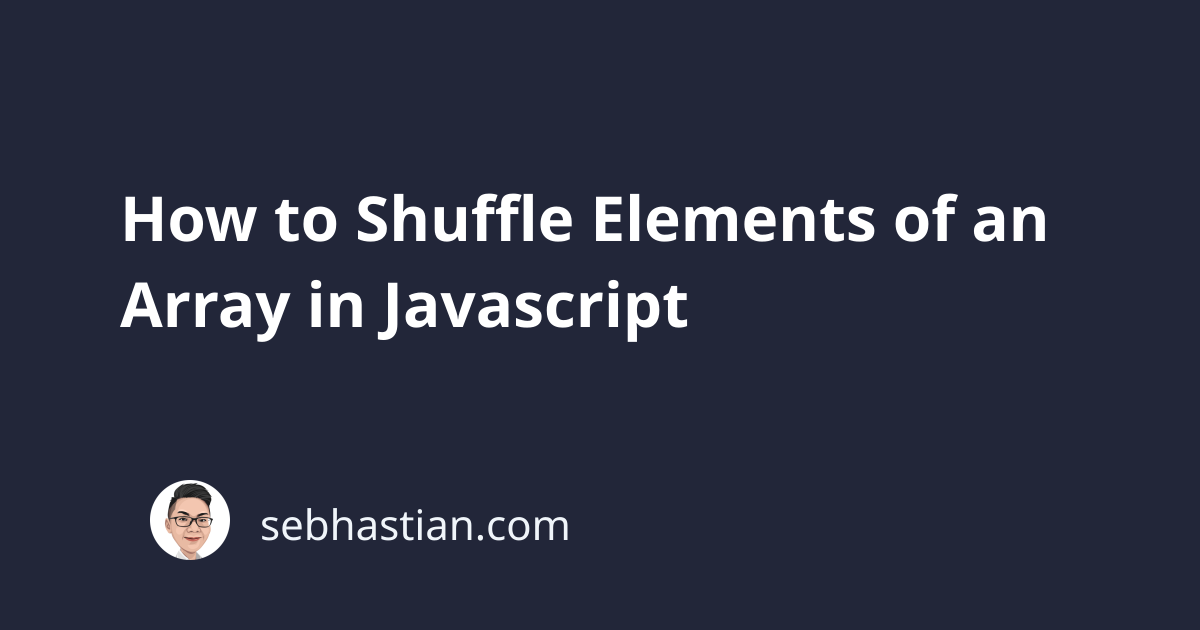
A JavaScript array elements can be shuffled by using the sort()
method.
Consider the code example below:
let numbers = [1, 2, 3, 4, 5, 6, 7, 8];
let shuffledNumbers = numbers.sort(function () {
return Math.random() - 0.5;
});
console.log(shuffledNumbers);
// [7, 8, 3, 1, 5, 4, 2, 6]
The JavaScript Array sort()
method is used to sort the elements of an array.
The method accepts a comparison function and performs a sort based on the value returned by that function.
The comparison function is executed on every element in your array. Usually, two elements of the array are passed as arguments to the function like this:
numbers.sort(function (a, b) {
return Math.random() - 0.5;
});
But since you’re not using the elements in the function body, you can omit the arguments.
The rule of the sort()
method is that when the comparison function returns > 0
then the element a
is put before b
.
When the function returns a value < 0
then a
is put after b
.
Consider the following examples:
// Example 1: a after b
let pair = [17, 99]; // a, b
console.log(pair.sort(() => -1));
// [99, 17] // b, a
// Example 2: a before b
let anotherPair = [27, 5]; // a, b
console.log(anotherPair.sort(() => 1));
// [27, 5] // a, b
The Math.random()
method returns a random number between 0
to 0.999
.
When you return Math.random() - 0.5
from the sort()
method, it means that the method will return a random number between -0.5
and 0.499
.
This makes the sort()
method perform the sort randomly, shuffling the elements in your array without any clear pattern.
And that’s how you can shuffle an array using JavaScript.
You can shorten the function into a one-liner using the arrow function syntax as follows:
let numbers = [1, 2, 3, 4, 5];
let shuffled = numbers.sort(() => Math.random() - 0.5);
console.log(shuffled);
// [1, 2, 5, 3, 4]
The sort()
method above randomly reorders the array every time you run the code.
You can also write a JavaScript function named shuffle()
using the sort()
method as follows:
function shuffle(array) {
return array.sort(() => Math.random() - 0.5);
}
let numbers = [1, 2, 3, 4, 5];
console.log(shuffle(numbers));
// [1, 2, 5, 3, 4]
let letters = ["a", "b", "c", "d", "e"];
console.log(shuffle(letters));
// ["c", "e", "b", "a", "d"]
The shuffle
function accepts an array
as its argument and returns a shuffled array from the given array
.
Please note that the sort()
method modifies the original array.
If you want to keep the original array, then you need to use the spread operator (...
) to create a shallow copy of the array.
You need to call the sort()
method on the array copy:
function shuffle(array) {
return [...array].sort(() => Math.random() - 0.5);
}
let numbers = [1, 2, 3, 4, 5];
console.log(shuffle(numbers));
// [1, 2, 5, 3, 4]
console.log(numbers);
// [1, 2, 3, 4, 5]
let letters = ["a", "b", "c", "d", "e"];
console.log(shuffle(letters));
// ["c", "e", "b", "a", "d"]
console.log(letters);
// ["a", "b", "c", "d", "e"]
You can learn more about the array sort()
method here:
Feel free to use the code in this tutorial for your JavaScript application. 😉