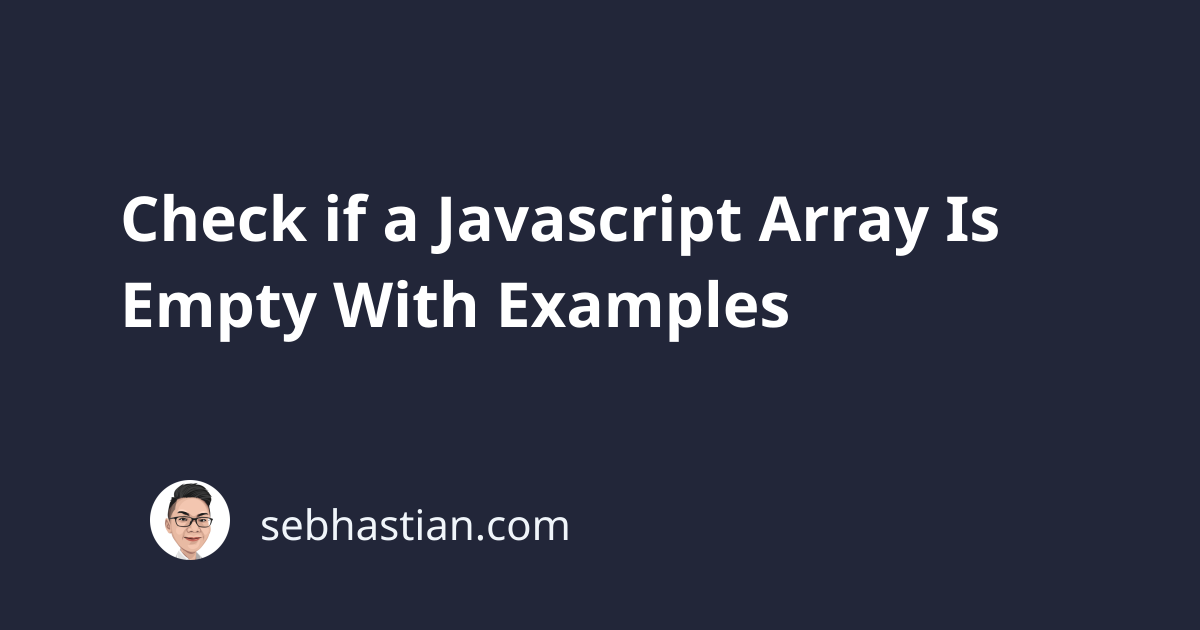
You can use the .length
array property to check whether a JavaScript array is empty or not. Your array will return any value other than zero as long as you have an element in your array.
Here’s an example:
let emptyArr = [];
console.log(emptyArr.length); // 0
let newArr = ["John", 7];
console.log(newArr.length); // 2
You can create an if..else
statement with the .length
property to execute a piece of code only when the array is not empty:
let newArr = [2, 2, 5];
if (newArr.length) {
console.log(`The array has ${newArr.length} elements!`);
} else {
console.log("The array is empty!");
}
You can also reverse the condition to run the if
statement block only when it’s empty with !newArr.length
or newArr === 0
. The exclamation mark (!
) is also known as the NOT operator in JavaScript, and it will reverse a true
to false
and false
to true
:
let emptyArr = [];
console.log(!emptyArr.length); // true
let newArr = ["John", 7];
console.log(!newArr.length); // false
Any positive number beside zero implicitly has true
boolean value. The following examples will produce the same results:
let newArr = [2, 2, 5];
if (!newArr.length) {
console.log("The array is empty!");
} else {
console.log(`The array has ${newArr.length} elements!`);
}
let newArr = [2, 2, 5];
if (newArr.length === 0) {
console.log("The array is empty!");
} else {
console.log(`The array has ${newArr.length} elements!`);
}
It’s just more intuitive in programming to check for positive values like newArr.length
rather than !newArr.length
, but it’s totally up to you.
Add an extra check with Array.isArray
A JavaScript string
also has the .length
property that returns how many characters the string has:
let newString = "hello";
console.log(newString.length); // 5
To guard your code from type mismatch between an array and a string, you can use the Array.isArray()
method first to check whether your variable is really an array type.
Although Array.isArray
seems an overkill. It’s actually useful to check if your variable is undefined
, null
, or a number
type.
Your code may return undefined
value if you call the .length
property to non-array or non-string data.
The following code will throw an error:
let newArr;
console.log(newArr.length); // Error: Cannot read property 'length' of undefined
A number data type will return undefined
:
let newArr = 9;
console.log(newArr.length); // undefined
But you can mitigate both problems with Array.isArray()
:
let newArr;
console.log(Array.isArray(newArr) && newArr.length); // false
Here’s the example to check whether a JavaScript variable is an array and it’s not empty:
let newArr = [2, 2, 5];
if (Array.isArray(newArr) && newArr.length) {
console.log(`The variable is an array and it has ${newArr.length} elements!`);
} else {
console.log("The variable is NOT an array or it's currently empty!");
}
Feel free to use the code snippet for your projects 😉