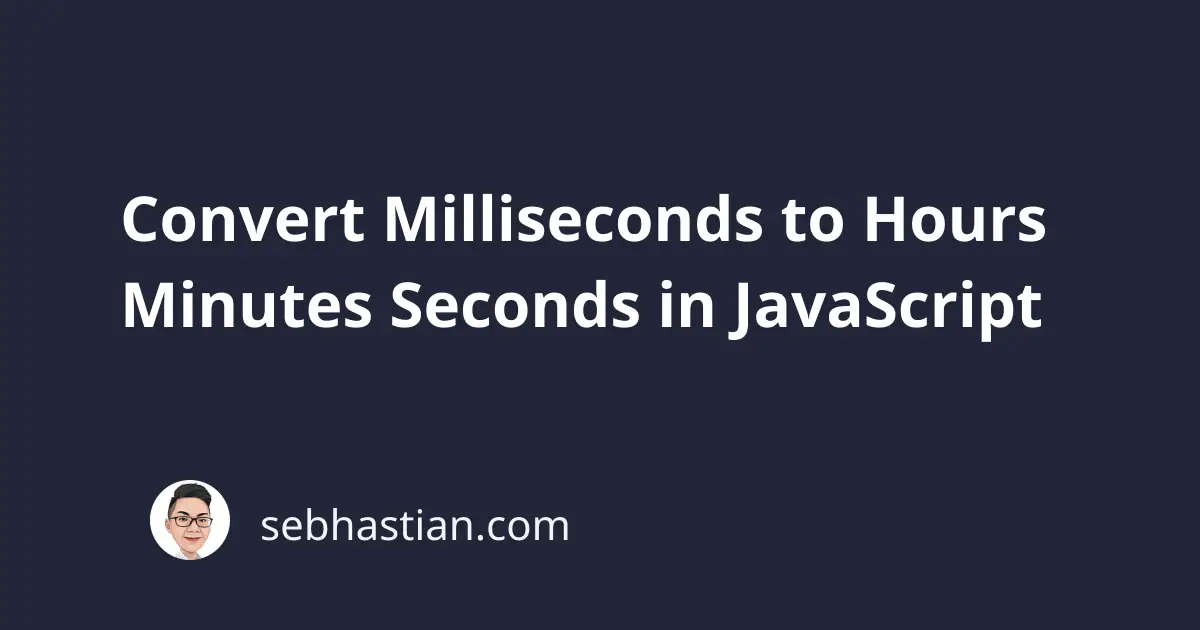
Hi everyone! In this article, I’m going to show you how to convert milliseconds into hours, minutes, and seconds in HH:MM:SS
format using JavaScript.
To format the milliseconds value into the right hours, minutes, and seconds value, you need to divide the milliseconds value, then use the modulo %
operator to get the remaining value from the result.
Convert Milliseconds to Hours
Let’s do it step by step from hours to seconds. Suppose you have a milliseconds value as shown below:
const ms = 46589302;
One hour is equal to 3,600,000 milliseconds, so you need to divide the ms
value by 3,600,000 and modulo the result by 24. Also, use the Math.floor()
method to round down the value:
const ms = 46589302;
const hours = Math.floor((ms / 3600000) % 24);
console.log(hours); // 12
Now that we got the hours part, let’s continue with the minutes part.
Convert Milliseconds to Minutes
To convert milliseconds to minutes, you need to divide the milliseconds by 60000 and module the result by 60.
Here’s how you do it:
const ms = 46589302;
const hours = Math.floor((ms / 3600000) % 24);
const minutes = Math.floor((ms / 60000) % 60);
console.log(minutes); // 56
Now we have the minutes part. Only the seconds part left.
Convert Milliseconds to Seconds
To convert milliseconds to seconds, divide the ms
value by 1000 and module the result by 60:
const ms = 46589302;
const hours = Math.floor((ms / 3600000) % 24);
const minutes = Math.floor((ms / 60000) % 60);
const seconds = Math.floor((ms / 1000) % 60);
console.log(seconds); // 29
Now we got all the values we needed from the milliseconds. Nice work!
The next step is to format the values into HH:MM:SS
format.
Formatting the time result
To format the time result, you need to call the toString()
method, followed by the padStart()
method to add zero before the numbers.
The padStart()
would be used to keep the format consistent when you have a single digit time value.
To format the code nicely, we’ll create an array from the values, and then join them together. See the example below:
const ms = 46589302;
const hours = Math.floor((ms / 3600000) % 24);
const minutes = Math.floor((ms / 60000) % 60);
const seconds = Math.floor((ms / 1000) % 60);
const timeResult = [
hours.toString().padStart(2, '0'),
minutes.toString().padStart(2, '0'),
seconds.toString().padStart(2, '0'),
].join(':');
console.log(timeResult); // 12:56:29
Now you know how to get the hours, minutes, and seconds from a milliseconds value.
You can wrap the code in a custom function called formatHMS()
so you can just call the function anytime you need to format the milliseconds:
function formatHMS(ms) {
const hours = Math.floor((ms / 3600000) % 24);
const minutes = Math.floor((ms / 60000) % 60);
const seconds = Math.floor((ms / 1000) % 60);
return [
hours.toString().padStart(2, '0'),
minutes.toString().padStart(2, '0'),
seconds.toString().padStart(2, '0'),
].join(':');
}
const myMilliseconds = 32161180
const timeFromMs = formatHMS(myMilliseconds);
console.log(timeFromMs) // 08:56:01
As you can see, now you get the time formatted correctly from the milliseconds using the formatHMS()
function.
Conclusion
This article has shown you how to get the hours, minutes, and seconds part from a value of milliseconds.
I have more JavaScript how-tos for you here:
JavaScript How to Format Date as DD/MM/YYYY
Javascript Format Date as YYYY-MM-DD
Javascript Convert Milliseconds to a Date
I hope these articles are useful. See you in other articles!