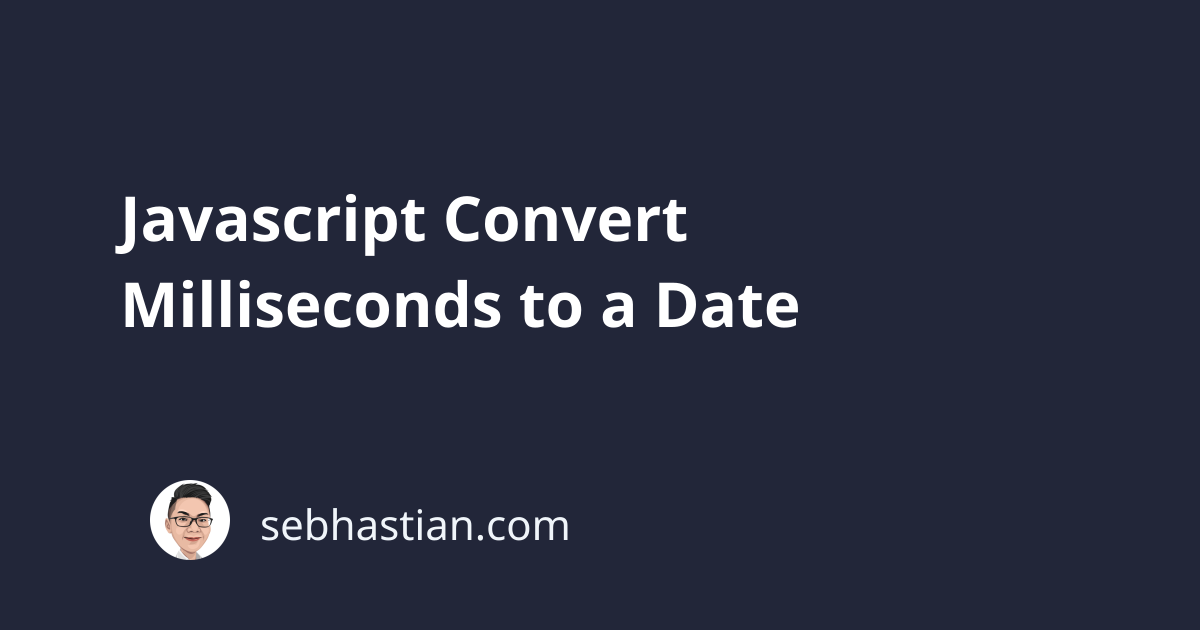
To convert milliseconds to date, you can use the Date()
constructor which creates a Date
object.
The following example shows how to convert milliseconds representing 30th August 2023 00:00:00 to a Date object:
let milliseconds = 1693353600000;
let date = new Date(milliseconds); // Wed Aug 30 2023 04:00:00 GMT+0400
Note that the Date()
constructor also takes into account the timezone set in the browser. Since my browser is GMT+4, there’s a +4 time offset in the returned Date
object.
If you want to represent the Date in the UTC zone and remove the offset, you need to use the getTimezoneOffset()
method to calculate the offset and add it to your milliseconds
value as follows:
let milliseconds = 1693353600000;
// get date with offset
let dateWithOffset = new Date(milliseconds);
// get the offset from date
let offset = dateWithOffset.getTimezoneOffset() * 60 * 1000;
// add the offset to your date
let dateWithoutOffset = new Date(milliseconds + offset);
console.log(dateWithOffset); // Wed Aug 30 2023 04:00:00 GMT+0400
console.log(dateWithoutOffset); // Wed Aug 30 2023 00:00:00 GMT+0400
Here, you can see that the dateWithoutOffset
time value is 00:00:00
.
This is because the 4 hours offset is removed from the milliseconds
when we do milliseconds + offset
, reducing the time by 4 hours
After removing the offset, the Date()
constructor we called adds the 4 hours offset back, so the time represented is 00:00:00 but in the browser timezone (GMT+4 in my case)
And that’s how you convert a timestamp into a Date in JavaScript. Here are other articles related to JavaScript date manipulation that you might find useful:
- Javascript Convert ISO String to a Date object
- How to subtract days from a JavaScript Date object
- and Javascript Convert ISO Date to a Timestamp
The JavaScript Date object is quite tricky for beginners, so I hope these tutorials can help you understand it better.
Happy coding!