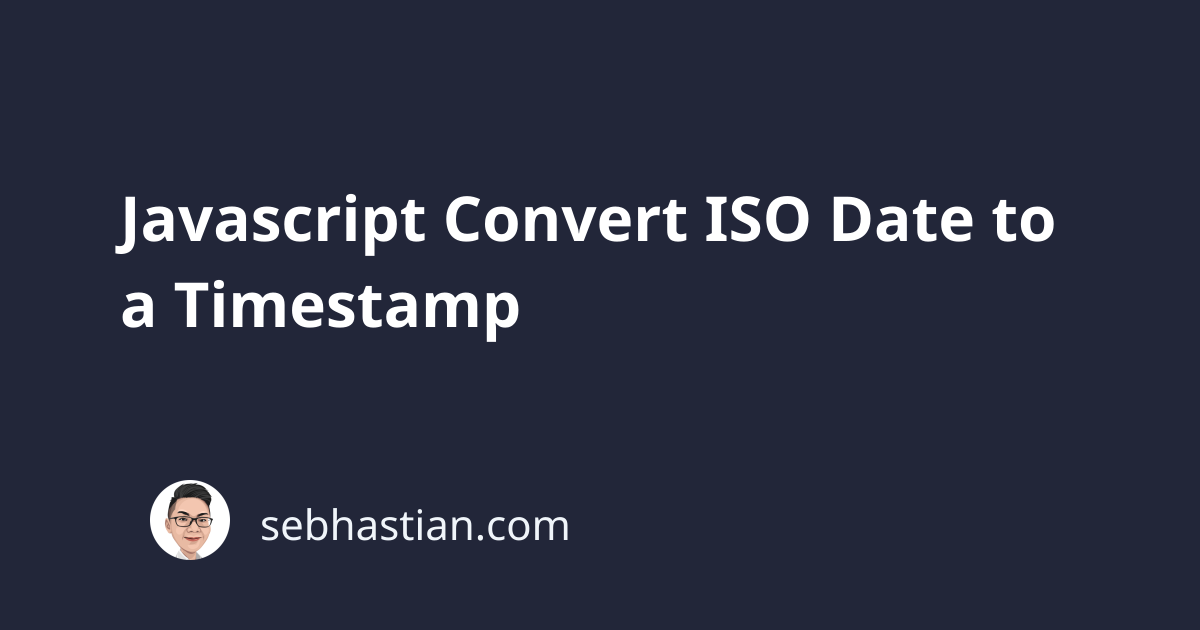
You can use the getTime()
method to convert an ISO Date object to a timestamp.
The getTime()
method returns the number of milliseconds since 00:00:00 UTC on Thursday, 1 January 1970 (the Unix Epoch)
When you have a Date
object, you can use the method to convert the date value into a timestamp as follows:
let myDate = new Date("2023-08-30T00:00:00.000Z");
console.log(myDate); // Wed Aug 30 2023 04:00:00 GMT+0400
let timestamp = myDate.getTime();
console.log(timestamp); // 1693353600000
Here, you can see that we created a new Date
object that represents today (30th August 2023) in ISO ‘YYYY-MM-DD’ format.
From the Date
object, we called the getTime()
method, which returns the number of milliseconds since Unix Epoch.
Note that the getTime()
method always uses the UTC time zone, so the browser’s time zone doesn’t change the returned time value.
This is useful because it means you can use the returned timestamp to create the same Date
object:
let myDate = new Date("2023-08-30T00:00:00.000Z");
console.log(myDate); // Wed Aug 30 2023 04:00:00 GMT+0400
let timestamp = myDate.getTime();
console.log(timestamp); // 1693353600000
let fromTimestamp = new Date(timestamp);
console.log(fromTimestamp); // Wed Aug 30 2023 04:00:00 GMT+0400
The value of myDate
and fromTimestamp
is the same because the timestamp
is in UTC zone.
And that’s how you convert an ISO Date into a timestamp.
I have other articles related to JavaScript date manipulation that you might find useful:
- Javascript Convert ISO String to a Date object
- How to subtract days from a JavaScript Date object
- and How to get tomorrow’s date in JavaScript
The JavaScript Date object is difficult to understand for beginners, so I hope these tutorials can help you out.
Happy coding!