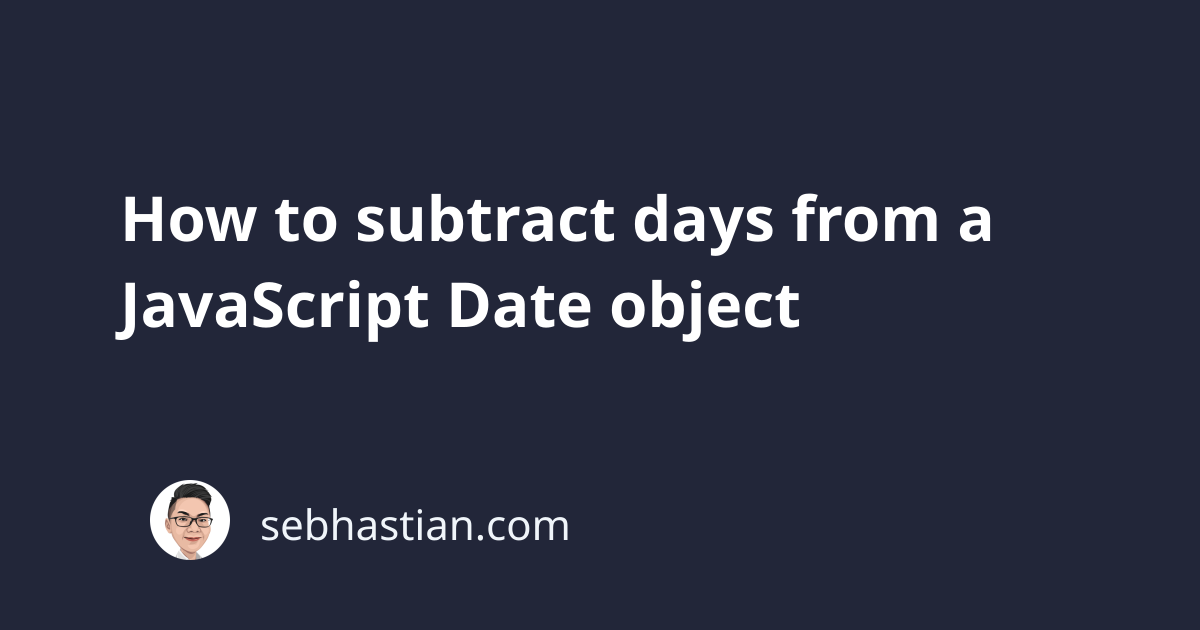
To subtract days from a JavaScript Date
object, you need to:
- Call the
getDate()
method to get the day of theDate
object - Subtract the number of days you want from the
getDate()
returned value. - Call the
setDate()
method to set the day of theDate
object
This tutorial will show you how to do the steps above.
Later, you will also learn how to get the differences between two Date
objects in JavaScript.
Subtract dates using getDate() and setDate()
The getDate()
method will return the day number of the Date
object you have:
const date = new Date("04/20/2021"); // 20th April 2021
console.log(date.getDate()); // 20
Meanwhile, the setDate()
method is used to set the day of the Date
object:
const date = new Date("04/20/2021"); // 20th April 2021
// 👇 Set the day to 5th
date.setDate(5);
console.log(date); // 5th April 2021
Combining the two methods, you can subtract days from the Date
object like this:
const date = new Date("04/20/2021"); // 20th April 2021
// 👇 Subtract 10 days from the Date object
date.setDate(date.getDate() - 10);
console.log(date); // 10th April 2021
The setDate()
method automatically adjusts the month and the year of the Date
object forward and backward.
In the code below, subtracting the days push the month back to March:
const date = new Date("04/20/2021"); // 20th April 2021
// 👇 Subtract 40 days from the Date object
date.setDate(date.getDate() - 40);
console.log(date); // 11th Mar 2021
And that’s how you subtract days from a JavaScript Date
object.
Next, let’s see how you can get the differences between two Date
objects in JavaScript.
Get the difference between two dates
To get the difference between two dates in the same month, just get the month days using getDate()
and subtract them as shown below:
const dateOne = new Date("04/20/2021"); // 20th April 2021
const dateTwo = new Date("04/10/2021"); // 10th April 2021
console.log(dateOne.getDate() - dateTwo.getDate()); // 10
When the right side operand is further date than the left side, then the result will be a negative number:
const dateOne = new Date("04/20/2021"); // 20th April 2021
const dateTwo = new Date("04/28/2021"); // 28th April 2021
console.log(dateOne.getDate() - dateTwo.getDate()); // -8
If you don’t want the result to be a negative number, than you need to convert the returned value using Math.abs()
method, which returns the absolute number value:
const dateOne = new Date("04/20/2021"); // 20th April 2021
const dateTwo = new Date("04/28/2021"); // 28th April 2021
console.log(Math.abs(dateOne.getDate() - dateTwo.getDate())); // 8
But using the getDate()
method will give the wrong result when the dates are in different months because the method only returns the day of the month.
In the following example, the difference between 20th April 2021
and 10th March 2021
is calculated as 10
even though the right result should be 41
days:
const dateOne = new Date("04/20/2021"); // 20th April 2021
const dateTwo = new Date("03/10/2021"); // 10th March 2021
console.log(dateOne.getDate() - dateTwo.getDate()); // 10
To calculate the difference between two dates that are in different months, you will need to use the getTime()
method.
Subtract dates using getTime() method
The built-in getTime()
method converts your Date
object value to the number of milliseconds since 1st January 1970
.
You can use the returned millisecond values to subtract two different dates as shown below:
const dateOne = new Date("04/20/2021"); // 20th April 2021
const dateTwo = new Date("03/10/2021"); // 10th March 2021
const difference = dateOne.getTime() - dateTwo.getTime();
console.log(difference); // 3542400000
Then, you can use the returned difference
value and convert it to months, days, minutes, or seconds depending on the measurement required by your project.
To convert the difference
from millisecond to second, just divide the difference
by 1000
:
const dateOne = new Date("04/20/2021"); // 20th April 2021
const dateTwo = new Date("03/10/2021"); // 10th March 2021
const difference = dateOne.getTime() - dateTwo.getTime();
console.log(difference / 1000); // 3542400
To convert the difference
to minutes, you need to divide it by 1000
and then divide it again by 60
because one minute equals sixty seconds:
console.log(difference / (1000 * 60)); // 59040
To find the difference
in hours, you need to divide the difference
further by 60
because one hour equals sixty minutes:
console.log(difference / (1000 * 60 * 60)); // 984
Finally, to calculate the difference
in days, divide it by 24
because one day equals twenty four hours:
console.log(difference / (1000 * 60 * 60 * 24)); // 41
And that’s how you can subtract dates in JavaScript.
Subtracting dates without Daylight Saving Time
During the Daylight Saving Time period between March and November, the time is advanced by one hour.
To calculate the date difference correctly and ignore Daylight Saving Time, you need to convert the date to UTC using the Date.UTC()
method.
To use the Date.UTC()
method, you need to pass the year, month, and day to the method.
Take a look at the following example:
const dateOne = new Date("04/20/2021"); // 20th April 2021
const dateTwo = new Date("03/10/2021"); // 10th March 2021
const dateOneUTC = Date.UTC(
dateOne.getFullYear(),
dateOne.getMonth(),
dateOne.getDate()
);
const dateTwoUTC = Date.UTC(
dateTwo.getFullYear(),
dateTwo.getMonth(),
dateTwo.getDate()
);
const difference = dateOneUTC - dateTwoUTC;
console.log(difference / (1000 * 60 * 60 * 24)); // 41
And with that, the DST will be ignored during calculation.
Now you’ve learned how to subtract days from a JavaScript Date
object, as well as how you can get the day differences between two Date
objects.
I hope this tutorial has been useful for you 🙏