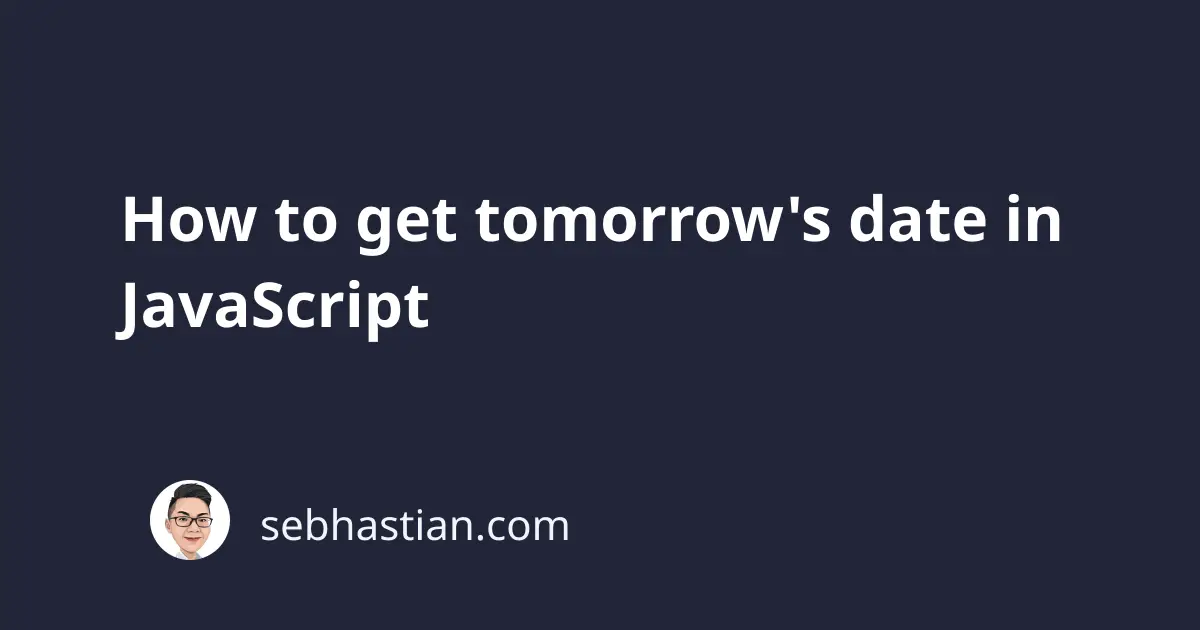
This article will help you learn how to get tomorrow’s date, how to check if a date is tomorrow, and how to format tomorrow’s date in a specific format.
How to get tomorrow’s date
To get tomorrow’s date in JavaScript, you need to perform the following steps:
- Create a new
Date
object representing today - Use
setDate()
andgetDate()
methods to properly add a day to the date object
Here’s an example code you can run:
const today = new Date();
const tomorrow = new Date();
// change tomorrow to next day
tomorrow.setDate(today.getDate() + 1);
To add one day to a Date
object, you need to call the setDate()
method, then pass getDate() + 1
into the method.
The getDate()
method returns the day of the date (between 1 and 31) while the setDate()
method updates the date of the Date
object in a proper way.
If the today
date is the end of the month, then using setDate(today.getDate() + 1)
will update the date to the beginning of the next month.
This means the code works even when you have a new month or a new year tomorrow.
Check if a date is tomorrow
If you need to check whether a specific date is tomorrow, then you can use the setDate()
and getDate()
methods to create tomorrow’s date, then compare it to the date you want to check.
In the example below, we want to check if the expiryDate
value equals tomorrow:
const expiryDate = new Date("2023-09-23");
// get tomorrow's date
const today = new Date();
const tomorrow = new Date();
tomorrow.setDate(today.getDate() + 1);
// compare expiryDate and tomorrow
const expiryIsTomorrow =
expiryDate.getDate() === tomorrow.getDate() &&
expiryDate.getMonth() === tomorrow.getMonth() &&
expiryDate.getFullYear() === tomorrow.getFullYear();
First, you need to get tomorrow’s date using the code you’ve learned in the previous section.
Then, you need to check if the getDate()
, getMonth()
and getFullYear()
from expiryDate
and tomorrow
are all equal. If they are, then expiryDate
is tomorrow. Here, the result is saved as expiryIsTomorrow
variable.
When you need to check if a date is tomorrow often, then you can create a function called isTomorrow
with the following code:
function isTomorrow(dateInput) {
if (!(dateInput instanceof Date)) {
throw new Error("");
}
// get tomorrow's date
const today = new Date();
const tomorrow = new Date();
tomorrow.setDate(today.getDate() + 1);
// compare dateInput and tomorrow
return (
dateInput.getDate() === tomorrow.getDate() &&
dateInput.getMonth() === tomorrow.getMonth() &&
dateInput.getFullYear() === tomorrow.getFullYear()
);
}
The isTomorrow()
function returns true
when dateInput
is tomorrow, otherwise it returns false
.
Get tomorrow’s date in dd/mm/yyyy format
If you want to have tomorrow’s date in a specific format, then you need to use the combination of getDate()
, getMonth()
and getFullYear()
to create a string of date format.
Here’s an example of formatting tomorrow’s date in dd/mm/yyyy
format:
const today = new Date();
const tomorrow = new Date();
// change tomorrow to next day
tomorrow.setDate(today.getDate() + 1);
console.log(today);
console.log(tomorrow);
// create a string in dd/mm/yyyy format
let day = tomorrow.getDate();
day = day < 10 ? "0" + day : day;
let month = tomorrow.getMonth() + 1;
month = month < 10 ? "0" + month : month;
const year = tomorrow.getFullYear();
const tomorrowStr = `${day}/${month}/${year}`;
Notice how you need to modify the day
and month
variables to add 0
in front of the values if those values are single digits.
Formatting a Date
object in JavaScript is annoying without an external library, so if you need to format dates extensively, I recommend you use a date library like Luxon and Day.js
Using Luxon, you can call the toFormat()
method and pass the string format like this:
const { DateTime } = require("luxon");
const dt = DateTime.local();
const dtString = dt.toFormat('dd/MM/yyyy');
Instead of having many lines of code, you can generate the date in the format you want in just one line. You also don’t need to check the date values to add leading zeroes manually.
Now you’ve learned how to get tomorrow’s date, how to check if a date is tomorrow, and how to have the date in a specific ISO format.
I hope this tutorial is helpful. See you in other tutorials! 👋