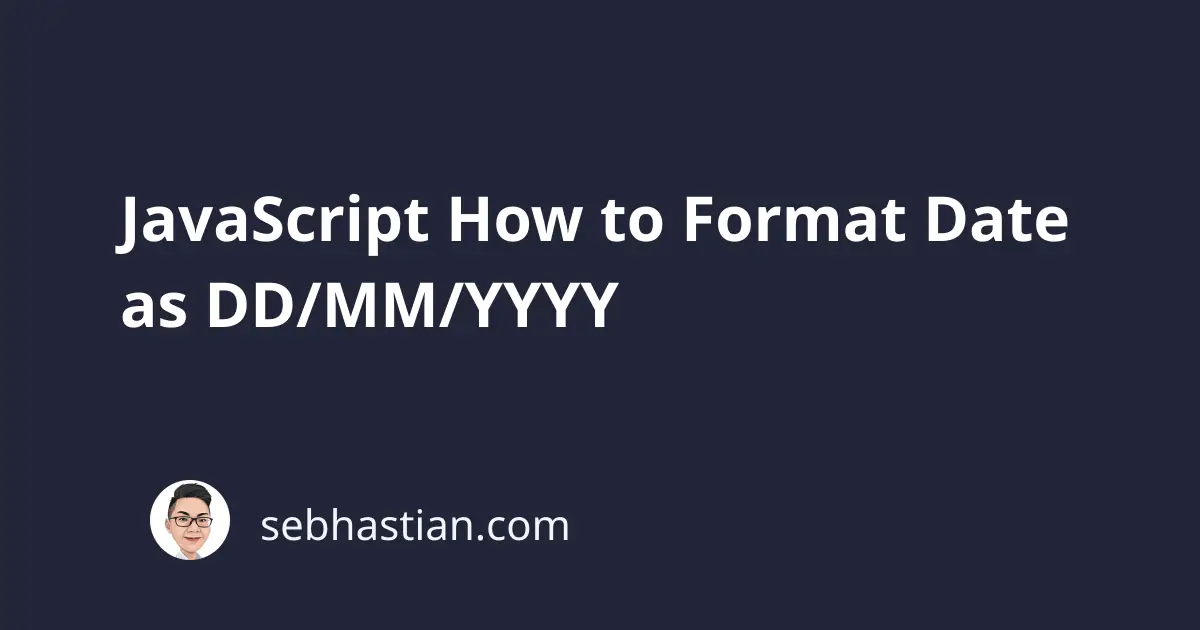
One of the most challenging tasks when programming in JavaScript is to format the Date object to fit your requirements.
Even after coding for 7+ years, I still find myself confused with the way JavaScript Date object works. In this article, I will help you learn how to format a JavaScript Date object as DD/MM/YYYY first using pure JavaScript and then with the help of a library.
How to format JavaScript Date object as DD/MM/YYYY
The JavaScript Date object format is never made clear in any part of the documentation, but by using console.log()
and printing the Date object, you can see the default representation is as follows:
let date = new Date();
console.log(date);
// Fri Sep 22 2023 13:45:45 GMT+0800 (Singapore Standard Time)
Here, you can see that JavaScript uses 3 letters for the day, 3 letters for the month, 2 digits for the date and 4 digits for the year. Then the time format follows.
The Date object has a method called toISOString()
that can format the date into a string that adheres to the ISO standard for Date and Time. Call the method on the Date object, and you’ll get the following result:
let date = new Date();
console.log(date.toISOString()); // 2023-09-22T06:45:45.185Z
The ISO format is YYYY-MM-DD, so this is not exactly what we want. To format the date in DD/MM/YYYY format, you need to use three methods available from the Date object:
- getDate()
- getMonth()
- getFullYear()
The getDate()
method will be used to get the date part, the getMonth()
method for the month part, and the getFullYear()
method for the year part.
Here’s an example of using the methods:
let date = new Date();
let day = date.getDate();
day = day < 10 ? "0" + day : day;
let month = date.getMonth() + 1;
month = month < 10 ? "0" + month : month;
const year = date.getFullYear();
const dateString = `${day}/${month}/${year}`;
console.log(dateString) // 22/09/2023
The getDate()
and getMonth()
methods return a single digit number when the result is less than 10, so we add a zero in front of these when we get a single digit result.
The getMonth()
method returns a number representing the current month, but since it starts from 0
for January, we add 1
to the result to make the representation correct.
And finally we add these variables in one string using JavaScript template strings, adding a slash /
to separate the entities. You can use a dash -
instead if you need it.
Now you know how to format a date as DD/MM/YYYY using vanilla JavaScript. Well done!
2. Format date as DD/MM/YYYY using Luxon
If you want to use a library to format a date, try out Luxon.
Luxon is a JavaScript library for date manipulation. Using Luxon, you can call the toFormat()
method and pass the string format like this:
<body>
<script src="luxon.js"></script>
<script>
var DateTime = luxon.DateTime;
const dt = DateTime.local();
const dtString = dt.toFormat('dd/MM/yyyy');
console.log(dtString); // 22/09/2023
</script>
</body>
Instead of having many lines of code, you can generate the date in the format you want in just one line. You also don’t need to manually check the date values to add leading zeroes.
You can check Luxon installation guide on how to download the library and more details.
Conclusion
Working with the JavaScript Date object is always a tricky business. To format the Date object as a DD/MM/YYYY string, you need to use the methods available in the Date object and manipulate the result to the format you want.
For easy solution, you can use the Luxon date and time library, but this might be an overkill if you only need to format a Date object. If you’re building a serious project, you probably will have lots of things to do with the Date object, so using Luxon will save time.
By the way, I also have more articles related to the JavaScript Date object here:
- Javascript Convert ISO String to a Date object
- How to subtract days from a JavaScript Date object
- and Javascript Convert Milliseconds to a Date
These tutorials should help you be confident in manipulating the Date object. Happy coding and see you later! 👍